How to use Vue Router to implement data preprocessing before page jump?
How to use Vue Router to implement data preprocessing before page jump?
Introduction:
When using Vue to develop single-page applications, we often use Vue Router to manage jumps between pages. Sometimes, we need to preprocess some data before jumping, such as obtaining data from the server, or verifying user permissions, etc. This article will introduce how to use Vue Router to implement data preprocessing before page jump.
1. Install and configure Vue Router
First, we need to install Vue Router. Run the following command in the command line:
npm install vue-router --save
In the entry file of the project (such as main.js), you need to introduce Vue Router and configure routing:
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter) const routes = [ // 定义路由 ] const router = new VueRouter({ routes }) new Vue({ router, render: h => h(App) }).$mount('#app')
2. Use Vue Router’s beforeEach Hook
Vue Router provides a global hook function named beforeEach
, which performs specific operations before each route jump. We can perform data preprocessing in this hook function.
The following is an example, assuming that we need to get data from the server before jumping to a certain page:
router.beforeEach((to, from, next) => { // 在这里进行数据预处理的操作 fetchDataFromServer(to.path) .then(data => { // 将数据保存到Vue实例或者Vuex中 store.commit('setData', data) next() }) .catch(error => { console.error('数据获取失败', error) next(false) // 停止路由跳转 }) })
In the above code, the fetchDataFromServer
function is a return The asynchronous function of Promise object, which can be used to send requests to the server and obtain data. After the data is successfully obtained, we save the data to the Vue instance or Vuex for use in the target page. If the data acquisition fails, we can stop the routing jump by next(false)
.
3. Unregister the hook function
After we complete the preprocessing of the data, we also need to unregister the hook function to avoid affecting the jump of other pages. When the Vue instance is destroyed, we need to remove the hook function from the Vue Router:
const unregisterHook = router.beforeEach((to, from, next) => { // 钩子函数的具体操作 }) new Vue({ router, beforeDestroy() { unregisterHook() // 注销钩子函数 }, render: h => h(App) }).$mount('#app')
In the above code, the unregisterHook
function returns a cancellation function, which we can save In a Vue instance, the hook function can be unregistered by calling this function when the Vue instance is destroyed.
Summary:
It is very simple to use Vue Router to implement data preprocessing before page jump. We only need to use the beforeEach
hook function to obtain and process data before jumping, and then save the data to the Vue instance or Vuex. At the same time, we need to log out the hook function when the Vue instance is destroyed to ensure that it does not affect the jump of other pages. In this way, we can control page jumps more flexibly and perform necessary data preprocessing operations.
Code example:
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter) const fetchDataFromServer = async (path) => { // 向服务器发送请求并获取数据的逻辑 } const routes = [ // 定义路由 ] const router = new VueRouter({ routes }) const unregisterHook = router.beforeEach((to, from, next) => { fetchDataFromServer(to.path) .then(data => { // 将数据保存到Vue实例或者Vuex中 store.commit('setData', data) next() }) .catch(error => { console.error('数据获取失败', error) next(false) // 停止路由跳转 }) }) new Vue({ router, beforeDestroy() { unregisterHook() // 注销钩子函数 }, render: h => h(App) }).$mount('#app')
The above is the method and sample code for using Vue Router to implement data preprocessing before page jump. By using the beforeEach
hook function, we can perform data preprocessing operations before routing jumps, thereby better controlling the page jump process. I hope this article will be helpful to you when developing single-page applications using Vue Router.
The above is the detailed content of How to use Vue Router to implement data preprocessing before page jump?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










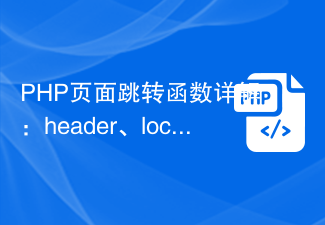
Detailed explanation of PHP page jump functions: Page jump techniques for header, location, redirect and other functions, which require specific code examples. Introduction: When developing a Web website or application, jumping between pages is an essential function. PHP provides a variety of ways to implement page jumps, including header functions, location functions, and jump functions provided by some third-party libraries, such as redirect. This article will introduce in detail how to use these functions
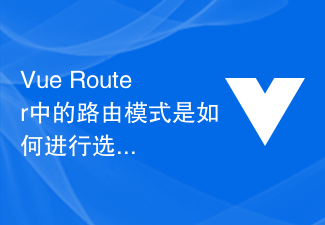
VueRouter is the routing manager officially provided by Vue.js. It can help us implement page navigation and routing functions in Vue applications. When using VueRouter, we can choose different routing modes according to actual needs. VueRouter provides three routing modes, namely hash mode, history mode and abstract mode. The following will introduce in detail the characteristics of these three routing modes and how to choose the appropriate routing mode. Hash mode (default
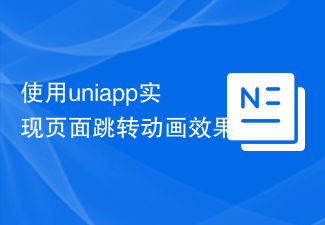
Title: Using uniapp to achieve page jump animation effect In recent years, the user interface design of mobile applications has become one of the important factors in attracting users. Page jump animation effects play an important role in improving user experience and visualization effects. This article will introduce how to use uniapp to achieve page jump animation effects, and provide specific code examples. uniapp is a cross-platform application development framework developed based on Vue.js. It can compile and generate applications for multiple platforms such as mini programs, H5, and App through a set of codes.
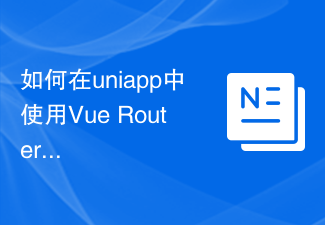
How to use VueRouter for routing jumps in uniapp Using VueRouter for routing jumps in uniapp is a very common operation. This article will introduce in detail how to use VueRouter in the uniapp project and provide specific code examples. 1. Install VueRouter Before using VueRouter, we need to install it first. Open the command line, enter the root directory of the uniapp project, and then execute the following command to install
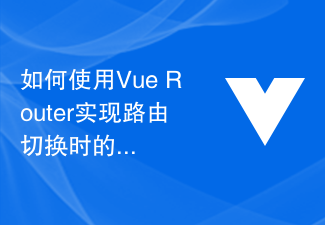
How to use VueRouter to achieve transition effect when routing switching? Introduction: VueRouter is a routing management library officially recommended by Vue.js for building SPA (SinglePageApplication). It can achieve switching between pages by managing the correspondence between URL routing and components. In actual development, in order to improve user experience or meet design needs, we often use transition effects to add dynamics and beauty to page switching. This article will introduce how to use
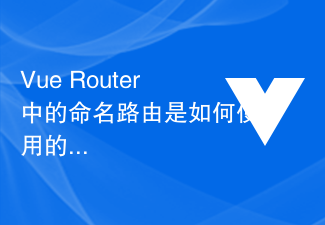
How to use named routes in VueRouter? In Vue.js, VueRouter is an officially provided routing manager that can be used to build single-page applications. VueRouter allows developers to define routes and map them to specific components to control jumps and navigation between pages. Named routing is one of the very useful features. It allows us to specify a name in the routing definition, and then jump to the corresponding route through the name, making the routing jump more convenient.
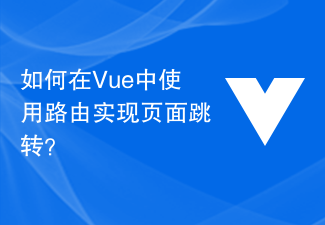
How to use routing to implement page jump in Vue? With the continuous development of front-end development technology, Vue.js has become one of the most popular front-end frameworks. In Vue development, page jump is an essential part. Vue provides VueRouter to manage application routing, and seamless switching between pages can be achieved through routing. This article will introduce how to use routing to implement page jumps in Vue, with code examples. First, install the vue-router plugin in the Vue project.
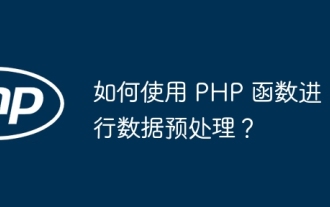
PHP data preprocessing functions can be used for type conversion, data cleaning, date and time processing. Specifically, type conversion functions allow variable type conversion (such as int, float, string); data cleaning functions can delete or replace invalid data (such as is_null, trim); date and time processing functions can perform date conversion and formatting (such as date, strtotime, date_format).
