


How to use Java programming to implement weather forecast query of Amap Map API
How to use Java programming to implement weather forecast query of Amap Map API
Introduction:
Amap is a well-known map service provider in China. Its API contains a wealth of functions, among which One is weather forecast query. This article will introduce how to use Java programming to implement weather forecast query of Amap API, and give corresponding code examples.
1. Register on the Amap Open Platform and obtain the API Key
First, we need to register on the Amap Open Platform (https://lbs.amap.com/) and create an application to obtain API Key. The specific steps are as follows:
- Open the Amap Open Platform website and click the "Register" button in the upper right corner.
- Fill in the registration information according to the website guidelines and complete the registration.
- Log in to the AutoNavi open platform, click the "Account" button in the upper right corner, and select "Create Application".
- Fill in the basic information of the application and click the "Create Application" button.
- After successful creation, enter the application management page, find the "API Key" in the "Developer Key" column, copy and save it.
2. Introducing the Java SDK of the Amap API
Before using Java to implement the weather forecast query of the Amap API, we need to introduce the corresponding Java SDK. The specific steps are as follows:
- Open the configuration file (pom.xml or build.gradle) of the Java build tool (such as Maven or Gradle).
- Add the Java SDK dependency statement of the Amap API in the configuration file. The specific dependency version can be flexibly selected according to the official documentation.
Maven configuration example:
<dependencies> <dependency> <groupId>com.amap.api</groupId> <artifactId>amap-java-sdk</artifactId> <version>2.9.0</version> </dependency> </dependencies>
Gradle configuration example:
dependencies { implementation 'com.amap.api:amap-java-sdk:2.9.0' }
3. Write Java code to implement weather forecast query
Next, we start writing Java Code to implement weather forecast query. The specific steps are as follows:
- Create a Java class, such as WeatherForecastQuery.
- Add a main method to the class as the entry point of the program.
The sample code is as follows:
import com.amap.api.weather.WeatherSearch; import com.amap.api.weather.model.WeatherSearchQuery; public class WeatherForecastQuery { public static void main(String[] args) { // 替换为你自己的API Key String apiKey = "Your API Key"; // 创建天气查询的请求对象 WeatherSearchQuery query = new WeatherSearchQuery("北京市", WeatherSearchQuery.WEATHER_TYPE_FORECAST); // 创建天气查询的对象 WeatherSearch search = new WeatherSearch(apiKey); // 发起天气查询 search.searchWeatherAsyn(query, new WeatherSearch.OnWeatherSearchListener() { @Override public void onWeatherLiveSearched(com.amap.api.weather.model.LocalWeatherLiveResult localWeatherLiveResult, int i) { // 处理实时天气查询结果 } @Override public void onWeatherForecastSearched(com.amap.api.weather.model.LocalWeatherForecastResult localWeatherForecastResult, int i) { // 处理天气预报查询结果 if (i == 1000) { // 查询成功 com.amap.api.weather.model.LocalWeatherForecast forecast = localWeatherForecastResult.getForecastResult(); // 处理天气预报数据 System.out.println(forecast.getReportTime()); for (com.amap.api.weather.model.WeatherForecast forecastItem : forecast.getWeatherForecast()) { System.out.println(forecastItem.getDate()); System.out.println(forecastItem.getDayWeather()); System.out.println(forecastItem.getNightWeather()); // 其他相关天气信息... } } else { // 查询失败 System.out.println("查询失败,错误码:" + i); } } }); } }
4. Run the code and get the weather forecast results
Replace "Your API Key" in the code with your own API Key, and Run the code to get the weather forecast results. Here we take querying the weather forecast of Beijing as an example.
After the code is executed, if the query is successful, the queried weather forecast data will be printed out, including forecast time, date, daytime weather, nighttime weather and other information.
Summary:
This article introduces the steps of how to use Java programming to implement the weather forecast query of the Amap API, from registering the Amap open platform and obtaining the API Key, to introducing the Java SDK of the Amap API , and then write Java code to implement weather forecast query, and provide corresponding code examples. Through these steps, we can easily use the Amap API to obtain weather forecast data, and process and display it in our own application.
The above is the detailed content of How to use Java programming to implement weather forecast query of Amap Map API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










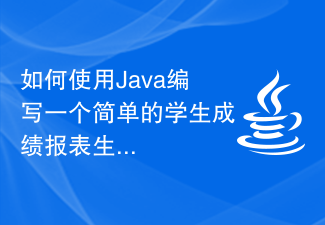
How to write a simple student performance report generator using Java? Student Performance Report Generator is a tool that helps teachers or educators quickly generate student performance reports. This article will introduce how to use Java to write a simple student performance report generator. First, we need to define the student object and student grade object. The student object contains basic information such as the student's name and student number, while the student score object contains information such as the student's subject scores and average grade. The following is the definition of a simple student object: public
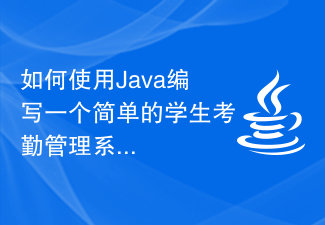
How to write a simple student attendance management system using Java? With the continuous development of technology, school management systems are also constantly updated and upgraded. The student attendance management system is an important part of it. It can help the school track students' attendance and provide data analysis and reports. This article will introduce how to write a simple student attendance management system using Java. 1. Requirements Analysis Before starting to write, we need to determine the functions and requirements of the system. Basic functions include registration and management of student information, recording of student attendance data and
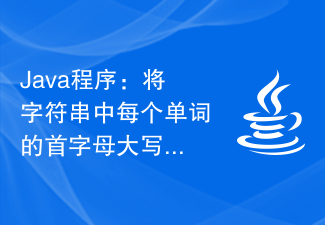
Astringisaclassof'java.lang'packagethatstoresaseriesofcharacters.ThosecharactersareactuallyString-typeobjects.Wemustenclosethevalueofstringwithindoublequotes.Generally,wecanrepresentcharactersinlowercaseanduppercaseinJava.And,itisalsopossibletoconver
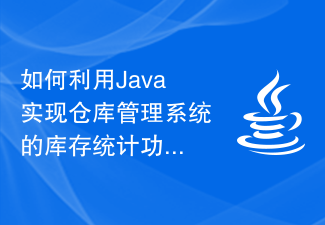
How to use Java to implement the inventory statistics function of the warehouse management system. With the development of e-commerce and the increasing importance of warehousing management, the inventory statistics function has become an indispensable part of the warehouse management system. Warehouse management systems written in the Java language can implement inventory statistics functions through concise and efficient code, helping companies better manage warehouse storage and improve operational efficiency. 1. Background introduction Warehouse management system refers to a management method that uses computer technology to perform data management, information processing and decision-making analysis on an enterprise's warehouse. Inventory statistics are
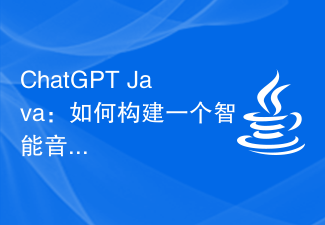
ChatGPTJava: How to build an intelligent music recommendation system, specific code examples are needed. Introduction: With the rapid development of the Internet, music has become an indispensable part of people's daily lives. As music platforms continue to emerge, users often face a common problem: how to find music that suits their tastes? In order to solve this problem, the intelligent music recommendation system came into being. This article will introduce how to use ChatGPTJava to build an intelligent music recommendation system and provide specific code examples. No.
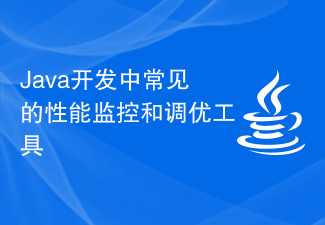
Common performance monitoring and tuning tools in Java development require specific code examples Introduction: With the continuous development of Internet technology, Java, as a stable and efficient programming language, is widely used in the development process. However, due to the cross-platform nature of Java and the complexity of the running environment, performance issues have become a factor that cannot be ignored in development. In order to ensure high availability and fast response of Java applications, developers need to monitor and tune performance. This article will introduce some common Java performance monitoring and tuning
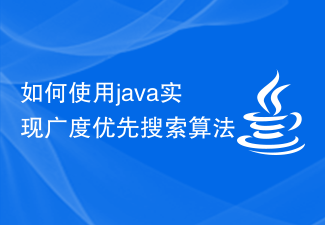
How to use Java to implement breadth-first search algorithm Breadth-First Search algorithm (Breadth-FirstSearch, BFS) is a commonly used search algorithm in graph theory, which can find the shortest path between two nodes in the graph. BFS is widely used in many applications, such as finding the shortest path in a maze, web crawlers, etc. This article will introduce how to use Java language to implement the BFS algorithm, and attach specific code examples. First, we need to define a class for storing graph nodes. This class contains nodes
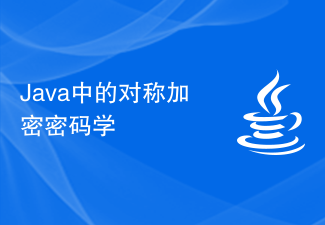
IntroductionSymmetric encryption, also known as key encryption, is an encryption method in which the same key is used for encryption and decryption. This encryption method is fast and efficient and suitable for encrypting large amounts of data. The most commonly used symmetric encryption algorithm is Advanced Encryption Standard (AES). Java provides strong support for symmetric encryption, including classes in the javax.crypto package, such as SecretKey, Cipher, and KeyGenerator. Symmetric encryption in Java The JavaCipher class in the javax.crypto package provides cryptographic functions for encryption and decryption. It forms the core of the Java Cryptozoology Extensions (JCE) framework. In Java, the Cipher class provides symmetric encryption functions, and K
