


Browser compatibility and adaptation of small programs developed using PHP
Browser compatibility and adaptation using PHP to develop small programs
With the development of the Internet, small programs have become a very popular application form. As a commonly used server-side scripting language, PHP is widely used in the development of websites and applications. When developing small programs, how to deal with browser compatibility and adaptability issues is a very important consideration. This article will explore how to deal with browser compatibility and adaptability issues when developing small programs using PHP, and provide some practical code examples.
Browser compatibility refers to the ability of a small program to run correctly in different browsers. Different browsers have some differences in the parsing and execution of HTML, CSS and JavaScript, so certain browser compatibility processing is required when developing small programs.
A common way to deal with this is to use browser proxy detection. The user's browser information is obtained through PHP, and then the code of the mini program is processed differently according to the type and version of the browser. The following is a sample code:
<?php // 获取用户的浏览器信息 $user_agent = $_SERVER['HTTP_USER_AGENT']; // 判断用户使用的浏览器,并作出不同处理 if (strpos($user_agent, 'MSIE') !== false) { // IE浏览器的处理 echo "您正在使用IE浏览器。"; } elseif (strpos($user_agent, 'Firefox') !== false) { // Firefox浏览器的处理 echo "您正在使用Firefox浏览器。"; } elseif (strpos($user_agent, 'Chrome') !== false) { // Chrome浏览器的处理 echo "您正在使用Chrome浏览器。"; } elseif (strpos($user_agent, 'Safari') !== false) { // Safari浏览器的处理 echo "您正在使用Safari浏览器。"; } else { // 其他浏览器的处理 echo "您正在使用其他浏览器。"; } ?>
In the above code, the user's browser information is obtained by using PHP's $_SERVER['HTTP_USER_AGENT']
global variable. Then use the strpos()
function to determine the user's browser type and output different information according to different browser types.
In addition to browser compatibility, the adaptability of small programs is also an issue that needs to be considered. Adaptability refers to the ability of a mini program to display and run correctly on different screen sizes and devices.
When developing small programs in PHP, you can use CSS media queries to adapt to different screen sizes. The following is a sample code:
<?php // 判断当前设备的屏幕宽度,并作出不同处理 if (isset($_SERVER['HTTP_USER_AGENT']) && preg_match('/iphone/i',strtolower($_SERVER['HTTP_USER_AGENT'])) === 1) { echo '<link rel="stylesheet" type="text/css" href="iphone.css">'; } elseif (isset($_SERVER['HTTP_USER_AGENT']) && preg_match('/ipad/i',strtolower($_SERVER['HTTP_USER_AGENT'])) === 1) { echo '<link rel="stylesheet" type="text/css" href="ipad.css">'; } else { echo '<link rel="stylesheet" type="text/css" href="default.css">'; } ?>
In the above code, the user's device information is obtained by using the $_SERVER['HTTP_USER_AGENT']
global variable. Then use the preg_match()
function to determine the device type according to the device type, and introduce different style files according to different device types.
In addition to using media queries to implement adaptation, JavaScript can also be used to dynamically change the layout and style of the mini program. The following is a sample code:
<?php // 判断当前设备的屏幕宽度 if (isset($_SERVER['HTTP_USER_AGENT']) && preg_match('/iphone/i',strtolower($_SERVER['HTTP_USER_AGENT'])) === 1) { ?> <script type="text/javascript"> // 在iPhone上动态改变小程序的布局和样式 document.getElementById('example').style.width = '100%'; </script> <?php } elseif (isset($_SERVER['HTTP_USER_AGENT']) && preg_match('/ipad/i',strtolower($_SERVER['HTTP_USER_AGENT'])) === 1) { ?> <script type="text/javascript"> // 在iPad上动态改变小程序的布局和样式 document.getElementById('example').style.width = '50%'; </script> <?php } else { ?> <script type="text/javascript"> // 在其他设备上动态改变小程序的布局和样式 document.getElementById('example').style.width = '75%'; </script> <?php } ?>
In the above code, different JavaScript codes are used on different devices to change the layout and style of the mini program. By dynamically modifying the attribute values of DOM elements, adaptation on different devices can be achieved.
Through the above code examples, we can see how to use PHP to deal with browser compatibility and adaptability issues of mini programs. Through browser proxy detection and media query, mini programs can be displayed and run correctly on different browsers and devices. At the same time, by dynamically modifying the attribute values of DOM elements, adaptation on different devices can also be achieved. In actual development work, based on specific needs and target devices, the code can be further improved and optimized to enhance the user experience of the mini program.
The above is the detailed content of Browser compatibility and adaptation of small programs developed using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










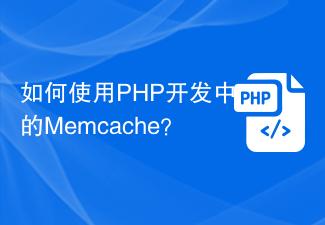
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
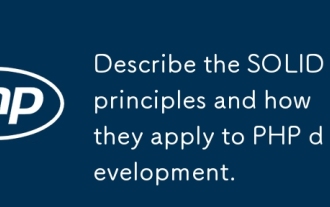
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
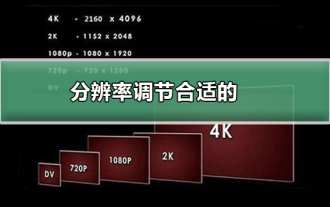
After reinstalling the system, we may not have the resolution we want, and some resolutions may look very uncomfortable. The reason may be that the graphics card driver is not installed or the driver version is out of date. After installing a driver, the problem will appear after opening it. You will be prompted for the drivers you need to install. After all are installed, restart the computer. Generally, it can automatically match the resolution suitable for your monitor. If it still doesn't work, we need to customize one. Let's take a look at the details. There is no suitable solution for the resolution. 1. Install driver life, update all drivers according to the prompts, and then restart the computer; 2. Right-click the computer desktop and select NVIDIA Control Panel to open. If not, click the start menu program in the lower left corner of the computer. 3. Select <Change Resolution>→<Customize>
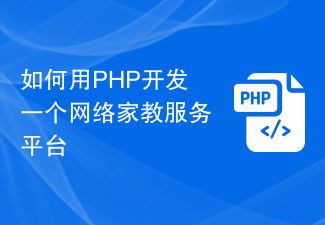
How to use PHP to develop an online tutoring service platform. With the rapid development of the Internet, online tutoring service platforms have attracted more and more people's attention and demand. Parents and students can easily find suitable tutors through such a platform, and tutors can also better demonstrate their teaching abilities and advantages. This article will introduce how to use PHP to develop an online tutoring service platform. First, we need to clarify the functional requirements of the platform. An online tutoring service platform needs to have the following basic functions: Registration and login system: users can
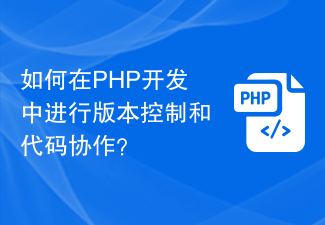
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
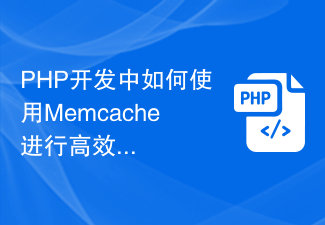
How to use Memcache for efficient data writing and querying in PHP development? With the continuous development of Internet applications, the requirements for system performance are getting higher and higher. In PHP development, in order to improve system performance and response speed, we often use various caching technologies. One of the commonly used caching technologies is Memcache. Memcache is a high-performance distributed memory object caching system that can be used to cache database query results, page fragments, session data, etc. By storing data in memory
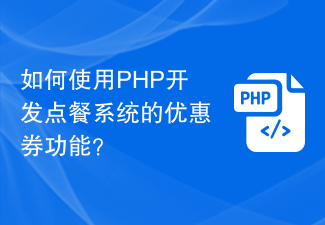
How to use PHP to develop the coupon function of the ordering system? With the rapid development of modern society, people's life pace is getting faster and faster, and more and more people choose to eat out. The emergence of the ordering system has greatly improved the efficiency and convenience of customers' ordering. As a marketing tool to attract customers, the coupon function is also widely used in various ordering systems. So how to use PHP to develop the coupon function of the ordering system? 1. Database design First, we need to design a database to store coupon-related data. It is recommended to create two tables: one
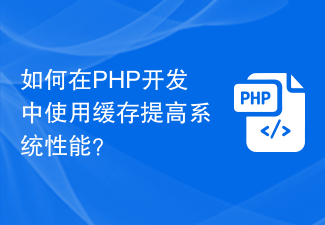
How to use caching to improve system performance in PHP development? In today's era of rapid Internet development, system performance has become a crucial indicator. For PHP development, caching is an important means to improve system performance. This article will explore how to use caching in PHP development to improve system performance. 1. Why use caching to improve system performance: Caching can reduce frequent access to resources such as databases, thereby reducing system response time and improving system performance and throughput. Reduce server load: By using caching, you can reduce
