


How to use JIT compilation to optimize the execution speed of Python programs
How to use JIT compilation to optimize the execution speed of Python programs
1. Introduction
In Python programming, due to its interpretation and execution characteristics, the execution speed is often slow. In order to improve the performance of Python programs, a common method is to use just-in-time (JIT) technology. JIT can compile Python code into local machine code to accelerate code execution.
2. JIT compiler
The JIT compiler is a dynamic compiler that compiles source code into machine code when the program is running. In Python, there are several JIT compilers to choose from, such as PyPy, Numba, and Cython. These tools can optimize based on the characteristics of the code and convert it into more efficient machine code.
3. Use PyPy to accelerate Python programs
PyPy is a Python interpreter that uses JIT compilation technology. Relative to the standard CPython interpreter, PyPy has higher execution speed. The following is an example of using PyPy to accelerate Python programs:
# 使用PyPy解释器执行Python代码 def factorial(n): if n == 0 or n == 1: return 1 return n * factorial(n-1) if __name__ == "__main__": import time start_time = time.time() result = factorial(1000) end_time = time.time() print("Result: ", result) print("Execution time: ", end_time - start_time)
4. Using Numba to accelerate Python programs
Numba is a JIT compiler based on LLVM, which can compile Python code into efficient machine code . The following is an example of using Numba to accelerate Python programs:
# 使用Numba加速Python代码 from numba import jit @jit def factorial(n): if n == 0 or n == 1: return 1 return n * factorial(n-1) if __name__ == "__main__": import time start_time = time.time() result = factorial(1000) end_time = time.time() print("Result: ", result) print("Execution time: ", end_time - start_time)
5. Use Cython to accelerate Python programs
Cython is a tool that converts Python code into C code. Cython can be used to execute Python programs. Significant speed improvements. The following is an example of using Cython to accelerate a Python program:
# 使用Cython加速Python代码 import cython @cython.ccall def factorial(n): if n == 0 or n == 1: return 1 return n * factorial(n-1) if __name__ == "__main__": import time start_time = time.time() result = factorial(1000) end_time = time.time() print("Result: ", result) print("Execution time: ", end_time - start_time)
6. Summary
By using the JIT compiler, we can greatly improve the execution speed of Python programs. This article introduces three commonly used JIT compilers: PyPy, Numba and Cython, and gives corresponding code examples. These tools can be selected on a case-by-case basis to achieve efficient optimization of Python code.
The above is the detailed content of How to use JIT compilation to optimize the execution speed of Python programs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










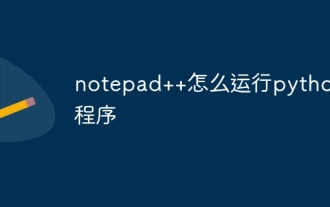
Using Notepad++ to run a Python program requires the following steps: 1. Install the Python plug-in; 2. Create a Python file; 3. Set the run options; 4. Run the program.
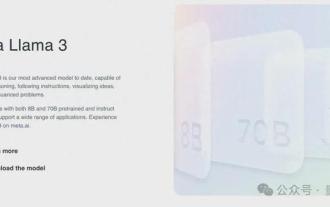
Llama3 is here! Just now, Meta’s official website was updated and the official announced Llama 38 billion and 70 billion parameter versions. And it is an open source SOTA after its launch: Meta official data shows that the Llama38B and 70B versions surpass all opponents in their respective parameter scales. The 8B model outperforms Gemma7B and Mistral7BInstruct on many benchmarks such as MMLU, GPQA, and HumanEval. The 70B model has surpassed the popular closed-source fried chicken Claude3Sonnet, and has gone back and forth with Google's GeminiPro1.5. As soon as the Huggingface link came out, the open source community became excited again. The sharp-eyed blind students also discovered immediately
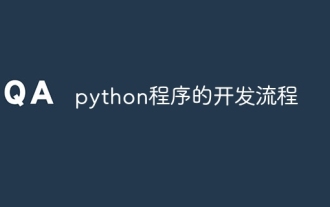
The Python program development process includes the following steps: Requirements analysis: clarify business needs and project goals. Design: Determine architecture and data structures, draw flowcharts or use design patterns. Writing code: Program in Python, following coding conventions and documentation comments. Testing: Writing unit and integration tests, conducting manual testing. Review and Refactor: Review code to find flaws and improve readability. Deploy: Deploy the code to the target environment. Maintenance: Fix bugs, improve functionality, and monitor updates.
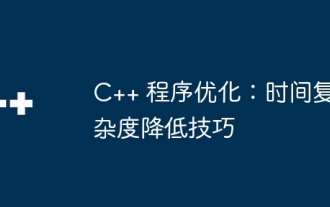
Time complexity measures the execution time of an algorithm relative to the size of the input. Tips for reducing the time complexity of C++ programs include: choosing appropriate containers (such as vector, list) to optimize data storage and management. Utilize efficient algorithms such as quick sort to reduce computation time. Eliminate multiple operations to reduce double counting. Use conditional branches to avoid unnecessary calculations. Optimize linear search by using faster algorithms such as binary search.
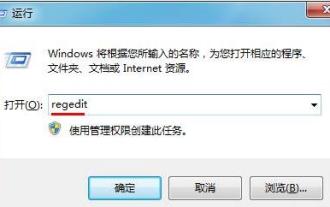
1. Press the key combination (win key + R) on the desktop to open the run window, then enter [regedit] and press Enter to confirm. 2. After opening the Registry Editor, we click to expand [HKEY_CURRENT_USERSoftwareMicrosoftWindowsCurrentVersionExplorer], and then see if there is a Serialize item in the directory. If not, we can right-click Explorer, create a new item, and name it Serialize. 3. Then click Serialize, then right-click the blank space in the right pane, create a new DWORD (32) bit value, and name it Star
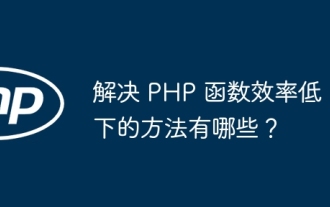
Five ways to optimize PHP function efficiency: avoid unnecessary copying of variables. Use references to avoid variable copying. Avoid repeated function calls. Inline simple functions. Optimizing loops using arrays.
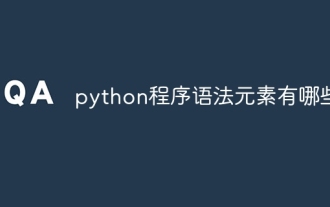
Python program syntax elements include: 1. Keywords are used to define program structure and functions; 2. Identifiers are used to name variables and objects; 3. Operators are used to perform arithmetic, logical and comparison operations; 4. Data types include integers, Floating point numbers, Boolean values, etc.; 5. Statements are used to perform operations; 6. Expressions are evaluations of values; 7. Comments are used to provide code information.
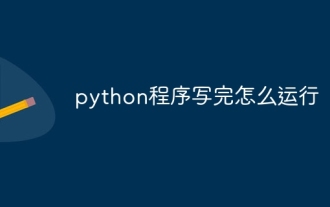
There are two ways to run a program in Python: 1. Using the Python interpreter; 2. Calling a script directly. Specific steps include: ① Create a Python script file; ② Open a terminal or command prompt; ③ Navigate to the script directory; ④ Use the corresponding method to run the script; ⑤ View the output.
