


Interpretation of Amap API documentation: Java code implements positioning function
Interpretation of Amap API documentation: Java code to implement positioning function
Abstract: With the popularity of mobile applications, positioning function has become one of the core requirements of many applications. In this article, we will use Java code to implement a simple positioning function by interpreting the documentation of the Amap API.
1. Introduction
Amap API provides a variety of functional interfaces, including positioning services. We can use the Amap SDK to obtain the location information of the device and perform corresponding processing in the application. This article will use Java code as an example to introduce how to use the Amap API to implement positioning functions.
2. Obtain the Amap developer account and key
- Visit the Amap open platform website: https://lbs.amap.com/dev/
- Log in or register an AutoNavi open platform account and create a new application.
- In the application management page, find the application's Key, which will be used as the authentication credential for API requests. Copy this Key, we will use it in the code.
3. Import the Amap SDK
When using the Amap API in a Java project, you need to import the corresponding SDK. We can download the latest version of the SDK from the AMAP open platform.
- Download address: https://lbs.amap.com/api/android-sdk/guide/create-project/manual-import/
- Unzip the downloaded SDK file , and import the jar package into the project's dependencies.
4. Write positioning code
The following is a simple Java code example to implement the positioning function:
import com.amap.api.location.AMapLocation; import com.amap.api.location.AMapLocationClient; import com.amap.api.location.AMapLocationClientOption; import com.amap.api.location.AMapLocationListener; public class LocationService { // 定位回调监听器 private AMapLocationListener mLocationListener; // 定位客户端 private AMapLocationClient mLocationClient; // 初始化定位 public void initLocation(Context context) { // 创建定位客户端 mLocationClient = new AMapLocationClient(context); // 设置定位参数 AMapLocationClientOption clientOption = new AMapLocationClientOption(); clientOption.setLocationMode(AMapLocationClientOption.AMapLocationMode.Hight_Accuracy); clientOption.setNeedAddress(true); clientOption.setWifiScan(true); clientOption.setMockEnable(false); clientOption.setInterval(2000); mLocationClient.setLocationOption(clientOption); // 设置定位回调监听器 mLocationListener = new AMapLocationListener() { @Override public void onLocationChanged(AMapLocation aMapLocation) { if (aMapLocation != null) { // 处理定位结果 double latitude = aMapLocation.getLatitude(); double longitude = aMapLocation.getLongitude(); String address = aMapLocation.getAddress(); // TODO: 定位成功后的操作 } else { // 定位失败处理 } } }; // 设置定位回调监听 mLocationClient.setLocationListener(mLocationListener); } // 开始定位 public void startLocation() { if (mLocationClient != null) { // 启动定位 mLocationClient.startLocation(); } } // 停止定位 public void stopLocation() { if (mLocationClient != null) { // 停止定位 mLocationClient.stopLocation(); } } }
5. Use positioning service
To use this location service in your Java project, you can follow the following steps:
- Create an instance of LocationService and call the initLocation() method to initialize it.
- Where positioning is required, call the startLocation() method to start positioning.
- In places where positioning is not required, call the stopLocation() method to stop positioning.
6. Summary
Through the above code examples, we can understand how to use the Amap API to implement the positioning function. Of course, the Amap API also provides more functions, such as map display, route planning, etc. I hope this article can help you use the Amap API to implement positioning functions.
The above is the detailed content of Interpretation of Amap API documentation: Java code implements positioning function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
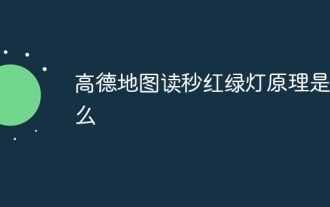
The principle of AutoNavi Map's countdown traffic lights is: 1. Realized through the real-time traffic data of AutoNavi Map; 2. Using its powerful algorithm and artificial intelligence technology to process and analyze the collected traffic data; 3. Based on the traffic of vehicles at the intersection Traffic flow, traffic congestion and other factors are predicted and calculated to provide more accurate remaining traffic light time; 4. Calculate the user's route based on the user's location and destination information.
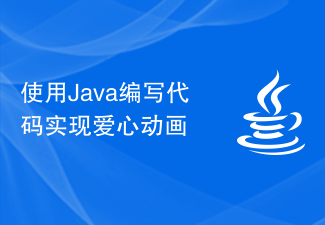
Realizing love animation effects through Java code In the field of programming, animation effects are very common and popular. Various animation effects can be achieved through Java code, one of which is the heart animation effect. This article will introduce how to use Java code to achieve this effect and give specific code examples. The key to realizing the heart animation effect is to draw the heart-shaped pattern and achieve the animation effect by changing the position and color of the heart shape. Here is the code for a simple example: importjavax.swing.
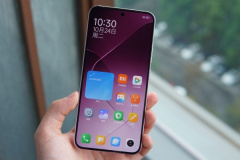
For mobile phones, the positioning function is very important. Most software now requires the positioning function to be turned on for normal use. If the positioning function of the mobile phone is not very accurate, it may cause bad consequences. As Xiaomi’s latest flagship product, Xiaomi Mi 14 naturally has the strongest positioning system. So how to enable the positioning function on Xiaomi Mi 14? How to enable positioning function on Xiaomi Mi 14? How to enable the positioning function on Xiaomi Mi 14 is as follows: Step 1: Open the settings application of your phone. Step 2: Find and click on the "Location" option. Step 3: In the location setting interface, confirm the switch to enable the positioning function. Sometimes the positioning function may be disabled, so make sure the switch is turned on. Step 4: You can further customize the accuracy and accuracy of the positioning function
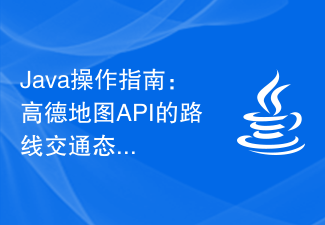
Java Operation Guide: Detailed explanation of the route traffic situation query function of Amap API Introduction: With the continuous development of urban transportation, our demand for real-time traffic conditions is becoming more and more important. As an excellent geographical information service platform, Amap provides a rich map API interface, including route and traffic situation query functions. This article will introduce in detail how to use Java language to operate the Amap API, and combine it with code examples to demonstrate the specific implementation of route traffic situation query. 1. Register and obtain the Key of AMAP API at
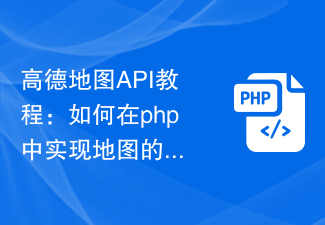
Amap API Tutorial: How to implement map path planning and navigation in PHP Map path planning and navigation is an important function in travel and navigation applications. In this tutorial, we will introduce how to use the Amap API to implement map route planning navigation in PHP. We will use the route planning interface provided by the Amap API to obtain the route planning results through HTTP requests and display them on the front-end map. Next, we will introduce it in detail step by step. Register an AutoNavi developer account and create an application. First, I
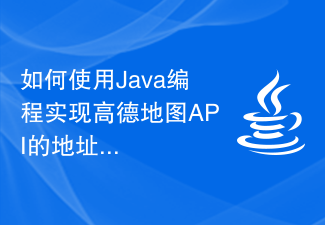
How to use Java programming to implement the address location search of the Amap API Introduction: Amap is a very popular map service and is widely used in various applications. Among them, the search function near the address location provides the ability to search for nearby POI (Point of Interest, points of interest). This article will explain in detail how to use Java programming to implement the address location search function of the Amap API, and use code examples to help readers understand and master related technologies. 1. Apply for Amap development
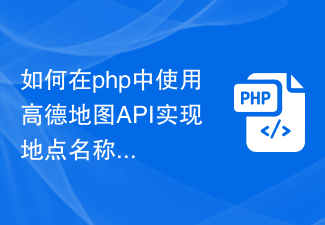
Overview of how to use the Amap API in PHP to implement fuzzy search of place names: When developing an application based on geographical location, sometimes it is necessary to perform a fuzzy search based on the place name entered by the user and return the search results. Amap provides a rich set of APIs that can easily implement this function. This article will introduce how to use the Amap API in PHP to implement fuzzy search of place names and provide you with code examples. Steps: Obtain the developer key of the Amap API. First, you need to open the Amap open platform
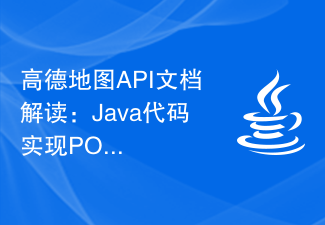
Interpretation of Amap API documentation: Java code implements POI search function With the development of the mobile Internet, map applications have become an indispensable part of our daily lives. As the leading map application provider in China, Amap's API documentation has become a must-have reference for many developers. This article will interpret the Amap API documentation and give Java code examples to implement the POI (Point of Interest) search function. First, we need to apply for API on the Amap open platform
