How to write custom functions in MySQL using C#
How to write custom functions in MySQL using C
#MySQL is a popular relational database management system, and C# is a powerful programming language. In MySQL, you can use C# to write custom functions to enhance the functionality of the database. This article will introduce how to use C# to write custom functions in MySQL through specific code examples.
Before you begin, make sure you have installed the MySQL database and C# development environment.
Step one: Create a C# class library project
First, we need to create a C# class library project. Open Visual Studio (or other C# development tools), select "New Project", then select the "Class Library" template, name it "MySQLUdf", and click "OK".
Step 2: Add MySQL Connector/Net reference
In the C# class library project, you need to add a MySQL Connector/Net reference in order to connect and operate the MySQL database. In Visual Studio, right-click References, select Manage NuGet Packages, enter "MySQL Connector/Net" in the search box, and click Install.
Step 3: Write the code of the custom function
In the C# class library project, open the "Class1.cs" file and rename it "MySQLUdf.cs". Then, paste the following code into the file.
using MySql.Data.MySqlClient; public class MySQLUdf { [System.ComponentModel.DataAnnotations.Schema.DbFunction("MySQL", "MyFunc")] public static int MyFunc(int arg1, int arg2) { // 定义数据库连接字符串 string connStr = "server=yourServerAddress;user id=yourUserId;password=yourPassword;database=yourDatabase;"; // 创建数据库连接对象 using (MySqlConnection conn = new MySqlConnection(connStr)) { try { // 打开数据库连接 conn.Open(); // 创建MySQL命令对象 using (MySqlCommand cmd = conn.CreateCommand()) { // 设置命令文本和参数 cmd.CommandText = "SELECT @arg1 + @arg2"; cmd.Parameters.AddWithValue("@arg1", arg1); cmd.Parameters.AddWithValue("@arg2", arg2); // 执行SQL语句并返回结果 int result = (int)cmd.ExecuteScalar(); return result; } } catch (Exception ex) { // 处理异常 throw ex; } } } }
The above code defines a custom function named "MyFunc", which accepts two integer parameters and returns their sum. Inside the function, the database connection string is first defined, and then a database connection object is created using the MySqlConnection class. Next, use the MySqlCommand class to create a SQL command object and set the command text and parameters. Finally, use the cmd.ExecuteScalar() method to execute the SQL statement and return the results.
Step 4: Generate class library project
Save and compile the C# class library project and generate class library files.
Step 5: Create a custom function in the MySQL database
In the MySQL database, you need to create the stored procedures and functions of the custom function.
Suppose we have created a database named "test" in the MySQL database.
In the MySQL database, open the command line tool or client and execute the following command:
CREATE PROCEDURE `test`.`my_func`(IN `arg1` INT, IN `arg2` INT, OUT `result` INT) BEGIN SET @result = CAST(0 AS INT); SELECT MySQLUdf.MyFunc(arg1, arg2) INTO result; END
The above command creates a stored procedure "my_func", which accepts two integer parameters "arg1" and "arg2", and outputs an integer result "result". In the stored procedure, the SELECT statement is used to call the custom function "MySQLUdf.MyFunc" we previously defined in the C# class library project.
Step 6: Test the custom function
In the MySQL database, execute the following command:
CALL my_func(1, 2, @result); SELECT @result;
The above command calls the stored procedure "my_func" we just created, Pass in parameters 1 and 2 and store the result in the variable "@result". Then, use a SELECT statement to display the results.
If everything is OK, you will see the output result is 3.
Summary:
This article introduces how to use C# to write custom functions in MySQL. By creating a C# class library project, adding a MySQL Connector/Net reference, and writing the code for the custom function, and then creating stored procedures and functions in the MySQL database, you can use custom functions written in C# in the MySQL database. Hope this article helps you!
The above is the detailed content of How to write custom functions in MySQL using C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
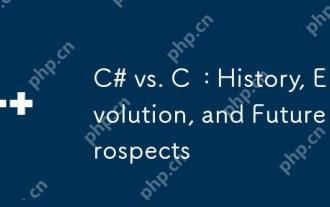
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
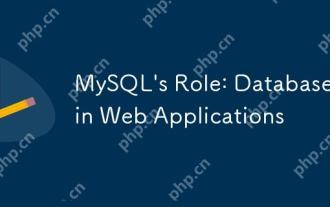
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
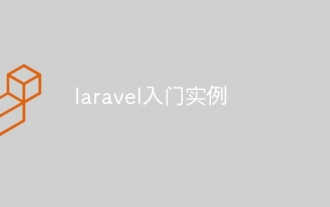
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
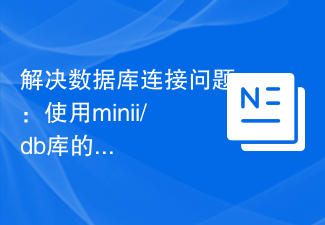
I encountered a tricky problem when developing a small application: the need to quickly integrate a lightweight database operation library. After trying multiple libraries, I found that they either have too much functionality or are not very compatible. Eventually, I found minii/db, a simplified version based on Yii2 that solved my problem perfectly.
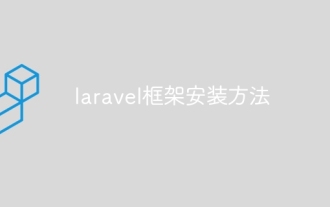
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.

MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
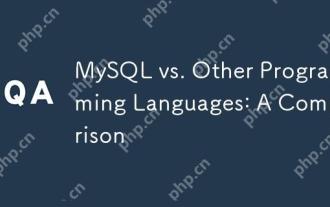
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
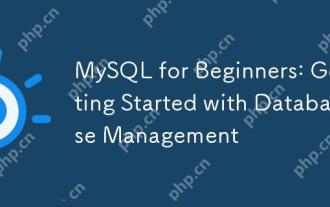
The basic operations of MySQL include creating databases, tables, and using SQL to perform CRUD operations on data. 1. Create a database: CREATEDATABASEmy_first_db; 2. Create a table: CREATETABLEbooks(idINTAUTO_INCREMENTPRIMARYKEY, titleVARCHAR(100)NOTNULL, authorVARCHAR(100)NOTNULL, published_yearINT); 3. Insert data: INSERTINTObooks(title, author, published_year)VA
