


How to deal with file system file retrieval and full-text retrieval of concurrent files in Go language?
Go language is a popular high-performance programming language. File retrieval and full-text retrieval in the file system through concurrent processing is one of the important tasks. In this article, we will discuss how to solve this problem using Go language and provide concrete code examples.
In the Go language, you can use the os and io packages provided in the standard library to handle file retrieval and full-text retrieval of the file system. First, we need to open the file and read the file contents. When processing large files, in order to efficiently read the file contents concurrently, we can use multiple goroutines to read the files in parallel. The following is a sample code:
package main import ( "fmt" "io/ioutil" "os" "path/filepath" "sync" ) func main() { rootDir := "/path/to/files" // 设置要检索的根目录 files, err := getFiles(rootDir) if err != nil { fmt.Println("获取文件列表失败:", err) return } // 设置并发读取文件的goroutine数量 concurrency := 10 fileChan := make(chan string, concurrency) wg := sync.WaitGroup{} wg.Add(concurrency) // 启动多个goroutine并行读取文件内容 for i := 0; i < concurrency; i++ { go func() { for file := range fileChan { content, err := readFileContent(file) if err != nil { fmt.Printf("读取文件 %s 失败: %v ", file, err) } else { // TODO: 处理文件内容 } } wg.Done() }() } // 将文件加入到文件通道 for _, file := range files { fileChan <- file } close(fileChan) wg.Wait() } func getFiles(rootDir string) ([]string, error) { var files []string err := filepath.Walk(rootDir, func(path string, info os.FileInfo, err error) error { if err != nil { return err } if !info.IsDir() { files = append(files, path) } return nil }) if err != nil { return nil, err } return files, nil } func readFileContent(file string) ([]byte, error) { content, err := ioutil.ReadFile(file) if err != nil { return nil, err } return content, nil }
In the above sample code, we first use the getFiles
function to get all file paths in the root directory. Then, we create a file channel fileChan
and a sync.WaitGroup
that uses semicolons to limit the number of concurrencies. Next, we started multiple goroutines to read the file contents in parallel. Finally, we add the file path to the file channel, close the channel, and call the Wait
method of sync.WaitGroup
to wait for all read operations to complete.
In the sample code, we simply read the file content and did not perform specific file retrieval or full-text retrieval. In practical applications, we can use string matching, regular expressions or other algorithms to implement search and filtering operations on file contents based on requirements.
By using concurrent processing, we can take full advantage of multi-core CPUs and improve the efficiency of file retrieval and full-text retrieval. At the same time, the rich concurrency primitives and functions in the standard library provided by the Go language can reduce the complexity of concurrent programming, making it simpler and more efficient to deal with file retrieval and full-text retrieval issues in the file system.
I hope this article can help readers understand how to use Go language to handle concurrent file retrieval and full-text retrieval issues in the file system, and the code examples provided can inspire readers to apply concurrent processing technology in actual development.
The above is the detailed content of How to deal with file system file retrieval and full-text retrieval of concurrent files in Go language?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
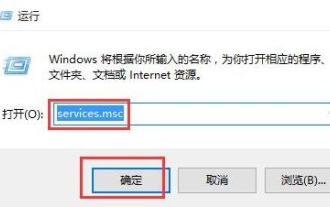
1. Press win+r to enter the run window, enter [services.msc] and press Enter. 2. In the service window, find [windows license manager service] and double-click to open it. 3. In the interface, change the startup type to [Automatic], and then click [Apply → OK]. 4. Complete the above settings and restart the computer.
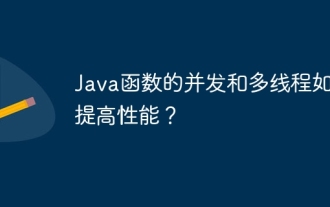
Concurrency and multithreading techniques using Java functions can improve application performance, including the following steps: Understand concurrency and multithreading concepts. Leverage Java's concurrency and multi-threading libraries such as ExecutorService and Callable. Practice cases such as multi-threaded matrix multiplication to greatly shorten execution time. Enjoy the advantages of increased application response speed and optimized processing efficiency brought by concurrency and multi-threading.
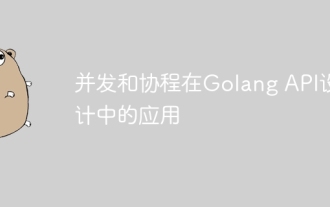
Concurrency and coroutines are used in GoAPI design for: High-performance processing: Processing multiple requests simultaneously to improve performance. Asynchronous processing: Use coroutines to process tasks (such as sending emails) asynchronously, releasing the main thread. Stream processing: Use coroutines to efficiently process data streams (such as database reads).
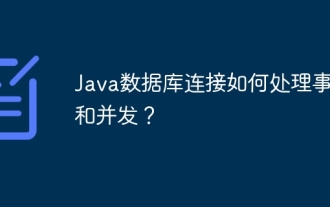
Transactions ensure database data integrity, including atomicity, consistency, isolation, and durability. JDBC uses the Connection interface to provide transaction control (setAutoCommit, commit, rollback). Concurrency control mechanisms coordinate concurrent operations, using locks or optimistic/pessimistic concurrency control to achieve transaction isolation to prevent data inconsistencies.
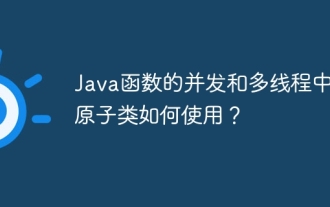
Atomic classes are thread-safe classes in Java that provide uninterruptible operations and are crucial for ensuring data integrity in concurrent environments. Java provides the following atomic classes: AtomicIntegerAtomicLongAtomicReferenceAtomicBoolean These classes provide methods for getting, setting, and comparing values to ensure that the operation is atomic and will not be interrupted by threads. Atomic classes are useful when working with shared data and preventing data corruption, such as maintaining concurrent access to a shared counter.
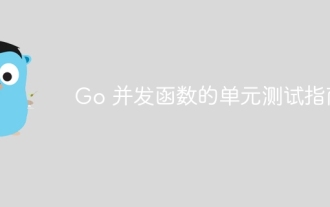
Unit testing concurrent functions is critical as this helps ensure their correct behavior in a concurrent environment. Fundamental principles such as mutual exclusion, synchronization, and isolation must be considered when testing concurrent functions. Concurrent functions can be unit tested by simulating, testing race conditions, and verifying results.
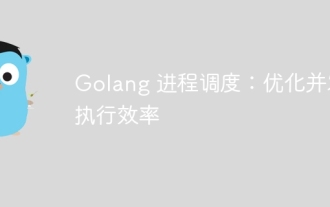
Go process scheduling uses a cooperative algorithm. Optimization methods include: using lightweight coroutines as much as possible to reasonably allocate coroutines to avoid blocking operations and use locks and synchronization primitives.
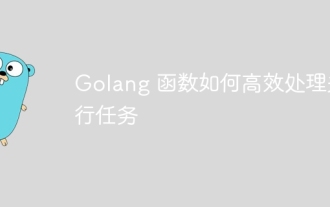
Efficient parallel task handling in Go functions: Use the go keyword to launch concurrent routines. Use sync.WaitGroup to count the number of outstanding routines. When the routine completes, wg.Done() is called to decrement the counter. The main program blocks using wg.Wait() until all routines are completed. Practical case: Send web requests concurrently and collect responses.
