


How to use Go language and Redis to develop an online question and answer platform
How to use Go language and Redis to develop an online question and answer platform
- Introduction
The online question and answer platform is a very common social platform that allows users to Post a question and get answers from other users. In this article, we will use Go language and Redis database to develop a simple online question and answer platform. Go is an efficient and reliable programming language, and Redis is a fast, scalable NoSQL database that is ideal for storing and retrieving questions and answers. - Environment preparation
Before you start, please make sure you have installed the Go language and Redis database, and have correctly configured the relevant environment variables. Project structure
We first need to create a new Go project, which can organize the code according to the following directory structure:-
project
- main.go
- question.go
- answer.go
在`main.go`文件中,我们将实现整个应用程序的入口点。在`question.go`和`answer.go`文件中,我们将定义问题和回答的相关结构和方法。
Copy after login Connect Redis
atIn the main.go
file, we first need to import thegithub.com/go-redis/redis
package and create a Redis client instance. We can achieve this through the following code:package main import ( "fmt" "github.com/go-redis/redis" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // 无密码 DB: 0, // 默认数据库 }) pong, err := client.Ping().Result() fmt.Println(pong, err) }
Copy after loginIn the above code, we created a Redis client instance named
client
and used thePing
method to test whether the connection is normal.Define the question structure
Next, in thequestion.go
file, we will define the structure of the question and related methods. The question structure can contain attributes such as title, content, creation time, etc. We can use the following code to achieve this:package main import "time" type Question struct { ID string `json:"id"` Title string `json:"title"` Content string `json:"content"` CreatedAt time.Time `json:"created_at"` } func (q *Question) Save(client *redis.Client) error { // 使用Redis的HSET命令保存问题 err := client.HSet("questions", q.ID, q).Err() if err != nil { return err } return nil } func (q *Question) GetByID(client *redis.Client, id string) error { // 使用Redis的HGET命令获取问题 val, err := client.HGet("questions", id).Result() if err != nil { return err } err = json.Unmarshal([]byte(val), q) if err != nil { return err } return nil } func (q *Question) GetAll(client *redis.Client) ([]Question, error) { // 使用Redis的HGETALL命令获取所有问题 vals, err := client.HGetAll("questions").Result() if err != nil { return nil, err } questions := make([]Question, len(vals)) i := 0 for _, val := range vals { err = json.Unmarshal([]byte(val), &questions[i]) if err != nil { return nil, err } i++ } return questions, nil }
Copy after loginIn the above code, we define a
Question
structure and implement methods to save questions, obtain questions based on ID, and obtain all questions.Define the answer structure
Similarly, in theanswer.go
file, we will define the answer structure and related methods. The answer structure can contain attributes such as question ID, answer content, creation time, etc. We can use the following code to achieve this:package main import "time" type Answer struct { ID string `json:"id"` QuestionID string `json:"question_id"` Content string `json:"content"` CreatedAt time.Time `json:"created_at"` } func (a *Answer) Save(client *redis.Client) error { // 使用Redis的HSET命令保存回答 err := client.HSet("answers", a.ID, a).Err() if err != nil { return err } return nil } func (a *Answer) GetByQuestionID(client *redis.Client, questionID string) ([]Answer, error) { // 使用Redis的HGETALL命令获取指定问题的所有回答 vals, err := client.HGetAll("answers").Result() if err != nil { return nil, err } answers := make([]Answer, 0) for _, val := range vals { answer := Answer{} err = json.Unmarshal([]byte(val), &answer) // 遍历所有回答,找到与指定问题ID相匹配的回答 if answer.QuestionID == questionID { answers = append(answers, answer) } } return answers, nil }
Copy after loginIn the above code, we define a
Answer
structure and implement the method of saving the answer and obtaining the answer based on the question ID.Using the Q&A platform
In themain
function of themain.go
file, we can test and demonstrate how to use online Q&A platform. We can implement it according to the following code:package main import ( "fmt" "github.com/go-redis/redis" ) func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // 无密码 DB: 0, // 默认数据库 }) // 创建问题 question := Question{ ID: "1", Title: "如何学习Go语言?", Content: "我想学习Go语言,请问有什么好的学习资源推荐吗?", CreatedAt: time.Now(), } err := question.Save(client) if err != nil { fmt.Println("保存问题失败:", err) return } // 根据ID获取问题 err = question.GetByID(client, "1") if err != nil { fmt.Println("根据ID获取问题失败:", err) return } fmt.Println("问题标题:", question.Title) // 获取所有问题 questions, err := question.GetAll(client) if err != nil { fmt.Println("获取所有问题失败:", err) return } for _, q := range questions { fmt.Println("问题标题:", q.Title) } // 创建回答 answer := Answer{ ID: "1", QuestionID: "1", Content: "推荐去官方网站学习Go语言。", CreatedAt: time.Now(), } err = answer.Save(client) if err != nil { fmt.Println("保存回答失败:", err) return } // 根据问题ID获取回答 answers, err := answer.GetByQuestionID(client, "1") if err != nil { fmt.Println("根据问题ID获取回答失败:", err) return } for _, a := range answers { fmt.Println("回答内容:", a.Content) } }
Copy after loginIn the above code, we demonstrate how to use the online Q&A platform by creating questions, getting questions based on IDs, getting all questions, creating answers, getting answers based on question IDs, etc.
So far, we have developed a simple online question and answer platform using Go language and Redis. Through this platform, users can post questions and get answers from other users. By studying the sample code provided in this article, you should be able to further extend and improve this platform to make it more suitable for practical application scenarios. Hope this article is helpful to you!
The above is the detailed content of How to use Go language and Redis to develop an online question and answer platform. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










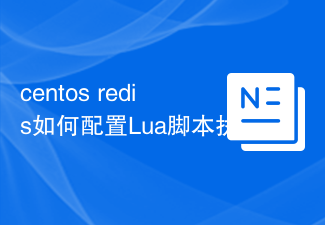
On CentOS systems, you can limit the execution time of Lua scripts by modifying Redis configuration files or using Redis commands to prevent malicious scripts from consuming too much resources. Method 1: Modify the Redis configuration file and locate the Redis configuration file: The Redis configuration file is usually located in /etc/redis/redis.conf. Edit configuration file: Open the configuration file using a text editor (such as vi or nano): sudovi/etc/redis/redis.conf Set the Lua script execution time limit: Add or modify the following lines in the configuration file to set the maximum execution time of the Lua script (unit: milliseconds)
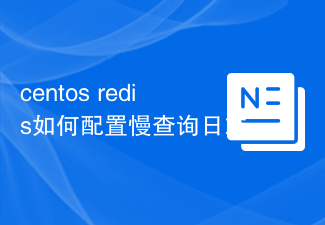
Enable Redis slow query logs on CentOS system to improve performance diagnostic efficiency. The following steps will guide you through the configuration: Step 1: Locate and edit the Redis configuration file First, find the Redis configuration file, usually located in /etc/redis/redis.conf. Open the configuration file with the following command: sudovi/etc/redis/redis.conf Step 2: Adjust the slow query log parameters in the configuration file, find and modify the following parameters: #slow query threshold (ms)slowlog-log-slower-than10000#Maximum number of entries for slow query log slowlog-max-len
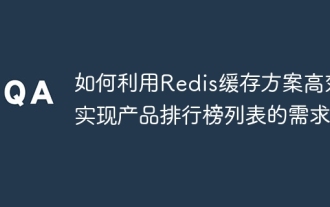
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
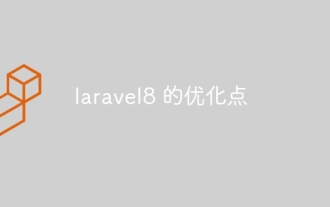
Laravel 8 provides the following options for performance optimization: Cache configuration: Use Redis to cache drivers, cache facades, cache views, and page snippets. Database optimization: establish indexing, use query scope, and use Eloquent relationships. JavaScript and CSS optimization: Use version control, merge and shrink assets, use CDN. Code optimization: Use Composer installation package, use Laravel helper functions, and follow PSR standards. Monitoring and analysis: Use Laravel Scout, use Telescope, monitor application metrics.
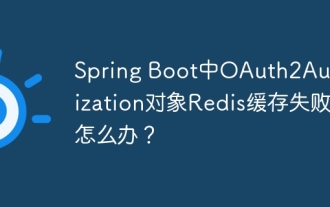
In SpringBoot, use Redis to cache OAuth2Authorization object. In SpringBoot application, use SpringSecurityOAuth2AuthorizationServer...
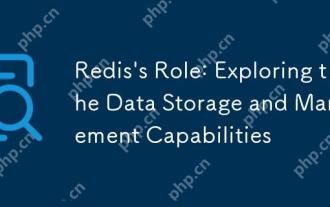
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.

When installing and configuring GitLab on a CentOS system, the choice of database is crucial. GitLab is compatible with multiple databases, but PostgreSQL and MySQL (or MariaDB) are most commonly used. This article analyzes database selection factors and provides detailed installation and configuration steps. Database Selection Guide When choosing a database, you need to consider the following factors: PostgreSQL: GitLab's default database is powerful, has high scalability, supports complex queries and transaction processing, and is suitable for large application scenarios. MySQL/MariaDB: a popular relational database widely used in Web applications, with stable and reliable performance. MongoDB:NoSQL database, specializes in
