


How to add multi-language support to your accounting system - How to develop multi-language accounting programs using PHP
How to add multi-language support to the accounting system - How to use PHP to develop multi-language accounting programs, requiring specific code examples
With the development of globalization, Multinational enterprises and international exchanges are becoming more and more frequent. In order to facilitate users from different countries and regions, multi-language support has become an essential feature of many software and websites. In this article, we will introduce how to add multi-language support to the accounting system, as well as specific methods and code examples for developing multi-language accounting programs using PHP.
1. Preparation
Before starting, we need to prepare the following working environment and tools:
- The PHP environment has been installed on the computer;
- The source code of the accounting system;
- Language pack file, which contains the translation content of each language.
2. Create a language pack file
First, we need to create a language pack file to store the translation content of each language. A language pack file is a text file that uses a specific format to describe translation content in different languages. The following is an example of a language pack file:
<?php return [ 'welcome' => '欢迎', 'hello' => '你好', 'goodbye' => '再见', // 其他翻译内容... ];
In this example, we define three translation contents: 'welcome', 'hello', and 'goodbye'. These contents correspond to translations in different languages.
3. Add multi-language support to the system
Next, we need to modify the source code of the accounting system and add multi-language support to it. First, add a configuration item to the system configuration file to specify the currently used language. The following is a sample configuration file:
<?php return [ // 其他配置项... 'language' => 'cn', // 当前使用的语言 ];
In this example, we set the current language to 'cn', which means Chinese.
Then, in the core code of the system, we need to load the corresponding language pack file according to the currently used language, and replace the original text according to the translation content. The following is a sample code:
<?php // 加载语言包文件 $language = require 'language/cn.php'; // 将原始文本替换为翻译内容 echo $language['welcome']; echo $language['hello']; echo $language['goodbye']; // 其他代码...
In this example, we first load the language pack file using the require function and assign it to the variable $language. Then, via the $language array, we can replace the original text based on the translation.
4. Switch the translation content according to the language selected by the user
In order to allow users to switch languages freely, we can add a language selection function to the accounting system. The following is a sample code:
<?php // 获取用户选择的语言 $selectedLanguage = $_GET['language']; // 根据用户选择的语言加载对应的语言包文件 switch ($selectedLanguage) { case 'cn': $language = require 'language/cn.php'; break; case 'en': $language = require 'language/en.php'; break; // 其他语言... } // 将原始文本替换为翻译内容 echo $language['welcome']; echo $language['hello']; echo $language['goodbye']; // 其他代码...
In this example, we obtain the language selected by the user through the $_GET variable, and load the corresponding language pack file based on the language selected by the user.
5. Summary
Through the above methods and code examples, we can add multi-language support to the accounting system. Users can choose different languages according to their needs, making it easier to use the accounting system. At the same time, developers can also add more language pack files as needed to support more languages and regions.
In short, by using PHP to develop multi-language accounting programs, we can meet the needs of users in different languages and improve the ease of use and applicability of the system. Hope this article helps you!
The above is the detailed content of How to add multi-language support to your accounting system - How to develop multi-language accounting programs using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
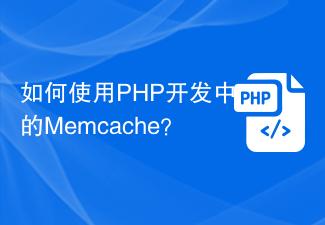
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
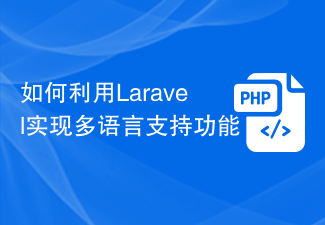
Laravel is a very popular PHP framework that provides a large number of features and libraries that make web application development easier and more efficient. One of the important features is multi-language support. Laravel achieves multi-language support through its own language package mechanism and third-party libraries. This article will introduce how to use Laravel to implement multi-language support and provide specific code examples. Using Laravel's language pack function Laravel comes with a language pack mechanism that allows us to easily implement multilingualism

Laravel is a widely used PHP framework that provides many convenient features and tools, including middleware that supports multiple languages. In this article, we will detail how to use middleware to implement Laravel's multi-language support and provide some specific code examples. Configuring the language pack First, we need to configure Laravel's language pack so that it can support multiple languages. In Laravel, language packages are usually placed in the resources/lang directory, where each language
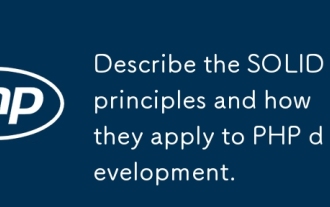
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
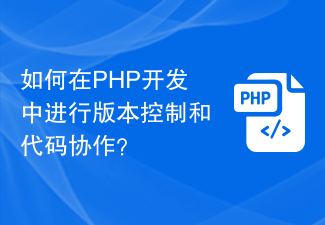
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you

How to implement permission-based multi-language support in Laravel Introduction: In modern websites and applications, multi-language support is a very common requirement. For some complex systems, we may also need to dynamically display different language translations based on the user's permissions. Laravel is a very popular PHP framework that provides many powerful features to simplify the development process. This article will introduce how to implement permission-based multi-language support in Laravel and provide specific code examples. Step 1: Configure multi-language support first
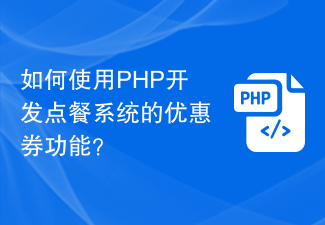
How to use PHP to develop the coupon function of the ordering system? With the rapid development of modern society, people's life pace is getting faster and faster, and more and more people choose to eat out. The emergence of the ordering system has greatly improved the efficiency and convenience of customers' ordering. As a marketing tool to attract customers, the coupon function is also widely used in various ordering systems. So how to use PHP to develop the coupon function of the ordering system? 1. Database design First, we need to design a database to store coupon-related data. It is recommended to create two tables: one
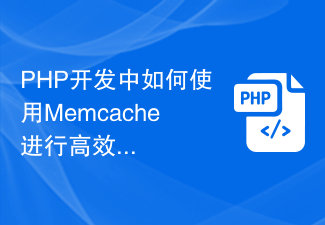
How to use Memcache for efficient data writing and querying in PHP development? With the continuous development of Internet applications, the requirements for system performance are getting higher and higher. In PHP development, in order to improve system performance and response speed, we often use various caching technologies. One of the commonly used caching technologies is Memcache. Memcache is a high-performance distributed memory object caching system that can be used to cache database query results, page fragments, session data, etc. By storing data in memory
