


How to develop geolocation verification for online employee attendance using PHP and Vue
How to use PHP and Vue to develop geolocation verification of online employee attendance
Introduction:
With the development of information technology, more and more companies tend to Yu adopts an online employee attendance system to replace the traditional paper attendance method. However, many companies still face many problems with employee attendance, one of which is the inability to ensure that employees are actually at their workplace during working hours. In order to solve this problem, this article will introduce how to use PHP and Vue to develop an online employee attendance system and use the geographical location verification function.
1. Technical preparation
To start developing this online employee attendance system, we need to prepare the following technologies and tools:
- PHP programming language: used for backend Develop, process data and logic controls.
- Vue.js framework: used for front-end development and building user interfaces.
- MySQL database: used to store employee, attendance records and other data.
- HTML/CSS/JavaScript: used for page rendering and interactive operations.
- Geolocation service API: used to obtain the geographical location information of the user's device.
2. Project construction and environment configuration
- Install the PHP environment: Set up the PHP environment locally or on the server to ensure that the PHP program can run normally.
- Install MySQL database: Create a new database and import the required data table structure.
- Create Vue project: Use Vue CLI to create a new Vue project and configure routing and components.
3. Implement employee attendance system
- Add a new employee: Use PHP to write an interface on the backend, receive the employee’s basic information through a POST request, and insert it into the database .
Sample code:
<?php // 处理添加员工接口 if ($_SERVER['REQUEST_METHOD'] === 'POST') { $name = $_POST['name']; $position = $_POST['position']; // 其他员工信息的获取 // 将员工信息插入数据库 $sql = "INSERT INTO employees (name, position) VALUES ('$name', '$position')"; // 执行SQL语句 } ?>
- Get the geographical location: Use the HTML5 geolocation API to obtain the user's geographical location information.
Sample code:
if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(showPosition); } else { console.log("浏览器不支持地理定位。"); } function showPosition(position) { var latitude = position.coords.latitude; var longitude = position.coords.longitude; // 其他操作,比如发送地理位置到后端 }
- Verification of geographical location: Use PHP to write an interface on the back end, receive the geographical location information sent by the front end, and compare it with the employee's working location.
Sample code:
<?php // 处理地理位置验证接口 if ($_SERVER['REQUEST_METHOD'] === 'POST') { $latitude = $_POST['latitude']; $longitude = $_POST['longitude']; // 其他操作,比如获取员工的工作地点 // 比对地理位置 if (checkLocation($latitude, $longitude, $workplace_latitude, $workplace_longitude)) { // 地理位置验证通过 } else { // 地理位置验证失败 } } function checkLocation($lat1, $lng1, $lat2, $lng2) { // 使用经纬度计算两点之间距离 // 如果距离小于等于阈值,返回true,否则返回false } ?>
- Display attendance records: Use Vue.js to display employee attendance records on the front end, including attendance time, location and other information.
Sample code:
<template> <div> <h2 id="employeeName-的考勤记录">{{ employeeName }}的考勤记录</h2> <ul> <li v-for="attendance in attendances" :key="attendance.id"> {{ attendance.time }} - {{ attendance.location }} </li> </ul> </div> </template> <script> export default { data() { return { employeeName: '员工名称', attendances: [ { id: 1, time: '2022-01-01 09:00:00', location: '工作地点A' }, { id: 2, time: '2022-02-01 09:30:00', location: '工作地点B' }, // 其他考勤记录数据 ] } } } </script>
IV. Summary
This article introduces how to use PHP and Vue to develop an online employee attendance system and implement the geographical location verification function. Obtain the user's current geographical location through the geolocation API, and then compare it with the employee's work location to ensure that the employee is actually at the work location during working hours. Such an online attendance system can improve the accuracy and reliability of attendance and provide more convenience for company management.
However, it should be noted that during the development process, issues such as data security, rights management, and compatibility of different devices and browsers also need to be considered. I hope this article will be helpful to developers who are developing online employee attendance systems, and that readers can make appropriate adjustments and optimizations according to specific needs in actual projects.
The above is the detailed content of How to develop geolocation verification for online employee attendance using PHP and Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










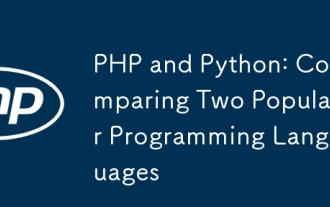
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
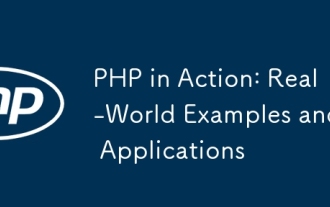
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
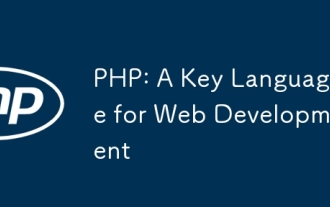
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
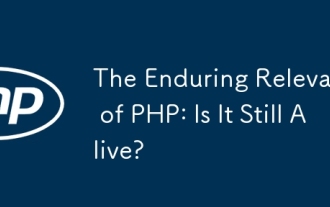
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
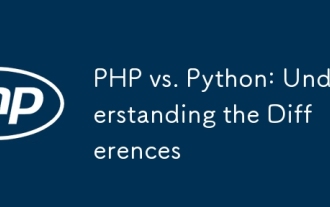
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
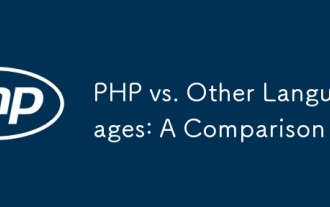
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
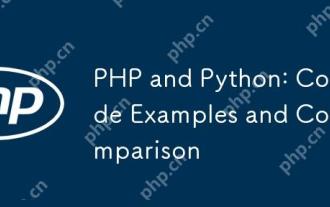
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
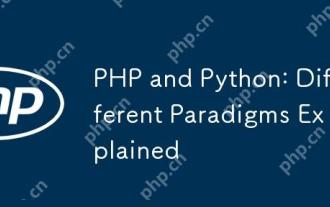
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
