How to use Java backend technology to implement message push function?
How to use Java backend technology to implement message push function?
With the development of mobile Internet, message push has become an important functional requirement in modern applications. In Java back-end development, we can use some mature technologies and frameworks to implement message push functions. This article will use WebSocket and Spring Boot as examples to introduce how to use Java back-end technology to implement the message push function.
1. Overview
WebSocket is a protocol for full-duplex communication on a single TCP connection. Unlike HTTP, HTTP is stateless, that is, the connection needs to be re-established with each request. The WebSocket protocol establishes a persistent connection between the client and the server, allowing the server to actively push messages to the client.
Spring Boot is a rapid development framework for Java back-end development, which provides rich functions and a good development experience. Combined with WebSocket, we can easily implement the message push function.
2. Use WebSocket to implement message push
- Configure WebSocket
First, we need to configure WebSocket support in Spring Boot. In the pom.xml file, add the following dependencies:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-websocket</artifactId> </dependency>
In the Spring Boot configuration class, enable WebSocket support:
@Configuration @EnableWebSocket public class WebSocketConfig implements WebSocketConfigurer { @Override public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) { registry.addHandler(new WebSocketHandler(), "/ws").setAllowedOrigins("*"); } }
- Implement WebSocketHandler
Next, we need to implement a WebSocketHandler to handle WebSocket messages and connections. Create a class, inherit from TextWebSocketHandler, and override several methods:
@Component public class WebSocketHandler extends TextWebSocketHandler { private List<WebSocketSession> sessions = new CopyOnWriteArrayList<>(); @Override public void afterConnectionEstablished(WebSocketSession session) { sessions.add(session); } @Override protected void handleTextMessage(WebSocketSession session, TextMessage message) throws Exception { for (WebSocketSession s : sessions) { s.sendMessage(message); } } @Override public void afterConnectionClosed(WebSocketSession session, CloseStatus status) { sessions.remove(session); } }
In the above code, we use CopyOnWriteArrayList to save all client connections. After the connection is established, add the WebSocketSession object to the sessions list. When a message arrives, traverse the sessions list and send the message to all clients. When the connection is closed, remove the WebSocketSession object from the sessions list.
- Front-end code
Finally, we need to add JavaScript code to the front-end page to establish a connection with WebSocket and process messages. An example is as follows:
var webSocket = new WebSocket("ws://localhost:8080/ws"); webSocket.onopen = function(event) { console.log("WebSocket连接已建立"); }; webSocket.onmessage = function(event) { console.log("收到消息:" + event.data); }; webSocket.onclose = function(event) { console.log("WebSocket连接已关闭"); };
In the above code, we create a WebSocket object and specify the address of the server. Through callback functions such as onopen, onmessage and onclose, we can handle events such as WebSocket connection establishment, message reception and connection closing.
4. Summary
Through the above steps, we successfully implemented the message push function using Java back-end technology. With the help of WebSocket and Spring Boot framework, we can easily implement real-time message push function. In actual applications, we can flexibly use the functions and features of WebSocket and Spring Boot according to needs to achieve more expansion and customization.
The above is the detailed content of How to use Java backend technology to implement message push function?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










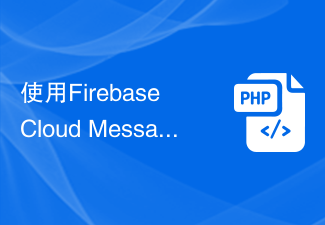
Use Firebase Cloud Messaging (FCM) to implement message push function in PHP applications. With the rapid development of mobile applications, real-time message push has become one of the indispensable functions of modern applications. Firebase Cloud Messaging (FCM) is a cross-platform messaging service that helps developers push real-time messages to Android and iOS devices. This article will introduce how to use FCM to implement message push function in PHP applications.
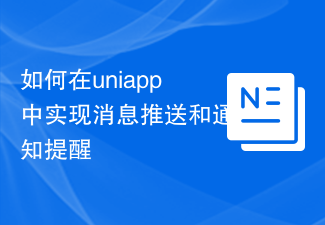
How to implement message push and notification reminders in uniapp With the rapid development of mobile Internet, message push and notification reminders have become indispensable functions in mobile applications. In uniapp, we can implement message push and notification reminders through some plug-ins and interfaces. This article will introduce a method to implement message push and notification reminder in uniapp, and provide specific code examples. 1. Message Push The premise for implementing message push is that we need a background service to send push messages. Here I recommend using Aurora Push.
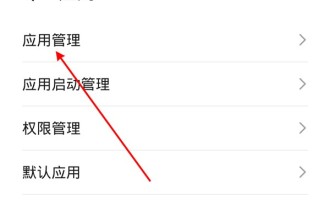
1. Open the phone settings, click Applications, and click Application Management. 2. Find and click to enter the Amap. 3. Click Notification Management and turn off the Allow Notifications switch to turn off message push notifications. This article takes Honor magic3 as an example and is applicable to Amap v11.10 version of MagicUI5.0 system.
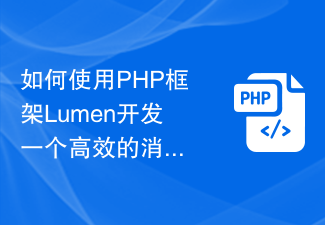
With the rapid development of mobile Internet and changes in user needs, the message push system has become an indispensable part of modern applications. It can realize instant notification, reminder, promotion, social networking and other functions to provide users and business customers with better services. experience and service. In order to meet this demand, this article will introduce how to use the PHP framework Lumen to develop an efficient message push system to provide timely push services. 1. Introduction to Lumen Lumen is a micro-framework developed by the Laravel framework development team. It is a
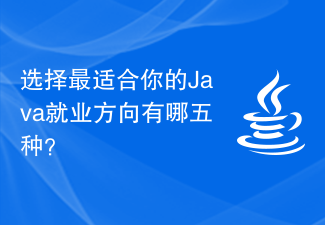
There are five employment directions in the Java industry, which one is suitable for you? Java, as a programming language widely used in the field of software development, has always been popular. Due to its strong cross-platform nature and rich development framework, Java developers have a wide range of employment opportunities in various industries. In the Java industry, there are five main employment directions, including JavaWeb development, mobile application development, big data development, embedded development and cloud computing development. Each direction has its characteristics and advantages. The five directions will be discussed below.
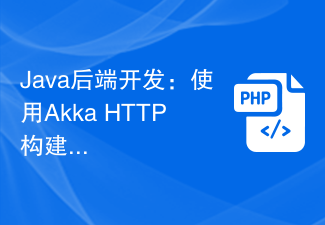
Reactive programming is becoming more and more important in today's web development. AkkaHTTP is a high-performance HTTP framework based on Akka, suitable for building reactive REST-style APIs. This article will introduce how to use AkkaHTTP to build a reactive API, while providing some practical examples. Let’s get started! Why choose AkkaHTTP When developing reactive APIs, it is important to choose the right framework. AkkaHTTP is a very good choice because
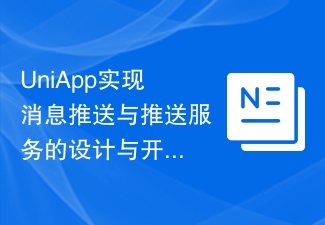
UniApp is a framework for developing cross-platform applications that can run on iOS, Android and Web platforms at the same time. When implementing the message push function, UniApp can cooperate with the back-end push service to realize the design and development of message push. 1. Design overview of message push To implement the message push function in UniApp, you need to design a push service to send push messages to the App. The push service needs to implement the following functions: establish a connection with the App and send messages.
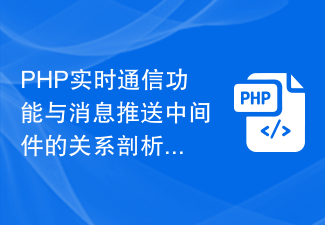
Analysis of the relationship between PHP real-time communication function and message push middleware With the development of the Internet, the importance of real-time communication function in Web applications has become increasingly prominent. Real-time communication allows users to send and receive messages in real-time in applications, and can be applied to a variety of scenarios, such as real-time chat, instant notification, etc. In the field of PHP, there are many ways to implement real-time communication functions, and one of the common ways is to use message push middleware. This article will introduce the relationship between PHP real-time communication function and message push middleware, and how to use message push
