


Avoid pitfalls: Precautions and common mistakes in mastering Golang pointers
Precautions and common mistakes of Golang pointers to help you avoid pitfalls
In Golang, a pointer is a special data type that stores a variable the address of. Through pointers, we can directly access and modify the value stored at the corresponding address. Using pointers can improve program efficiency and flexibility, but it is also prone to errors. This article will introduce the precautions for Golang pointers and help you avoid possible pitfalls through specific code examples.
- Don’t return pointers to local variables
When we want to return a pointer, we must be careful not to return pointers to local variables. Because the local variable's memory is released when the function ends, returning its pointer will result in an invalid pointer reference.
Sample code:
func createPointer() *int { i := 10 return &i // 错误!返回了局部变量的指针 } func main() { p := createPointer() fmt.Println(*p) // 会导致编译错误或者运行时错误 }
The correct approach is to use the new
keyword or use the make
function to create a pointer.
func createPointer() *int { i := 10 return new(int) // 创建一个新的指针 } func main() { p := createPointer() *p = 10 fmt.Println(*p) // 输出 10 }
- Don’t dereference nil pointers
In Golang, a nil pointer is a null pointer, that is, a pointer that points to no valid address. When we dereference a nil pointer, it causes a runtime error.
Sample code:
func main() { var p *int fmt.Println(*p) // 运行时错误 }
In order to avoid dereferencing a nil pointer, we can ensure that the pointer is valid by determining whether it is nil before using it.
func main() { var p *int if p != nil { fmt.Println(*p) } else { fmt.Println("p is nil") } }
- Pay attention to the type comparison of pointers
In Golang, the type of pointers also needs to be paid attention to. Pointers of different types cannot be compared directly.
Sample code:
func main() { var p1 *int var p2 *int if p1 == p2 { // 编译错误 fmt.Println("p1 和 p2 相等") } }
In order to compare the values of pointers, we need to convert the pointers to the same type.
func main() { var p1 *int var p2 *int if p1 == (*int)(unsafe.Pointer(p2)) { fmt.Println("p1 和 p2 相等") } }
- Avoid null references of pointers
When using pointers, be careful to avoid null references of pointers, that is, pointers do not point to valid memory addresses. If you use a null-referenced pointer for dereference or assignment, a runtime error will result.
Sample code:
func main() { var p *int *p = 10 // 运行时错误 }
In order to avoid the null reference of the pointer, we can use the new
keyword or the make
function to create the pointer and assign the corresponding memory space.
func main() { p := new(int) *p = 10 // 指针指向了有效的内存地址 fmt.Println(*p) // 输出 10 }
- Pay attention to the passing of pointers
In Golang, when calling a function, parameters are passed by value. If the argument type is a pointer, a copy of the pointer is passed, not the pointer itself. This means that modifications to the pointer within the function will not affect the original pointer.
Sample code:
func modifyPointer(p *int) { i := 10 p = &i } func main() { var p *int modifyPointer(p) fmt.Println(p) // 输出空指针 nil }
In order to modify the value of a pointer inside a function, we need to pass a pointer to the pointer or use a reference to the pointer.
func modifyPointer(p *int) { i := 10 *p = i } func main() { var p *int modifyPointer(&p) fmt.Println(*p) // 输出 10 }
Through the above precautions and sample code, I hope it can help you understand the use of Golang pointers and avoid common mistakes. When you use pointers correctly, they become a powerful tool in your programming, improving the efficiency and flexibility of your program.
The above is the detailed content of Avoid pitfalls: Precautions and common mistakes in mastering Golang pointers. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
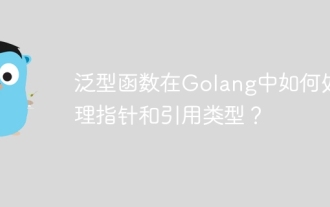
When a generic function handles pointer types in Go, it will receive a reference to the original variable, allowing the variable value to be modified. Reference types are copied when passed, making the function unable to modify the original variable value. Practical examples include using generic functions to compare strings or slices of numbers.
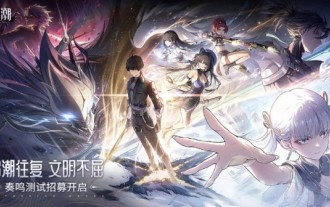
During the Mingchao test, please avoid system upgrades, factory resets, and parts replacement to prevent information loss and abnormal game login. Special reminder: There is no appeal channel during the testing period, so please handle it with caution. Introduction to matters needing attention during the Mingchao test: Do not upgrade the system, restore factory settings, replace equipment components, etc. Notes: 1. Please upgrade the system carefully during the test period to avoid information loss. 2. If the system is updated, it may cause the problem of being unable to log in to the game. 3. At this stage, the appeal channel has not yet been opened. Players are advised to choose whether to upgrade at their own discretion. 4. At the same time, one game account can only be used with one Android device and one PC. 5. It is recommended that you wait until the test is completed before upgrading the mobile phone system or restoring factory settings or replacing the device.
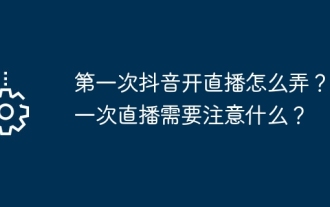
With the rise of short video platforms, Douyin has become an indispensable part of many people's daily lives. Live broadcasting on Douyin and interacting with fans are the dreams of many users. So, how do you start a live broadcast on Douyin for the first time? 1. How to start a live broadcast on Douyin for the first time? 1. Preparation To start live broadcast, you first need to ensure that your Douyin account has completed real-name authentication. You can find the real-name authentication tutorial in "Me" -> "Settings" -> "Account and Security" in the Douyin APP. After completing the real-name authentication, you can meet the live broadcast conditions and start live broadcast on the Douyin platform. 2. Apply for live broadcast permission. After meeting the live broadcast conditions, you need to apply for live broadcast permission. Open Douyin APP, click "Me"->"Creator Center"->"Direct
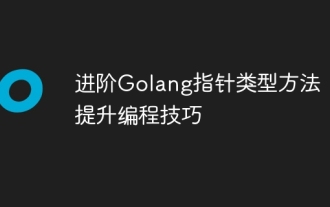
The pointer type approach is available in Go language, which allows you to define a function of pointer type in order to modify the value pointed to without explicitly passing the pointer in the method signature. This provides code simplicity and efficiency since copy-by-value passes do not need to be copied. The syntax of pointer type method is: typeTypeName*Type\nfunc(t*TypeName)MethodName(). To use pointer type methods, you create a pointer to an instance of the type and then use that pointer to call the method. The benefits of pointer type methods include code simplicity, efficiency, and modifiability. It should be noted that the pointer type method can only be used for pointer types, and you need to be careful when using it, because the structure value pointed to may be accidentally
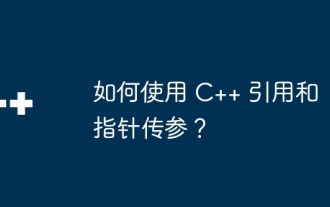
References and pointers in C++ are both methods of passing function parameters, but there are differences. A reference is an alias for a variable. Modifying the reference will modify the original variable, while the pointer stores the address of the variable. Modifying the pointer value will not modify the original variable. When choosing to use a reference or a pointer, you need to consider factors such as whether the original variable needs to be modified, whether a null value needs to be passed, and performance considerations.
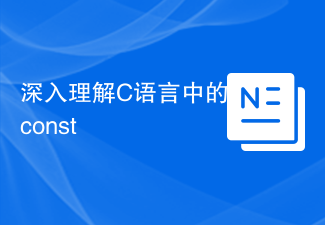
Detailed explanation and code examples of const in C In C language, the const keyword is used to define constants, which means that the value of the variable cannot be modified during program execution. The const keyword can be used to modify variables, function parameters, and function return values. This article will provide a detailed analysis of the use of the const keyword in C language and provide specific code examples. const modified variable When const is used to modify a variable, it means that the variable is a read-only variable and cannot be modified once it is assigned a value. For example: constint
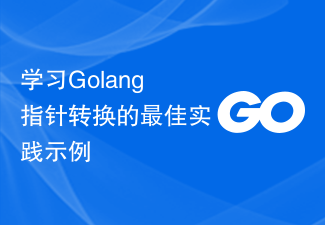
Golang is a powerful and efficient programming language that can be used to develop various applications and services. In Golang, pointers are a very important concept, which can help us operate data more flexibly and efficiently. Pointer conversion refers to the process of pointer operations between different types. This article will use specific examples to learn the best practices of pointer conversion in Golang. 1. Basic concepts In Golang, each variable has an address, and the address is the location of the variable in memory.
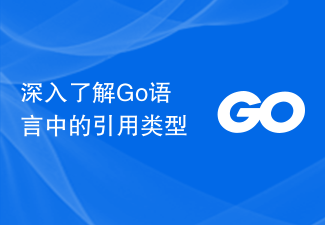
Reference types are a special data type in the Go language. Their values do not directly store the data itself, but the address of the stored data. In the Go language, reference types include slices, maps, channels, and pointers. A deep understanding of reference types is crucial to understanding the memory management and data transfer methods of the Go language. This article will combine specific code examples to introduce the characteristics and usage of reference types in Go language. 1. Slices Slices are one of the most commonly used reference types in the Go language.
