


How to handle the load balancing problem of concurrent database connections in Go language?
How to handle the load balancing problem of concurrent database connections in Go language?
In modern web applications, the database is one of the essential components. As the traffic of web applications increases, how to handle the load balancing problem of concurrent database connections has become a key challenge. As a high-performance concurrent programming language, Go language provides many excellent solutions to deal with this problem. This article will introduce how to handle the load balancing issue of concurrent database connections in Go language, and provide specific code examples.
1. Load balancing strategy
Load balancing refers to the process of evenly distributing concurrent connections among multiple servers (or database instances). In handling load balancing issues of concurrent database connections, we need to consider the following aspects:
- Connection pool management: In order to improve performance, we can maintain a database connection pool, obtain and Reuse connections to reduce connection creation and destruction overhead.
- Connection routing: Route concurrent connection requests to different database instances to achieve load balancing. The target database instance can be selected based on certain strategies, such as polling, random, etc.
- Connection timeout and retry mechanism: When there are too many connection requests, connection timeout may occur. In this case, it is necessary to implement a connection timeout and retry mechanism to ensure the stability and reliability of the system.
2. Code Example
The following is a sample code that uses Go language to handle the load balancing problem of concurrent database connections. We use the database/sql package of the Go language to implement database connection pool management, and use the built-in goroutine and channel of the Go language to implement the scheduling and control of concurrent database connections.
package main import ( "database/sql" "fmt" "log" "sync" _ "github.com/go-sql-driver/mysql" ) var ( databasePool []*sql.DB loadBalancer int lock sync.Mutex ) func init() { databasePool = make([]*sql.DB, 0) loadBalancer = 0 } // 初始化数据库连接池 func initDatabasePool(dsn string, maxConn int) { for i := 0; i < maxConn; i++ { db, err := sql.Open("mysql", dsn) if err != nil { log.Fatalf("Failed to open database connection: %v", err) } err = db.Ping() if err != nil { log.Fatalf("Failed to ping database: %v", err) } databasePool = append(databasePool, db) } } // 获取数据库连接 func getDatabaseConn() *sql.DB { lock.Lock() defer lock.Unlock() loadBalancer = (loadBalancer + 1) % len(databasePool) return databasePool[loadBalancer] } // 用户查询函数 func queryUser(name string) { db := getDatabaseConn() rows, err := db.Query("SELECT * FROM user WHERE name = ?", name) if err != nil { log.Printf("Failed to query user: %v", err) return } defer rows.Close() for rows.Next() { // 处理查询结果 } if err = rows.Err(); err != nil { log.Printf("Failed to iterating over query results: %v", err) return } } func main() { initDatabasePool("username:password@tcp(localhost:3306)/mydb", 5) for i := 0; i < 10; i++ { go queryUser("test") } select {} }
In the above code, we first use the init function to initialize the database connection pool and specify the DSN and maximum number of connections for the database connection. Then in the queryUser function, use the getDatabaseConn function to obtain the database connection, and use the connection to perform query operations. Finally, start 10 goroutines in the main function to concurrently execute the queryUser function.
In this way, we can achieve load balancing of concurrent database connections and ensure system performance and stability.
Summary:
Through the above code examples, we show how to use the Go language to handle the load balancing problem of concurrent database connections. Through reasonable load balancing strategies, such as connection pool management, connection routing, connection timeout and other mechanisms, we can effectively improve the performance and reliability of the system. I hope this article can help you understand the load balancing problem of concurrent database connections in Go language.
The above is the detailed content of How to handle the load balancing problem of concurrent database connections in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
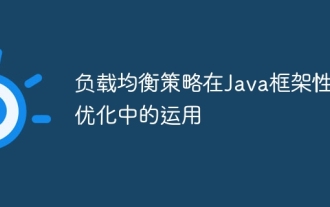
Load balancing strategies are crucial in Java frameworks for efficient distribution of requests. Depending on the concurrency situation, different strategies have different performance: Polling method: stable performance under low concurrency. Weighted polling method: The performance is similar to the polling method under low concurrency. Least number of connections method: best performance under high concurrency. Random method: simple but poor performance. Consistent Hashing: Balancing server load. Combined with practical cases, this article explains how to choose appropriate strategies based on performance data to significantly improve application performance.
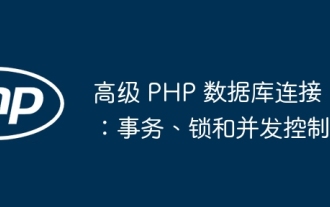
Advanced PHP database connections involve transactions, locks, and concurrency control to ensure data integrity and avoid errors. A transaction is an atomic unit of a set of operations, managed through the beginTransaction(), commit(), and rollback() methods. Locks prevent simultaneous access to data via PDO::LOCK_SHARED and PDO::LOCK_EXCLUSIVE. Concurrency control coordinates access to multiple transactions through MySQL isolation levels (read uncommitted, read committed, repeatable read, serialized). In practical applications, transactions, locks and concurrency control are used for product inventory management on shopping websites to ensure data integrity and avoid inventory problems.
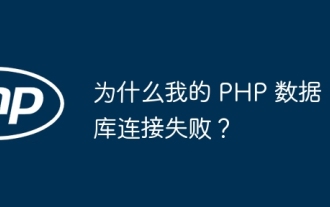
Reasons for a PHP database connection failure include: the database server is not running, incorrect hostname or port, incorrect database credentials, or lack of appropriate permissions. Solutions include: starting the server, checking the hostname and port, verifying credentials, modifying permissions, and adjusting firewall settings.
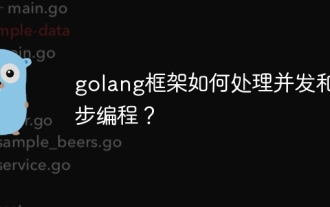
The Go framework uses Go's concurrency and asynchronous features to provide a mechanism for efficiently handling concurrent and asynchronous tasks: 1. Concurrency is achieved through Goroutine, allowing multiple tasks to be executed at the same time; 2. Asynchronous programming is implemented through channels, which can be executed without blocking the main thread. Task; 3. Suitable for practical scenarios, such as concurrent processing of HTTP requests, asynchronous acquisition of database data, etc.
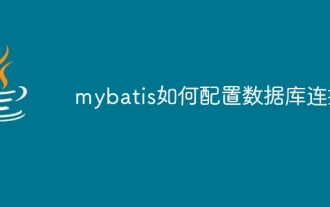
How to configure database connection in mybatis: 1. Specify the data source; 2. Configure the transaction manager; 3. Configure the type processor and mapper; 4. Use environment elements; 5. Configure aliases. Detailed introduction: 1. Specify the data source. In the "mybatis-config.xml" file, you need to configure the data source. The data source is an interface, which provides a database connection; 2. Configure the transaction manager to ensure the normality of database transactions. For processing, you also need to configure the transaction manager; 3. Configure the type processor and mapper, etc.
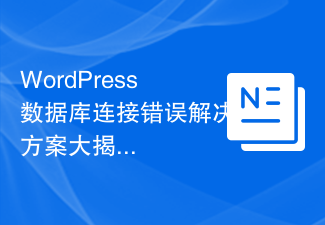
WordPress is currently one of the most popular website building platforms in the world, but during use, you sometimes encounter database connection errors. This kind of error will cause the website to be unable to be accessed normally, causing trouble to the website administrator. This article will reveal how to solve WordPress database connection errors and provide specific code examples to help readers solve this problem more quickly. Problem Analysis WordPress database connection errors are usually caused by the following reasons: Incorrect database username or password data
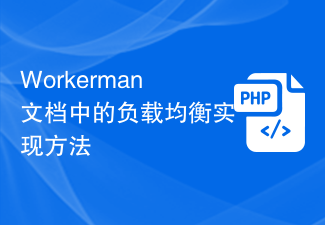
Workerman is a high-performance network framework developed based on PHP and is widely used to build real-time communication systems and high-concurrency services. In actual application scenarios, we often need to improve system reliability and performance through load balancing. This article will introduce how to implement load balancing in Workerman and provide specific code examples. Load balancing refers to allocating network traffic to multiple back-end servers to improve the system's load capacity, reduce response time, and increase system availability and scalability. In Wo
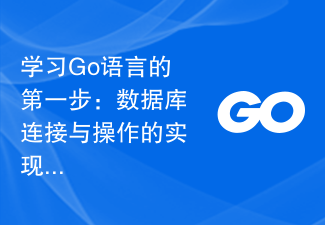
Learn Go language from scratch: How to implement database connection and operation, specific code examples are required 1. Introduction Go language is an open source programming language, developed by Google, and widely used to build high-performance, reliable server-side software . In Go language, using a database is a very common requirement. This article will introduce how to implement database connection and operation in Go language, and give specific code examples. 2. Choose the appropriate database driver. In the Go language, there are many third-party database drivers to choose from, such as My
