


How does Arrays.binarySearch() method in Java find specific elements in an ordered array?
The Arrays class in Java provides a series of convenient methods to deal with arrays, including the binarySearch() method for finding elements in an ordered array. This article details how to use this method and provides corresponding code examples.
In Java, the Arrays class is a tool class that provides a series of static methods for operating and processing arrays. The binarySearch() method is one of them and is used to find a specific element in an ordered array.
The signature of the binarySearch() method is as follows:
public static int binarySearch(Object[] a, Object key)
The parameters of this method include an ordered array a of type Object and an element key to be searched. It returns a value of type int representing the index value of the found element; if the element is not found, it returns a negative number indicating the position where it should be inserted.
The following is a specific example demonstrating how to use the binarySearch() method to find specific elements.
import java.util.Arrays; public class BinarySearchExample { public static void main(String[] args) { // 定义一个有序数组 int[] arr = {2, 4, 6, 8, 10, 12, 14}; // 使用binarySearch()方法查找元素8 int index = Arrays.binarySearch(arr, 8); // 输出结果 if (index >= 0) { System.out.println("元素8在数组中的索引位置为:" + index); } else { System.out.println("元素8不在数组中,应该插入的位置为:" + (-index - 1)); } } }
In the above code, we define an ordered array arr and use the binarySearch() method to find element 8. If the element is found, its index position in the array is returned; if not found, a negative number is returned indicating the position where it should be inserted.
Run the above code, the output result is:
元素8在数组中的索引位置为:3
means that the index position of element 8 in the array is 3.
If the element we are looking for is not in the array, for example, to find element 5, the running result is:
元素5不在数组中,应该插入的位置为:2
means that element 5 is not in the array and should be inserted into index position 2 to maintain the array. of orderliness.
The binarySearch() method uses a binary search algorithm at the bottom, which requires that the array must be ordered. If the array is unordered, the return result of the binarySearch() method will be unpredictable.
When using the binarySearch() method, we should pay attention to the following points:
- The array must be ordered, otherwise the result will be unpredictable.
- The element type found must be consistent with the array element type, otherwise a compilation error will occur.
- If there are multiple identical elements in the array, the binarySearch() method does not guarantee that the index of the first matching element will be returned.
To sum up, the Arrays.binarySearch() method in Java is an efficient and convenient way to find elements in an ordered array. We only need to provide an ordered array and the elements we want to find, and we can get the results quickly. At the same time, we also need to pay attention to the orderliness of the array and the consistency of the type of elements found.
The above is the detailed content of How does Arrays.binarySearch() method in Java find specific elements in an ordered array?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










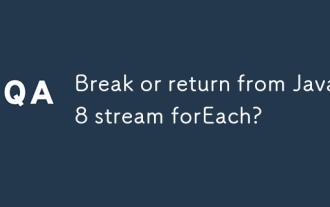
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
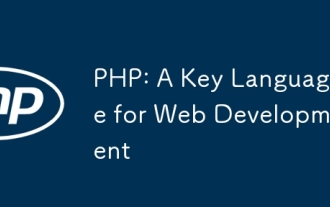
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
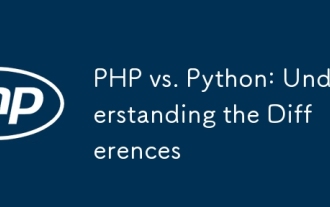
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
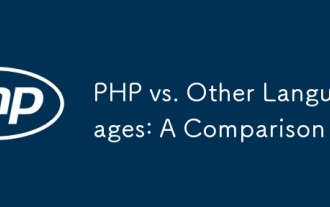
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
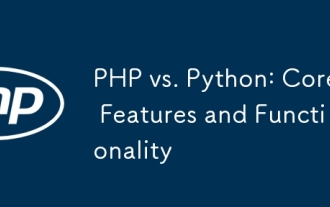
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
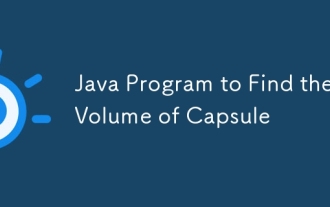
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
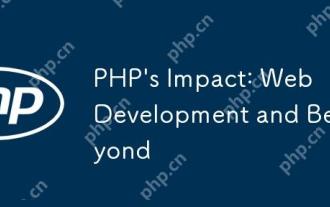
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
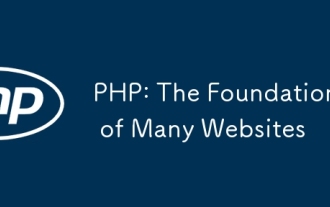
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
