Build high-performance image processing applications using Redis and C++
Use Redis and C to build high-performance image processing applications
Image processing is one of the important links in modern computer applications. Due to the complexity and large amount of calculations in image processing, how to provide stable services while ensuring high performance is a challenge. This article will introduce how to use Redis and C to build high-performance image processing applications, and provide some code examples.
Redis is an open source in-memory database with high performance and high availability. It supports various data structures, such as strings, hash tables, lists, etc., and can persist data to disk. In image processing applications, we can store image data in Redis and process the images through applications written in C.
First, we need to install Redis and start the Redis service. In the Ubuntu system, you can use the following command to install Redis:
$ sudo apt-get update $ sudo apt-get install redis-server
After the installation is complete, you can use the following command to start the Redis service:
$ redis-server
Next, we need to use C to write an image processing app. Below is a simple example of a program that uses the OpenCV library to read an image file and store the image data in Redis:
#include <iostream> #include <opencv2/opencv.hpp> #include <redisclient/redissyncclient.h> int main() { // 连接Redis RedisClient::SslOptions sslOption; RedisClient::Client redis("localhost", 6379, sslOption); // 读取图像文件 cv::Mat image = cv::imread("image.jpg", cv::IMREAD_UNCHANGED); // 将图像数据转换为字符串 std::vector<uchar> imageBuf; cv::imencode(".jpg", image, imageBuf); std::string imageStr(imageBuf.begin(), imageBuf.end()); // 存储图像数据到Redis redis.command("SET", "image", imageStr); // 从Redis获取图像数据 std::string result = redis.commandSync<std::string>("GET", "image"); // 将字符串转换为图像数据 cv::Mat resultImage = cv::imdecode(cv::Mat(result.size(), 1, CV_8UC1, (void*)result.c_str()), cv::IMREAD_UNCHANGED); // 显示图像 cv::imshow("result", resultImage); cv::waitKey(0); return 0; }
In the above example, we first connect to the Redis server. Then, use the OpenCV library to read the image file and convert the image data into a string. Next, we store the image data into Redis and obtain the image data through the Redis GET command. Finally, we convert the acquired image data into an OpenCV Mat object and display it in the window.
The above example is just a simple demonstration, and actual image processing applications may be more complex. More Redis commands and image processing algorithms can be used according to specific needs. In addition, in order to improve performance, you can use the pipeline function of Redis to execute multiple Redis commands at once.
Summary:
This article introduces how to use Redis and C to build high-performance image processing applications, and provides a simple code example. Use Redis to effectively manage image data and provide stable and high-performance services. In practical applications, it can be further optimized and expanded according to needs. I hope this article will be helpful to readers in building image processing applications.
The above is the detailed content of Build high-performance image processing applications using Redis and C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










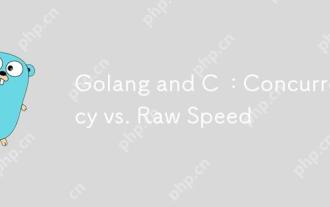
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
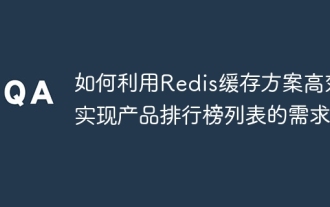
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
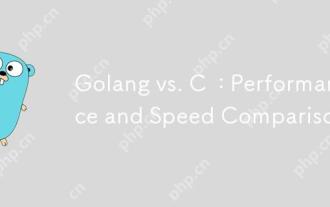
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
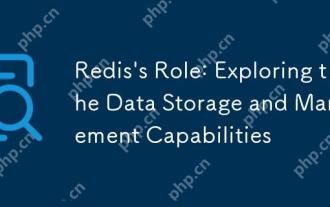
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
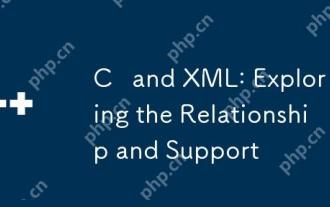
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
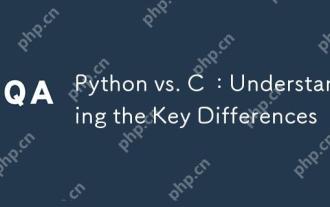
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
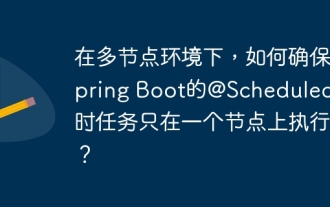
The optimization solution for SpringBoot timing tasks in a multi-node environment is developing Spring...
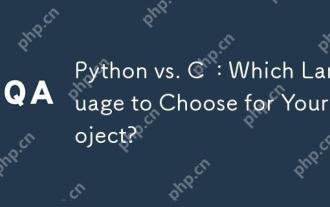
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
