How to implement the red-black tree algorithm in C#
How to implement the red-black tree algorithm in C# requires specific code examples
Introduction:
The red-black tree is a self-balancing binary search tree . It maintains the specific property such that for any valid red-black tree, the longest path is never more than twice the shortest path. This characteristic makes red-black trees have better performance in insertion, deletion and search operations. This article will introduce how to implement the red-black tree algorithm in C# and provide specific code examples.
Properties of red-black trees:
Red-black trees have the following 5 properties:
- Each node is either red or black.
- The root node is black.
- Each leaf node (NIL node, empty node) is black.
- If a node is red, both of its child nodes are black.
- For each node, the simple path from that node to all its descendant leaf nodes contains the same number of black nodes.
Implementation of red-black tree:
The following is a sample code to implement red-black tree in C#:
enum Color { Red, Black } class Node { public int key; public Node parent; public Node left; public Node right; public Color color; public Node(int key) { this.key = key; color = Color.Black; } } class RedBlackTree { private Node root; private void Insert(Node newNode) { Node current = root; Node parent = null; while (current != null) { parent = current; if (newNode.key < current.key) current = current.left; else current = current.right; } newNode.parent = parent; if (parent == null) root = newNode; else if (newNode.key < parent.key) parent.left = newNode; else parent.right = newNode; newNode.color = Color.Red; FixAfterInsertion(newNode); } private void FixAfterInsertion(Node newNode) { while (newNode != root && newNode.parent.color == Color.Red) { if (newNode.parent == newNode.parent.parent.left) { Node uncle = newNode.parent.parent.right; if (uncle != null && uncle.color == Color.Red) { newNode.parent.color = Color.Black; uncle.color = Color.Black; newNode.parent.parent.color = Color.Red; newNode = newNode.parent.parent; } else { if (newNode == newNode.parent.right) { newNode = newNode.parent; RotateLeft(newNode); } newNode.parent.color = Color.Black; newNode.parent.parent.color = Color.Red; RotateRight(newNode.parent.parent); } } else { Node uncle = newNode.parent.parent.left; if (uncle != null && uncle.color == Color.Red) { newNode.parent.color = Color.Black; uncle.color = Color.Black; newNode.parent.parent.color = Color.Red; newNode = newNode.parent.parent; } else { if (newNode == newNode.parent.left) { newNode = newNode.parent; RotateRight(newNode); } newNode.parent.color = Color.Black; newNode.parent.parent.color = Color.Red; RotateLeft(newNode.parent.parent); } } } root.color = Color.Black; } private void RotateLeft(Node node) { Node right = node.right; node.right = right.left; if (right.left != null) right.left.parent = node; right.parent = node.parent; if (node.parent == null) root = right; else if (node == node.parent.left) node.parent.left = right; else node.parent.right = right; right.left = node; node.parent = right; } private void RotateRight(Node node) { Node left = node.left; node.left = left.right; if (left.right != null) left.right.parent = node; left.parent = node.parent; if (node.parent == null) root = left; else if (node == node.parent.right) node.parent.right = left; else node.parent.left = left; left.right = node; node.parent = left; } // 其他方法:查找、删除等 // ... }
Summary:
This article introduces how to implement red-black tree in C# Implement the red-black tree algorithm and provide detailed code examples. Red-black tree is a self-balancing binary search tree with better performance in insertion, deletion and search operations. By using red-black trees, we can solve some common problems more efficiently. In practical applications, we can appropriately adjust and expand the functions of the red-black tree as needed. I hope this article will be helpful to you and spark your interest and in-depth research on red-black trees.
The above is the detailed content of How to implement the red-black tree algorithm in C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
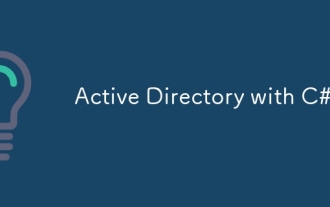
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
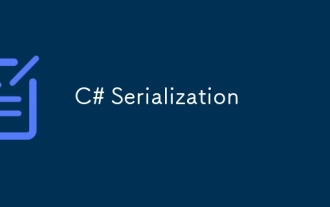
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
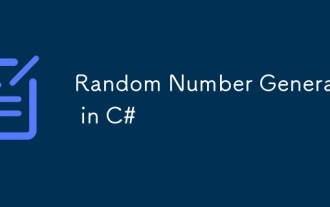
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
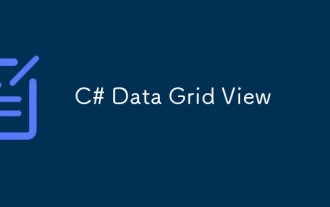
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
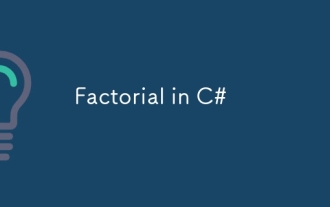
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
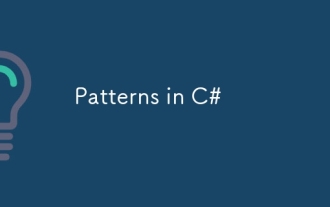
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
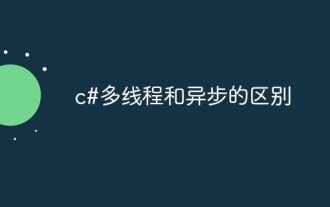
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
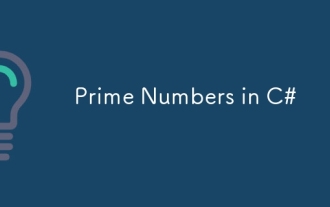
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
