


In-depth analysis of the underlying development principles of PHP7: Discussing the implementation principles of PHP exception handling
In-depth analysis of the underlying development principles of PHP7: Discussing the implementation principles of PHP exception handling
Introduction:
Exception handling is one of the very important features in modern programming languages , which provides an elegant and efficient way to handle errors and exceptions that may occur during program execution. In PHP7, the exception handling mechanism has been greatly improved and optimized. This article will deeply explore the implementation principles of PHP exception handling.
1. Introduction to exception handling mechanism
Exception handling is a mechanism that captures and handles errors and exceptions during program execution. In PHP, the exception handling mechanism consists of try-catch-finally statement blocks. The code segment contains code that may throw exceptions. When an exception occurs, the program will jump to the corresponding catch block for exception handling.
try {
// 可能抛出异常的代码
} catch (Exception $e) {
// 异常处理代码
} finally {
// 最终执行代码
}
In the above code block, the code in the try block is the code segment that may throw an exception. When an exception is thrown, the code in the catch block will be checked in turn to find the catch block that matches the exception type for processing. If no matching catch block is found, the exception will be passed up the call stack.
2. Implementation Principle of Exception Handling
The implementation principle of PHP exception handling involves some key mechanisms underlying PHP.
- Definition and inheritance of exception classes
In PHP, exception classes are used to represent specific types of errors and exceptions. We can customize exception classes and inherit from Exception or Error classes to better describe and handle different types of errors and exceptions.
class MyException extends Exception {
// 自定义异常类
}
- Throw exception
In PHP, we can use the throw keyword to throw an exception abnormal. When a certain condition is not met, we can throw an exception to interrupt the normal execution of the program.
function divide($numerator, $denominator) {
if ($denominator == 0) { throw new Exception('除数不能为零'); } return $numerator / $denominator;
}
- Exception catching and handling
When an exception is thrown, the program Will jump to the nearest catch block for exception handling. Catch blocks are checked in order until one is found that matches the type of exception thrown.
try {
echo divide(10, 0);
} catch (Exception $e) {
echo '捕获到异常:' . $e->getMessage();
}
In the above code, when "divisor" is thrown Cannot be zero" exception, "Caught exception: divisor cannot be zero" will be displayed in the catch block.
- Exception delivery
If a suitable catch block is not found in the current scope to handle the thrown exception, the exception will be passed to the caller's scope until a matching catch block is found. Or reach the top-level global scope.
function foo() {
try { bar(); } catch (Exception $e) { echo '捕获到异常:' . $e->getMessage(); }
}
function bar() {
throw new Exception('抛出异常');
}
In the above code , the thrown exception is passed from the bar function to the foo function, and is finally caught in the catch block of the foo function.
- Execution of finally block
The code in the finally block will always be executed after the exception is caught and handled. Regardless of whether an exception occurs, the code in the finally block will be executed.
try {
echo divide(10, 2);
} catch (Exception $e) {
echo '捕获到异常:' . $e->getMessage();
} finally {
echo '最终执行';
}
In the above code, regardless of whether the divide function throws an exception, "final execution" will be output.
Conclusion:
Through the introduction of this article, we understand the implementation principle of PHP exception handling. The exception handling mechanism can effectively help us capture and handle errors and exceptions during program execution, making the program more stable and robust. During the development process, we can customize exception classes according to actual needs to better describe and handle different types of errors and exceptions.
Reference:
- PHP Manual: http://php.net/manual/en/language.exceptions.php
The above is the detailed content of In-depth analysis of the underlying development principles of PHP7: Discussing the implementation principles of PHP exception handling. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










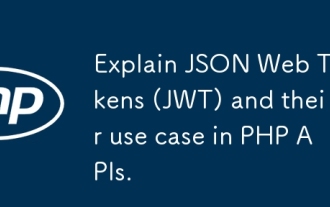
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
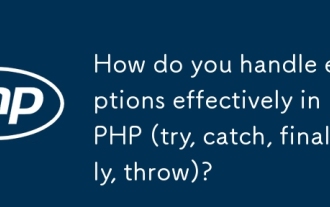
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
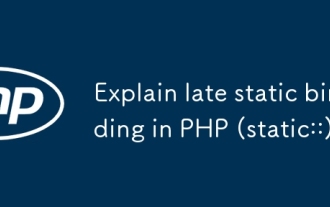
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
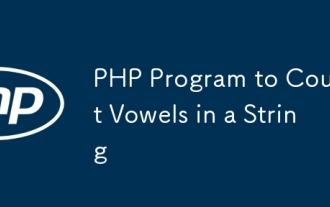
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
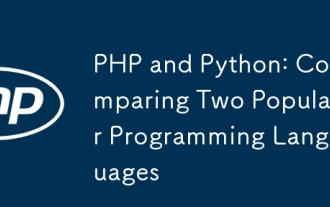
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
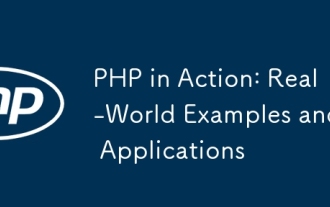
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
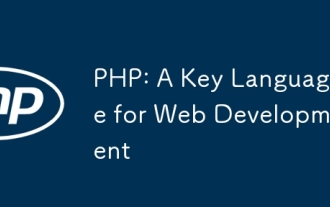
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
