


Application of Redis in JavaScript development: How to cache and speed up web page loading
Application of Redis in JavaScript development: How to cache and speed up web page loading
Introduction:
With the popularity of the Internet, the loading speed of web pages is becoming more and more important. In JavaScript development, we often encounter situations where we need to load a large amount of data, which not only increases the user's waiting time, but also consumes server resources. In order to solve this problem, we can use Redis to cache data and speed up the loading of web pages. This article will introduce the application of Redis in JavaScript development and how to use Redis to cache and speed up web page loading.
1. Introduction to Redis
Redis (Remote Dictionary Server) is an open source in-memory data storage system that can be used as a database, cache and message middleware. It supports a variety of data structures, such as strings, hash tables, lists, sets, etc., and has persistence, replication, transactions and other functions. Since Redis stores data in memory, it has very fast read and write speeds and is suitable for handling a large number of read and write operations.
2. Installation and use of Redis
- Installation of Redis
The installation of Redis is very simple. You can download and install it from the Redis official website (https://redis.io/) . After the installation is complete, run the Redis server. -
Connecting to Redis
In JavaScript development, you can use the node_redis library to connect to the Redis server. First you need to install the node_redis library:npm install redis
Copy after loginThen you can use the following code in the code to connect to the Redis server:
var redis = require("redis"); var client = redis.createClient();
Copy after login Store data to Redis
When loading a web page, We can store the data that needs to be loaded into Redis for next time use. For example, we have user information that needs to be read from the database. This information can be stored in Redis:var user = { id: 1, name: "John", age: 25 }; client.set("user:1", JSON.stringify(user));
Copy after loginReading data from Redis
Load the web page next time When, we can first read the data from Redis, use it directly if it exists, and read it from the database if it does not exist:client.get("user:1", function(err, reply) { if (reply) { var user = JSON.parse(reply); // 使用用户信息 } else { // 从数据库中读取用户信息 } });
Copy after loginSet the cache expiration time
In order to prevent After the cached data expires, the old data is still used. We can set an expiration time for the cache. For example, we can set the cache of user information for one day:client.setex("user:1", 86400, JSON.stringify(user));
Copy after loginCache data update
When the data changes, the cached data needs to be updated. For example, when user information changes, we need to update the user information in the cache:client.set("user:1", JSON.stringify(updatedUser));
Copy after login
3. Use Redis to cache and accelerate web page loading
In actual web development, We can use Redis to cache some reused data to speed up the loading of web pages. The following is an example to illustrate how to use Redis to cache and speed up web page loading.
Example: Website article list
Suppose we develop a blog website and need to display a list of the latest published articles. Every time a web page is loaded, we can get the latest list of articles from the database and store it in Redis. The next time we load a web page, we can first try to read the article list from Redis. If it exists, use it directly. If it does not exist, get it from the database and store it in Redis. Here is the sample code:
// 从Redis中读取文章列表 client.get("articles:list", function(err, reply) { if (reply) { var articles = JSON.parse(reply); // 使用文章列表 renderArticles(articles); } else { // 从数据库中获取文章列表 getArticles(function(articles) { // 存储文章列表到Redis中,并设置过期时间为一小时 client.setex("articles:list", 3600, JSON.stringify(articles)); // 使用文章列表 renderArticles(articles); }); } }); // 从数据库中获取文章列表的函数 function getArticles(callback) { // 从数据库中获取文章列表 // ... var articles = [/* 最新的文章列表 */]; callback(articles); } // 渲染文章列表的函数 function renderArticles(articles) { // 渲染文章列表 }
In the above example, we first try to read the list of articles from Redis. If the Redis cache exists, the cached data is used directly without obtaining it from the database; if the Redis cache does not exist, the latest article list is obtained from the database, stored in Redis, and the expiration time is set. In this way, when the web page is loaded again within an hour, the article list cached by Redis can be used directly, eliminating the time of obtaining data from the database.
Conclusion:
By using Redis to cache data, we can speed up the loading speed of web pages and improve user experience. In JavaScript development, we can use the node_redis library to connect to the Redis server, use the set and get methods to store and read data, and use the setex method to set the expiration time of cached data. By rationally using Redis cache, you can effectively reduce the load on the server and improve the performance of web pages.
Through the above examples, we learned about the application of Redis in JavaScript development and how to use Redis to cache and accelerate web page loading. I hope this article will help you understand and apply Redis in JavaScript development!
The above is the detailed content of Application of Redis in JavaScript development: How to cache and speed up web page loading. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










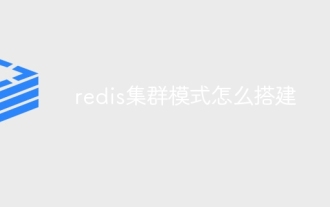
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
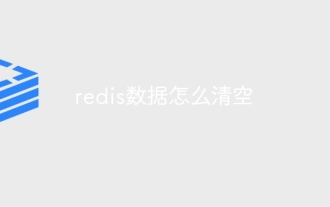
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
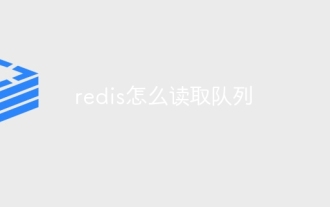
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
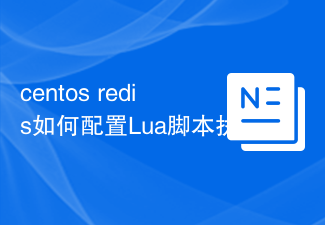
On CentOS systems, you can limit the execution time of Lua scripts by modifying Redis configuration files or using Redis commands to prevent malicious scripts from consuming too much resources. Method 1: Modify the Redis configuration file and locate the Redis configuration file: The Redis configuration file is usually located in /etc/redis/redis.conf. Edit configuration file: Open the configuration file using a text editor (such as vi or nano): sudovi/etc/redis/redis.conf Set the Lua script execution time limit: Add or modify the following lines in the configuration file to set the maximum execution time of the Lua script (unit: milliseconds)
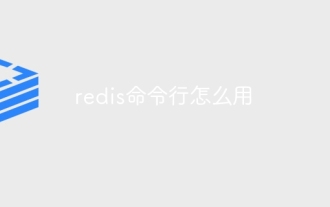
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
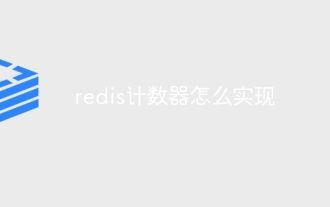
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.
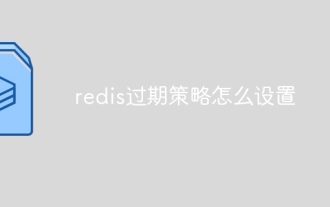
There are two types of Redis data expiration strategies: periodic deletion: periodic scan to delete the expired key, which can be set through expired-time-cap-remove-count and expired-time-cap-remove-delay parameters. Lazy Deletion: Check for deletion expired keys only when keys are read or written. They can be set through lazyfree-lazy-eviction, lazyfree-lazy-expire, lazyfree-lazy-user-del parameters.
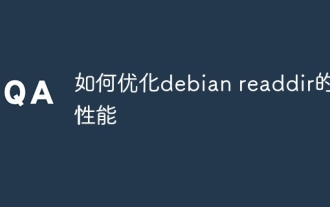
In Debian systems, readdir system calls are used to read directory contents. If its performance is not good, try the following optimization strategy: Simplify the number of directory files: Split large directories into multiple small directories as much as possible, reducing the number of items processed per readdir call. Enable directory content caching: build a cache mechanism, update the cache regularly or when directory content changes, and reduce frequent calls to readdir. Memory caches (such as Memcached or Redis) or local caches (such as files or databases) can be considered. Adopt efficient data structure: If you implement directory traversal by yourself, select more efficient data structures (such as hash tables instead of linear search) to store and access directory information
