Use Vue Router to implement role-based redirection control
Use Vue Router to implement role-based redirection control
When developing web applications, we often encounter situations where access permissions need to be restricted based on user roles. Vue Router is a very convenient routing management library that can help us implement routing functions in Vue.js applications. This article will introduce how to use Vue Router to implement role-based redirection control and provide specific code examples.
First, we need to install and configure Vue Router. Vue Router can be installed via npm or yarn:
npm install vue-router
After installation, introduce Vue Router in the main Vue.js instance:
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter)
Then, we need to define the routing configuration of the application. In the routing configuration, we can specify the path, components, and required permission roles of each route.
const routes = [ { path: '/', name: 'Home', component: Home, meta: { requiresAuth: true, requiredRoles: ['admin', 'user'] } }, { path: '/admin', name: 'Admin', component: Admin, meta: { requiresAuth: true, requiredRoles: ['admin'] } }, { path: '/user', name: 'User', component: User, meta: { requiresAuth: true, requiredRoles: ['user'] } }, { path: '/login', name: 'Login', component: Login } ]
In the above code, we defined four routes, namely home page (Home), administrator page (Admin), user page (User) and login page (Login). For routes that require permission control, we use the meta field to specify the required permission roles. In this example, the 'admin' role can access the administrator page, the 'user' role can access the user page, and the homepage requires permissions from both 'admin' and 'user' roles.
Next, we need to configure routing in the Vue Router instance:
const router = new VueRouter({ routes })
Then, we can use Vue Router’s global front guard to implement role-based redirection control. Before each route navigation, we check whether the user's role meets the roles required to access the route.
router.beforeEach((to, from, next) => { const requiresAuth = to.matched.some(record => record.meta.requiresAuth) const requiredRoles = to.meta.requiredRoles const currentUser = getUserFromLocalStorage() if (requiresAuth && !currentUser) { next({ path: '/login', query: { redirect: to.fullPath } }) } else if (requiresAuth && !requiredRoles.includes(currentUser.role)) { next({ path: '/', query: { redirect: to.fullPath } }) } else { next() } }) function getUserFromLocalStorage() { // 从本地存储中获取当前用户的角色信息 // 这里需要根据你的实际情况来实现 // 例如从 cookie 或 sessionStorage 中获取 return { role: 'admin' } }
In the above code, we implement permission control through the beforeEach guard. First, we check if the user is logged in (requiresAuth) and redirect to the login page if not, passing the path of the current page as the redirect parameter (query.redirect). We then check if the user's role meets the roles required to access the route, and if the role does not meet the requirements, redirect to the homepage.
Finally, we need to mount the routing instance into the Vue.js application:
new Vue({ router, render: h => h(App) }).$mount('#app')
At this point, we have completed the process of implementing role-based redirection control using Vue Router. With the above code example, we can restrict a user's access to different pages based on their role. This enables more flexible and secure web application development.
The above is the detailed content of Use Vue Router to implement role-based redirection control. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
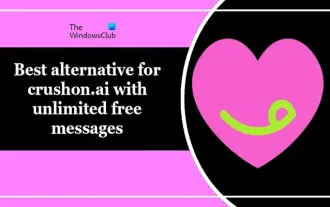
In this article we will introduce you to the best alternatives to CrushOn.AI with free and unlimited messaging capabilities. There are many artificial intelligence platforms on the market now that allow users to talk to characters from various media such as animation, which provides users with a more interesting and interactive experience. What is CrushOn.AI? CrushonAI is an AI chatbot platform that allows users to experience the fun of interaction by having conversations with virtual characters. Users have the opportunity to communicate with, build connections with, and create storylines related to their favorite characters across a variety of media including anime. The best alternative to CrushOn.AI that offers unlimited free messages If you are looking for the best Crush
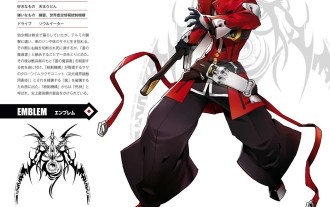
Blue Wings Chaos Effect features a diverse cast of characters, each with a unique identity and backstory. For this reason, the editor has specially compiled an introduction to the characters of BlazBlue Chaos Effect for all players. How many characters are there in BlazBlue Chaos Effect? Answer: There are 7 characters. 1. [God of Death] Ragnar Chad Bradedge (nicknamed RG, Nissan), his brother and sister were raised by church nuns. One day, one of the six heroes of the villain broke in, killed the nuns, and burned them down. Church, took his sister away, leaving behind his younger brother "The Weapon of Things" Ice Sword Snow Girl. 2. Noel Vermillion The adopted daughter of the Vermillion family looks almost the same as Ragnar's sister. After graduation, he joined the governing body as secretary to Ragnar's younger brother. 3. λ-11 is collectively known as Lambda and Eleventh Sister. After the original developer gave up, Kokonoe rescued and
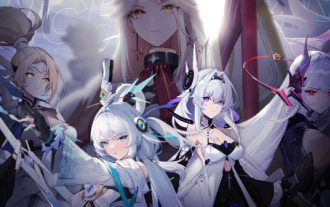
Anchor Arrival is a 3D turn-based card game with a high-definition beautiful girl two-dimensional theme. It provides a rich and exciting combination of characters for players to explore and experience. It has many powerful combinations of high-quality lineups. New players are also curious novices. What powerful characters are recommended in the pool? Let’s take a look at the selection reference for novices to win ten consecutive golds! Anchor Point Advent is a powerful character recommendation for novice pools. The first ten-consecutive pick is Alice. She is mainly a single-target lightning-type burst character. The output is very explosive, and the experience will be very friendly to newcomers, so it is highly recommended to choose it. It is recommended to choose the combination of "Alice" + "Antelope" for 10 points. Alice is the most worthy character to output the goldpire attribute, and is not even a bit stronger than the other two characters in the novice card pool. Alice can pass special
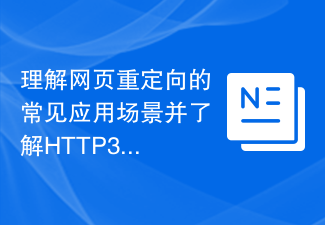
Understand the meaning of HTTP 301 status code: common application scenarios of web page redirection. With the rapid development of the Internet, people's requirements for web page interaction are becoming higher and higher. In the field of web design, web page redirection is a common and important technology, implemented through the HTTP 301 status code. This article will explore the meaning of HTTP 301 status code and common application scenarios in web page redirection. HTTP301 status code refers to permanent redirect (PermanentRedirect). When the server receives the client's
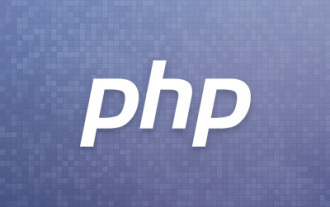
Redirects allow you to redirect client browsers to different URLs. You can use it when switching domains, changing website structure, or switching to HTTPS. In this article, I will show you how to redirect to another page using PHP. I'll explain exactly how PHP redirects work and show you what's happening behind the scenes. Learn PHP with Free Online Courses If you want to learn PHP, check out our PHP Basics free online course! PHP Basics Jeremy McPeak October 29, 2021 How do basic redirects work? Before we get into the details of PHP redirection, let’s take a quick look at how HTTP redirection actually works. Take a look at the image below. Let us understand the above screen
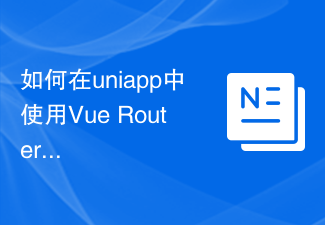
How to use VueRouter for routing jumps in uniapp Using VueRouter for routing jumps in uniapp is a very common operation. This article will introduce in detail how to use VueRouter in the uniapp project and provide specific code examples. 1. Install VueRouter Before using VueRouter, we need to install it first. Open the command line, enter the root directory of the uniapp project, and then execute the following command to install
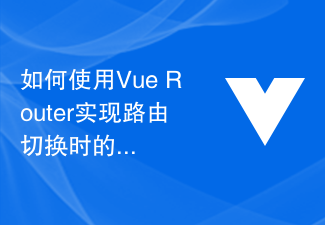
How to use VueRouter to achieve transition effect when routing switching? Introduction: VueRouter is a routing management library officially recommended by Vue.js for building SPA (SinglePageApplication). It can achieve switching between pages by managing the correspondence between URL routing and components. In actual development, in order to improve user experience or meet design needs, we often use transition effects to add dynamics and beauty to page switching. This article will introduce how to use
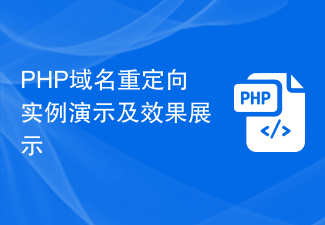
PHP domain name redirection is one of the commonly used technologies in website development. Through domain name redirection, users can automatically jump to another URL when visiting one URL, thereby achieving website traffic guidance, brand promotion and other purposes. The following will use a specific example to demonstrate the implementation method of PHP domain name redirection and show the effect. Create a simple PHP file named redirect.php with the following code:
