


CakePHP middleware: the ability to integrate third-party APIs and services
CakePHP middleware: The function of integrating third-party APIs and services
Overview:
In modern Web development, integrating third-party APIs and services has become a common requirement. CakePHP middleware provides a concise and elegant way to handle these requirements. This article will introduce how to use middleware in CakePHP to integrate the functions of third-party APIs and services, and provide some code examples.
What is middleware?
Middleware is a series of operations performed during the request and response process. It can perform certain actions before the request reaches the controller and perform other actions before the response is returned. In CakePHP, middleware handles requests and responses by adding various functions to the request process.
How to use middleware to integrate the functions of third-party APIs and services?
It is very simple to use middleware to integrate third-party APIs and services. The following are a basic steps:
- Create a middleware class:
First, we need to create a middleware class, which will be responsible for handling the logic of interacting with third-party APIs and services. For example, we can create a middleware class called ApiMiddleware.
// src/Middleware/ApiMiddleware.php namespace AppMiddleware; use CakeHttpResponse; use CakeHttpServerRequest; use GuzzleHttpClient; class ApiMiddleware { public function __invoke(ServerRequest $request, Response $response, $next) { // 处理与API的交互逻辑 // 发送请求到API $client = new Client(); $apiResponse = $client->get('https://api.example.com/data'); // 获取API响应数据 $data = json_decode($apiResponse->getBody(), true); // 将API响应数据设置到请求对象中,以便在控制器中使用 $request = $request->withAttribute('apiData', $data); // 继续传递请求和响应到下一个中间件或控制器 $response = $next($request, $response); return $response; } }
In the above code, we use the Guzzle HTTP client to send a request to an imaginary API and set the API response data into the request object.
- Configuring middleware:
Next, we need to configure the middleware. Add the following code to theconfig/middleware.php
file:
// config/middleware.php $middlewareQueue ->add(new AppMiddlewareApiMiddleware());
This will add ApiMiddleware to the middleware queue so that it can be executed during the request process.
- Using middleware data in the controller:
Now, we can access the middleware data in the controller through the request object. The following is the code of a sample controller:
// src/Controller/ExampleController.php namespace AppController; use CakeHttpExceptionNotFoundException; use CakeHttpResponse; use CakeORMTableRegistry; class ExampleController extends AppController { public function index() { // 获取中间件中设置的API数据 $apiData = $this->request->getAttribute('apiData'); // 使用API数据执行其他操作 // ... // 返回响应 $this->set([ 'apiData' => $apiData, '_serialize' => ['apiData'] ]); } }
In the above code, we obtain the API data set by the middleware through the getAttribute()
method of the request object, and Pass it to the view for display.
To enable the controller to serialize and return API data, we use the _serialize
option. This enables the response object to automatically serialize the data and convert it back into JSON format.
Summary:
By using CakePHP middleware, we can easily integrate the functions of third-party APIs and services. This article provides a basic example showing how to create and configure a middleware and use the middleware's data in a controller. I hope this article will help you integrate third-party APIs and services in CakePHP.
The above is the detailed content of CakePHP middleware: the ability to integrate third-party APIs and services. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










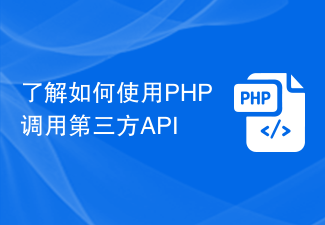
In recent years, more and more applications need to call third-party API interfaces. And one of the very popular languages is PHP. In this article, we will explore how to call third-party APIs using PHP. First, let's define what an API is. API stands for Application Programming Interface, which are rules that allow applications to communicate with each other. Specifically, an API is a set of predefined functions or methods that allow developers to access the services of other applications or platforms through a simple request/response model. Common
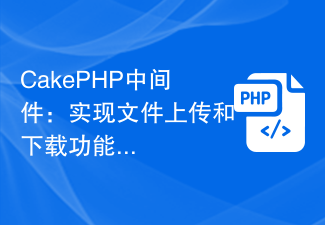
CakePHP middleware: realizing file upload and download functions. With the development of the Internet, file upload and download functions are becoming more and more common. When developing web applications, we often need to implement file upload and download. When developing applications using the CakePHP framework, middleware is a very useful tool that can help us simplify the code and implement file upload and download functions. Next, I will introduce how to use CakePHP middleware to implement file upload and download functions. First, we need to create a new
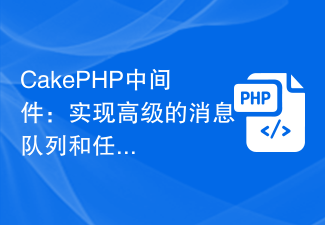
CakePHP Middleware: Implementing Advanced Message Queuing and Task Scheduling With the rapid development of the Internet, we are faced with the challenge of handling a large number of concurrent requests and task scheduling. The traditional request response model can no longer meet our needs. In order to better solve this problem, CakePHP introduces the concept of middleware and provides rich functions to implement advanced message queue and task scheduling. Middleware is one of the core components of CakePHP applications and can add custom logic to the request processing flow. through middleware
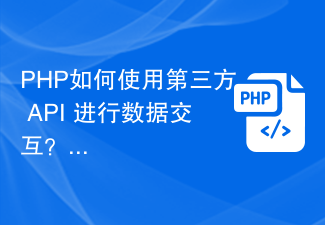
How does PHP use third-party APIs for data interaction? With the development of the Internet, many websites and applications need to interact with third-party APIs to obtain and process external data. As a popular server-side scripting language, PHP has powerful capabilities to handle these data interactions. This article will introduce how PHP uses third-party APIs for data interaction. Third-party API (ApplicationProgrammingInterface) refers to other groups
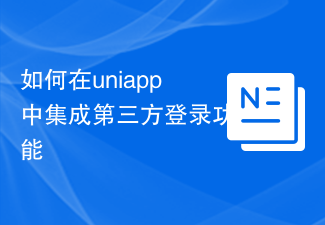
How to integrate third-party login function in uniapp In today's social media era, third-party login function has become an indispensable part of many applications. By integrating third-party login functions, users can use accounts on other platforms to quickly log in and use applications. This article will take uniapp as an example to introduce how to integrate the third-party login function in uniapp and provide specific code examples. To create a third-party open platform application, first, you need to register on the corresponding third-party open platform and create a
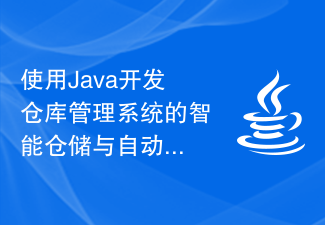
Title: Using Java to develop intelligent warehousing and automated storage equipment integration functions of warehouse management system Abstract: With the development of the logistics industry, warehouse management systems play an important role in improving warehousing efficiency and reducing costs. In order to meet the growing logistics needs, developing a warehouse management system with the help of Java and integrating the functions of intelligent warehousing and automated storage equipment has become a solution worth considering. This article will explore how to use Java to develop a warehouse management system and give relevant code examples. Introducing warehouse management systems that take full advantage of computing
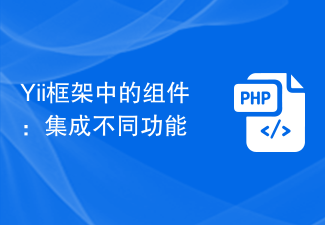
As applications become more complex, frameworks become a very useful and necessary tool. One of them is Yii (YesItIs), a high-performance PHP framework for rapid development of modern web applications. Among them, components are a very important part of the Yii framework and can integrate different functions into an application. This article will take an in-depth look at the components in the Yii framework and their roles. Component introduction A component in the Yii framework refers to any unit that organizes code. A component can be an object, module or
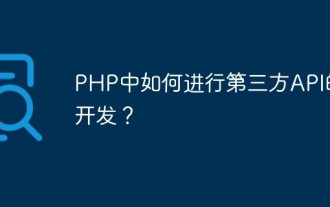
With the popularity of Internet applications, more and more applications need to interact and interconnect with third-party APIs. As one of the commonly used Web development languages, PHP also needs to provide a convenient way to develop third-party APIs. This article will introduce how to develop third-party APIs in PHP, including the following aspects: The concept of third-party APIs Third-party APIs refer to public interfaces provided by other developers or companies to allow other developers or companies to applications are able to access or use the API provided by
