


PHP form processing: using cookies to implement the remember me function
PHP form processing: using cookies to implement the remember me function
In web development, we often encounter user login situations. In order to improve user experience, we can use Cookie technology to implement the "remember me" function so that users do not need to log in again the next time they visit the webpage. This article will introduce how to use PHP to process forms and use cookies to achieve this function.
- HTML Form Design
First, we need to create an HTML form that allows users to enter their username and password, and provides a checkbox for users to choose whether to remember their login status.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>登录</title> </head> <body> <form action="login.php" method="POST"> <label for="username">用户名:</label> <input type="text" id="username" name="username"><br><br> <label for="password">密码:</label> <input type="password" id="password" name="password"><br><br> <label for="remember">记住我:</label> <input type="checkbox" id="remember" name="remember"><br><br> <input type="submit" value="登录"> </form> </body> </html>
- PHP form processing
Create a PHP file namedlogin.php
to process the data of the login form.
<?php if($_SERVER['REQUEST_METHOD'] == 'POST'){ // 获取表单提交的用户名和密码 $username = $_POST['username']; $password = $_POST['password']; // 验证用户名和密码是否正确 if($username == 'admin' && $password == '123456'){ // 如果用户选择记住登录状态,则设置Cookie保存用户名和密码 if(isset($_POST['remember'])){ setcookie('username', $username, time()+3600*24*7); // 保存7天 setcookie('password', $password, time()+3600*24*7); } // 登录成功后,跳转到其他页面 header("Location: welcome.php"); }else{ echo '用户名或密码错误!'; } } ?>
In the above code, use the $_POST
array to get the username and password in the form. Then, by comparing it with the preset user name and password, it is judged whether the user input is correct. If the username and password are correct, then determine whether the user selected the "Remember Me" option. If this option is selected, the Cookie is set through the setcookie
function, where we save the username and password, which is valid for 7 days.
Finally, redirect the user to the welcome.php
page through the header
function. If the login fails, an error message will be output.
- Welcome page
Create a PHP file namedwelcome.php
to display the welcome page after the user successfully logs in. In this page, we can welcome the user based on the username in the cookie.
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>欢迎</title> </head> <body> <?php if(isset($_COOKIE['username'])){ $username = $_COOKIE['username']; echo '<h1 id="欢迎回来-username">欢迎回来,'.$username.'!</h1>'; }else{ echo '<h1 id="请先登录">请先登录!</h1>'; } ?> </body> </html>
In the above code, use isset($_COOKIE['username'])
to determine whether the user name is saved in the cookie. If it exists, get the user name through $_COOKIE['username']
, and output the welcome message on the page. Otherwise, prompt the user to log in first.
Through the above steps, we can implement a simple PHP login form and use Cookie to implement the "remember me" function. When the user checks the "Remember Me" option and logs in successfully, they will automatically log in the next time they visit the webpage.
The above is the detailed content of PHP form processing: using cookies to implement the remember me function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
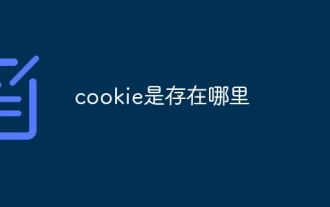
Cookies are usually stored in the cookie folder of the browser. Cookie files in the browser are usually stored in binary or SQLite format. If you open the cookie file directly, you may see some garbled or unreadable content, so it is best to use Use the cookie management interface provided by your browser to view and manage cookies.
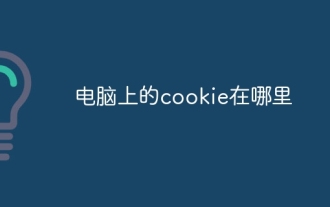
Cookies on your computer are stored in specific locations on your browser, depending on the browser and operating system used: 1. Google Chrome, stored in C:\Users\YourUsername\AppData\Local\Google\Chrome\User Data\Default \Cookies etc.
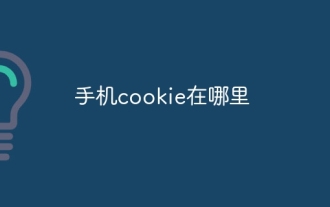
Cookies on the mobile phone are stored in the browser application of the mobile device: 1. On iOS devices, Cookies are stored in Settings -> Safari -> Advanced -> Website Data of the Safari browser; 2. On Android devices, Cookies Stored in Settings -> Site settings -> Cookies of Chrome browser, etc.
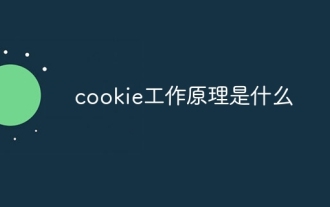
The working principle of cookies involves the server sending cookies, the browser storing cookies, and the browser processing and storing cookies. Detailed introduction: 1. The server sends a cookie, and the server sends an HTTP response header containing the cookie to the browser. This cookie contains some information, such as the user's identity authentication, preferences, or shopping cart contents. After the browser receives this cookie, it will be stored on the user's computer; 2. The browser stores cookies, etc.
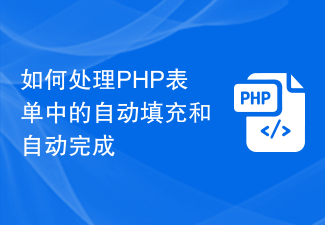
How to Handle Autofill and Autocomplete in PHP Forms As the Internet develops, people increasingly rely on autofill and autocomplete features to simplify their operations on the website. Implementing these functions in PHP forms is not complicated. This article will briefly introduce how to use PHP to handle auto-fill and auto-complete of forms. Before we begin, we need to clarify what autofill and autocomplete are. Autofill refers to automatically filling in the fields in a form for users based on their previous input or history. For example, when the user enters an email
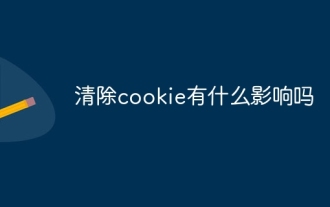
The effects of clearing cookies include resetting personalization settings and preferences, affecting ad experience, and destroying login status and password remembering functions. Detailed introduction: 1. Reset personalized settings and preferences. If cookies are cleared, the shopping cart will be reset to empty and products need to be re-added. Clearing cookies will also cause the login status on social media platforms to be lost, requiring re-adding. Enter your username and password; 2. It affects the advertising experience. If cookies are cleared, the website will not be able to understand our interests and preferences, and will display irrelevant ads, etc.
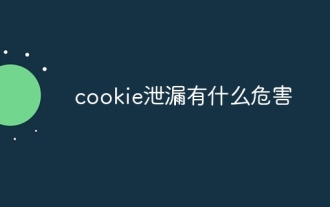
The dangers of cookie leakage include theft of personal identity information, tracking of personal online behavior, and account theft. Detailed introduction: 1. Personal identity information is stolen, such as name, email address, phone number, etc. This information may be used by criminals to carry out identity theft, fraud and other illegal activities; 2. Personal online behavior is tracked and analyzed through cookies With the data in the account, criminals can learn about the user's browsing history, shopping preferences, hobbies, etc.; 3. The account is stolen, bypassing login verification, directly accessing the user's account, etc.
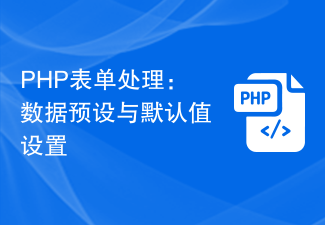
PHP form processing: data presets and default value settings When developing web applications, forms are an inevitable part. When a user submits a form, we need to process this data and act accordingly. This article will focus on how to handle presets and default value settings for form data in PHP. Data preset Data preset refers to setting default values for input fields in the form when the form is loaded. In this way, when users fill out the form, they can see that some fields already have default values, which is convenient for users to operate. In PHP, you can use HTML
