


How to speed up Python website access through code optimization?
How to speed up Python website access through code optimization?
With the rapid development of the Internet, website access speed is crucial to user experience and search engine optimization. Writing efficient code can speed up your Python website. This article will introduce some optimization tips and code examples to improve the performance of Python websites.
- Use appropriate data structures
Choosing appropriate data structures can reduce code complexity and speed up access. For example, use a Dictionary rather than a List to store large numbers of key-value pairs because dictionary lookups are much faster.
Sample code:
# 使用字典存储键值对 my_dict = {"key1": "value1", "key2": "value2"} # 使用列表存储数据 my_list = [1, 2, 3, 4, 5]
- Reduce the number of network requests
Reducing the website's dependence on external resources can significantly reduce the number of network requests, thereby improving access speed. Static resources, such as JavaScript and CSS files, can be served by combining them into a single file or using a CDN (Content Delivery Network).
Sample code:
<!-- 将多个CSS文件合并成一个文件 --> <link rel="stylesheet" href="style.css"> <!-- 使用CDN提供的JavaScript库 --> <script src="https://cdn.example.com/jquery.min.js"></script>
- Use cache
Reasonable use of cache can avoid repeated calculations and database queries, thereby improving the response speed of the website. Data and static files can be cached using an in-memory cache (such as Memcached or Redis) or a browser cache.
Sample code:
import time import functools from flask import Flask from flask_caching import Cache app = Flask(__name__) cache = Cache(app) @app.route("/") @cache.cached(timeout=60) # 缓存结果60秒 def index(): time.sleep(5) # 模拟一个耗时的操作 return "Hello World" if __name__ == "__main__": app.run()
- Using asynchronous programming
Using asynchronous programming can make full use of system resources, thereby improving concurrent processing capabilities. Asynchronous code can be written using Python's asynchronous frameworks such as asyncio or aiohttp.
Sample code:
import asyncio from aiohttp import web async def handle(request): await asyncio.sleep(5) # 模拟一个耗时的操作 return web.Response(text="Hello World") app = web.Application() app.router.add_get('/', handle) if __name__ == "__main__": web.run_app(app)
- Optimize database query
Database query is usually one of the bottlenecks of website performance. The speed of database queries can be improved by properly selecting indexes, optimizing SQL statements, and using caching and other techniques.
Sample code:
import sqlite3 # 使用索引来加快查询速度 conn = sqlite3.connect(":memory:") cursor = conn.cursor() cursor.execute("CREATE INDEX IF NOT EXISTS index_name ON my_table (column_name)") # 优化SQL语句来减少查询时间 cursor.execute("SELECT column1, column2 FROM my_table WHERE column3 = ? LIMIT 10", (value,)) # 使用缓存来避免重复查询 data = cache.get("my_key") if data is None: data = db.query("SELECT * FROM my_table") cache.set("my_key", data, timeout=60)
Through the optimization of the above aspects, the access speed of the Python website can be significantly improved. However, performance optimizations need to be adjusted on a case-by-case basis, with appropriate trade-offs to avoid over-optimization that results in increased code complexity. I hope the optimization tips and code examples in this article can be helpful in improving Python website performance.
The above is the detailed content of How to speed up Python website access through code optimization?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
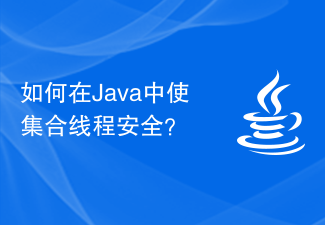
Collections class that specializes in handling java.util package methods of collections, which provide various additional operations involving polymorphic algorithms. This class provides different variants of the synchronizedCollection() method as follows - Sr.No method and description 1 Static collection synchronizedCollection(Collectionc) This method accepts any collection object and returns a synchronized (thread-safe) collection backed by the specified collection . 2 Static list synchronizedList (List list) This method accepts an object of the List interface and returns a synchronized (thread-safe) list supported by the specified list. 3Static map

How to use the caching function in the Yii framework Caching is a common performance optimization method that can significantly improve the response speed of a website or application. The Yii framework provides powerful caching functions that can help us simplify the cache use and management process. This article will introduce how to use the caching function in the Yii framework and provide some code examples. 1. Caching Component in Yii framework The caching function in Yii framework consists of a component called caching component (Cac

How to solve performance optimization problems encountered in Java Introduction: When developing Java applications, performance optimization is an important requirement. Optimizing the performance of your code can improve program responsiveness and efficiency, thereby improving user experience and system scalability. However, many Java developers are often confused when facing performance issues. This article will explore some common performance optimization issues and provide some solutions to help developers better solve performance problems in Java. 1. Problem identification and analysis in solving performance problems
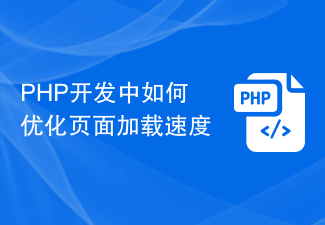
With the continuous development of Internet technology, the requirements for website page loading speed are also getting higher and higher. As a PHP developer, we need to know some optimization methods to ensure that the page loads quickly and improve the user experience. The following will introduce you to several common PHP page optimization techniques. Using Caches Caching is a technique for storing data in temporary storage for quick access. In PHP, we can use memory caching systems such as Memcached and Redis to store frequently used pages
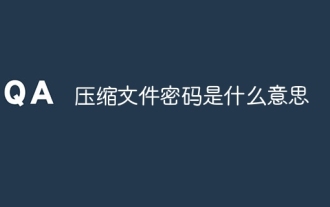
The purpose of setting a password for compressed files is to prevent others from stealing links. Generally, the password will be displayed on the right side of the compressed package. If not, you must find it on the download page, or directly ask the person who posted the file. File encryption can be divided into two categories according to the encryption method: 1. It is performed through the system’s own function. Encryption; 2. Use third-party software for encryption.
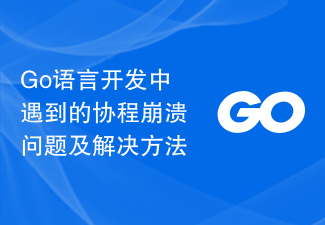
Concurrent coroutine crash problems encountered in Go language development and solutions Introduction: In the Go language development process, using concurrent coroutines (Goroutine) is a common way to implement concurrently running code. However, concurrent coroutines sometimes crash, causing the program to fail to run properly. This article will explore some common concurrent coroutine crash problems and provide solutions. 1. The crash problem of concurrent coroutines: Unhandled exceptions: Exceptions in concurrent coroutines may cause crashes. When an exception occurs in the coroutine but is not handled correctly
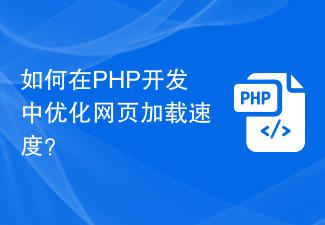
How to optimize web page loading speed in PHP development? With the rapid development of the Internet, web page loading speed has become more and more important to user experience. When a web page loads slowly, users often choose to close the page or leave the website. Therefore, optimizing web page loading speed is a very important task for PHP developers. Here are some ways to optimize web page loading speed. 1. Use cache In PHP development, using cache is one of the simplest and most effective ways to improve the loading speed of web pages. Various caching techniques can be used,
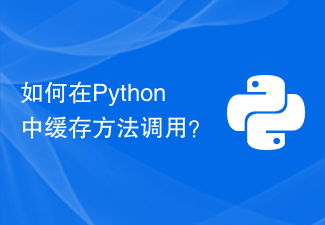
Two tools for caching methods are functools.cached_property() and functools.lru_cache(). Both modules are part of the functools module. The functools module is used for higher-order functions: functions that act on or return other functions. Let us first install and import the functools module - install functools To install functools module use pip − pip install functools import function tools To import functools − import functools Let us understand cache one by one - cached_property
