How to use PHP and Vue to implement data formatting function
How to use PHP and Vue to implement data formatting function
Overview
In Web development, data formatting is a common requirement. For example, format dates into specific strings, display numbers according to specific rules, etc. This article will introduce how to use PHP and Vue to implement data formatting functions, and provide specific code examples.
1. PHP data formatting
PHP is a server-side scripting language that can be used to process and format data. Here are some common data formatting functions:
- number_format function: used to format a number into a string with thousands separators. This function accepts three parameters: the number to format, the number of digits to retain after the decimal point, and the character for the thousands separator.
- date function: used to format the timestamp into a specified date string. This function accepts two parameters: timestamp and date format.
- strtotime function: used to convert date string to timestamp. This function accepts one parameter: a date string.
The specific code examples are as follows:
// 格式化数字 $number = 1234567.89; $formattedNumber = number_format($number, 2, '.', ','); // 输出结果:1,234,567.89 // 格式化日期 $timestamp = time(); $formattedDate = date('Y-m-d H:i:s', $timestamp); // 输出结果:2022-01-01 12:34:56 // 将日期字符串转换为时间戳 $dateString = '2022-01-01'; $timestamp = strtotime($dateString); // 输出结果:1640995200
2. Vue data formatting
Vue is a JavaScript framework used to build user interfaces and can be used to implement data on the front end format. The following are some common data formatting methods:
- computed attribute: Use the computed attribute to format the data and bind the formatted data to the page. For example, you can format dates into a specified format, format numbers into strings with units, etc.
- Filter: A filter is a method used when formatting text output. Filters can be defined in Vue instances and used in templates. Filters can accept parameters and process the data.
The specific code examples are as follows:
// computed属性 computed: { formattedDate() { return moment(this.timestamp).format('YYYY-MM-DD HH:mm:ss'); }, formattedNumber() { return this.number.toFixed(2).replace(/d(?=(d{3})+.)/g, '$&,'); } } // 过滤器 filters: { formatDate(timestamp) { return moment(timestamp).format('YYYY-MM-DD HH:mm:ss'); }, formatNumber(number) { return number.toFixed(2).replace(/d(?=(d{3})+.)/g, '$&,'); } }
In the above code, the moment.js library is used to format the date. You need to introduce the moment.js library before you can use it.
3. Combined use of PHP and Vue data formatting
In actual development, it is often necessary to use PHP on the back end to format the data, and then use Vue on the front end to display the formatted data. . At this time, the formatted data can be passed to the front end through the API and used in Vue. Specific code examples are as follows:
PHP code:
// 根据API获取到的数据进行格式化 $number = 1234567.89; $formattedNumber = number_format($number, 2, '.', ','); // 返回格式化后的数据 $data = [ 'formattedNumber' => $formattedNumber ]; header('Content-Type: application/json'); echo json_encode($data);
Vue code:
// 在Vue中使用axios获取后端API返回的数据 axios.get('/api/data').then(response => { this.formattedNumber = response.data.formattedNumber; });
In the above code, the backend returns formatted data through the API, and the frontend uses axios The library sends the request and binds the returned data to the formattedNumber property in the Vue instance.
Conclusion
This article introduces how to use PHP and Vue to implement data formatting functions. Data can be formatted easily by using PHP's data formatting functions and Vue's computed properties or filters. In actual development, you can choose the appropriate method to implement data formatting according to specific needs.
The above is the detailed content of How to use PHP and Vue to implement data formatting function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
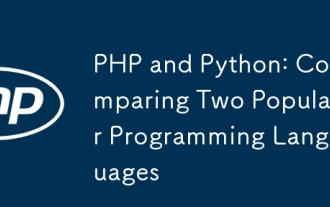
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
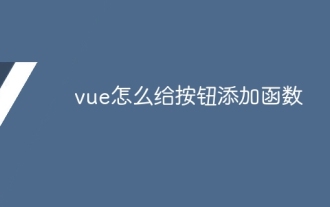
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
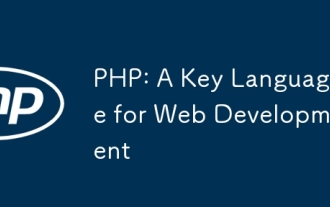
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7

The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
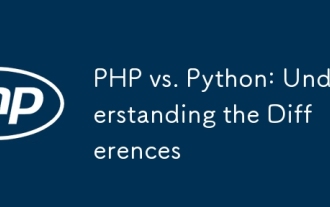
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
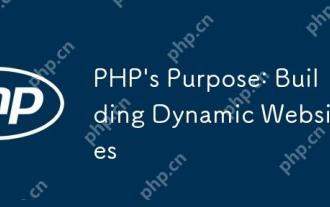
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.
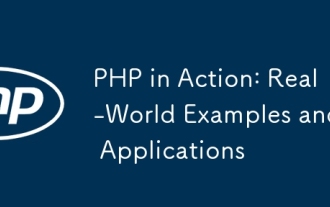
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
