Master regular expressions and string matching in JavaScript
Master regular expressions and string matching in JavaScript
Regular expression is a powerful string matching tool that can be widely used in JavaScript For string processing and data validation. Mastering regular expressions and string matching in JavaScript will help us process strings more efficiently, quickly find the required information and perform corresponding operations. This article will introduce the basic usage of regular expressions in JavaScript and provide specific code examples.
- Create regular expressions
In JavaScript, we can create regular expressions through literals or constructors. The following are two ways to create regular expressions:
1.1 Literal method
Regular expression literals are patterns surrounded by slashes (/). For example, /pattern/. The following is an example:
var pattern = /abc/;
1.2 Constructor method
Create a regular expression through the constructor method of the RegExp object. The constructor receives two parameters, the first parameter is the regular expression pattern, and the second parameter is the modifier (optional). Here is an example:
var pattern = new RegExp("abc");
- Testing for a match
Once the regular expression is created, we need to test whether it matches a the given string. JavaScript provides a few methods to test matches, including test() and exec().
2.1 test()
The test() method returns a Boolean value indicating whether content matching the regular expression is found. Here is an example:
var pattern = /abc/;
var str = "abcdefg";
console.log(pattern.test(str)); // Output: true
2.2 exec()
The exec() method returns an array containing information that matches the regular expression. If no matching content is found, null is returned. The following is an example:
var pattern = /abc/;
var str = "abcdefg";
console.log(pattern.exec(str)); // Output: ["abc "]
- String matching
In addition to testing matches, we can also perform operations such as replacement, extraction, and splitting in strings. JavaScript provides several methods to perform these operations, including replace(), match(), search(), and split().
3.1 replace()
replace() method is used to replace the part of the string that matches the regular expression with the specified string. Here is an example:
var pattern = /abc/;
var str = "abcdefg";
var newStr = str.replace(pattern, "123");
console. log(newStr); // Output: "123defg"
3.2 match()
match() method returns an array containing the parts that match the regular expression. If no matching content is found, null is returned. The following is an example:
var pattern = /abc/;
var str = "abcdefg";
console.log(str.match(pattern)); // Output: ["abc "]
3.3 search()
The search() method returns the index of the first matched position. If no matching content is found, -1 is returned. Here is an example:
var pattern = /abc/;
var str = "abcdefg";
console.log(str.search(pattern)); // Output: 0
3.4 split()
The split() method splits a string into an array based on a regular expression. Here is an example:
var pattern = /,/;
var str = "apple,banana,orange";
var arr = str.split(pattern);
console. log(arr); // Output: ["apple", "banana", "orange"]
This article introduces the basic usage of regular expressions in JavaScript, including creating regular expressions, testing matches and characters String matching related operations. By mastering these contents, we can handle strings more flexibly and improve the readability and efficiency of the code.
Summary
Regular expressions are powerful string matching tools in JavaScript. Mastering the basic usage of regular expressions is very important for string processing and data verification. Through the introduction and code examples of this article, I believe that readers have a clearer understanding of regular expressions and string matching in JavaScript, and can use them flexibly in actual development. I hope this article can be helpful to readers.
The above is the detailed content of Master regular expressions and string matching in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










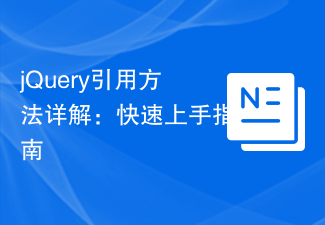
Detailed explanation of jQuery reference method: Quick start guide jQuery is a popular JavaScript library that is widely used in website development. It simplifies JavaScript programming and provides developers with rich functions and features. This article will introduce jQuery's reference method in detail and provide specific code examples to help readers get started quickly. Introducing jQuery First, we need to introduce the jQuery library into the HTML file. It can be introduced through a CDN link or downloaded
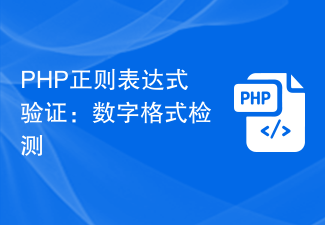
PHP regular expression verification: Number format detection When writing PHP programs, it is often necessary to verify the data entered by the user. One of the common verifications is to check whether the data conforms to the specified number format. In PHP, you can use regular expressions to achieve this kind of validation. This article will introduce how to use PHP regular expressions to verify number formats and provide specific code examples. First, let’s look at common number format validation requirements: Integers: only contain numbers 0-9, can start with a plus or minus sign, and do not contain decimal points. floating point
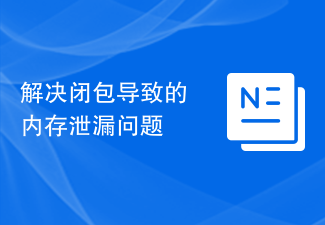
Title: Memory leaks caused by closures and solutions Introduction: Closures are a very common concept in JavaScript, which allow internal functions to access variables of external functions. However, closures can cause memory leaks if used incorrectly. This article will explore the memory leak problem caused by closures and provide solutions and specific code examples. 1. Memory leaks caused by closures The characteristic of closures is that internal functions can access variables of external functions, which means that variables referenced in closures will not be garbage collected. If used improperly,
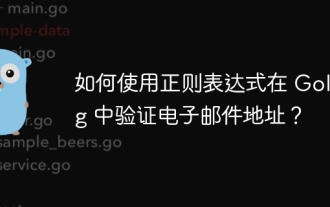
To validate email addresses in Golang using regular expressions, follow these steps: Use regexp.MustCompile to create a regular expression pattern that matches valid email address formats. Use the MatchString function to check whether a string matches a pattern. This pattern covers most valid email address formats, including: Local usernames can contain letters, numbers, and special characters: !.#$%&'*+/=?^_{|}~-`Domain names must contain at least One letter, followed by letters, numbers, or hyphens. The top-level domain (TLD) cannot be longer than 63 characters.
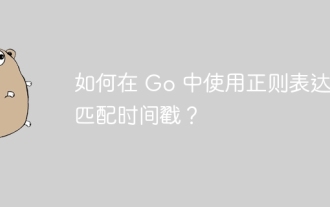
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
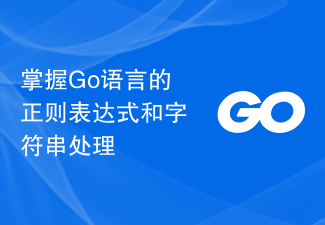
As a modern programming language, Go language provides powerful regular expressions and string processing functions, allowing developers to process string data more efficiently. It is very important for developers to master regular expressions and string processing in Go language. This article will introduce in detail the basic concepts and usage of regular expressions in Go language, and how to use Go language to process strings. 1. Regular expressions Regular expressions are a tool used to describe string patterns. They can easily implement operations such as string matching, search, and replacement.
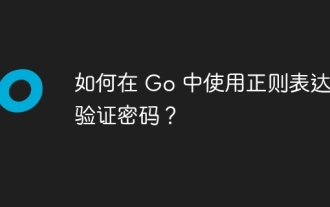
The method of using regular expressions to verify passwords in Go is as follows: Define a regular expression pattern that meets the minimum password requirements: at least 8 characters, including lowercase letters, uppercase letters, numbers, and special characters. Compile regular expression patterns using the MustCompile function from the regexp package. Use the MatchString method to test whether the input string matches a regular expression pattern.
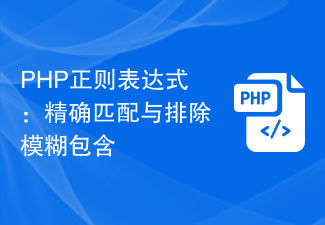
PHP Regular Expressions: Exact Matching and Exclusion Fuzzy inclusion regular expressions are a powerful text matching tool that can help programmers perform efficient search, replacement and filtering when processing text. In PHP, regular expressions are also widely used in string processing and data matching. This article will focus on how to perform exact matching and exclude fuzzy inclusion operations in PHP, and will illustrate it with specific code examples. Exact match Exact match means matching only strings that meet the exact condition, not any variations or extra words.
