


How to write a brand management system based on sentiment analysis using Java
With the rise of social media, brand management has become more and more important, and brand reputation has become the key to business success. Sentiment analysis is an effective data analysis technique that helps businesses understand how consumers feel about their brands. In this article, we will explore how to write a brand management system based on sentiment analysis using Java.
- Design database architecture
First, we need to design the database architecture. Considering that sentiment analysis requires processing large amounts of data, we recommend using a relational database management system (RDBMS) such as MySQL or PostgreSQL. Here are some examples:
- Brand table (Brand): stores information about the brand, including name, description, logo, etc.
- Comment table (Comment): stores all comments about the brand, including comment content, rating, date, user ID, etc.
- User table (User): stores user information, including user name, email address, password, etc.
We can also add other tables to store information about the brand, such as statistical information tables (Statistic).
- Crawling data
We need to crawl brand-related data on social media, forums and other sites. This can be achieved through a web crawler in Java. We can use the Jsoup library in Java to crawl HTML pages and use regular expressions to extract useful data from the pages.
For example, we can crawl all Twitter data related to a certain brand name. The following is a simple example:
String url = "https://twitter.com/search?q=" + brandName + "&src=typd"; Document doc = Jsoup.connect(url).get(); Elements tweets = doc.select("li.js-stream-item"); for (Element tweet : tweets) { String text = tweet.select("p.tweet-text").text(); String date = tweet.select("a.tweet-timestamp").attr("title"); String username = tweet.select("span.username").text(); //Save the data to the Comment table }
- Implementing Sentiment Analysis Algorithm
We can use natural language processing technology to implement sentiment analysis algorithms. There are many libraries available for natural language processing in Java, the most popular of which is Stanford CoreNLP. We can use this to analyze sentiment in reviews.
The following is a simple example:
Properties props = new Properties(); props.setProperty("annotators", "tokenize, ssplit, pos, lemma, parse, sentiment"); StanfordCoreNLP pipeline = new StanfordCoreNLP(props); Annotation annotation = pipeline.process(commentText); int sentiment = Integer.parseInt(annotation.get(SentimentCoreAnnotations.SentimentClass.class)); // Save the sentiment to the Comment table
We need to add a column named Sentiment to the database to save the sentiment score of the review.
- Implementing brand management functions
We can create a web-based user interface to display brand information and comments. Users can view a brand's description, logo and all reviews. They can also add new reviews and rate brands. We need to use a Web framework in Java, such as Spring or Struts, to implement the Web interface and business logic.
The following is a simple example:
@Controller public class BrandController { @Autowired private BrandService brandService; @RequestMapping(value="/brand/{id}", method=RequestMethod.GET) public ModelAndView viewBrand(@PathVariable("id") long brandId) { ModelAndView mv = new ModelAndView("brand"); Brand brand = brandService.getBrandById(brandId); mv.addObject("brand", brand); List<Comment> comments = brandService.getCommentsByBrandId(brandId); mv.addObject("comments", comments); return mv; } @RequestMapping(value="/brand/{id}", method=RequestMethod.POST) public ModelAndView addComment(@PathVariable("id") long brandId, Comment comment) { ModelAndView mv = new ModelAndView("redirect:/brand/{id}"); brandService.addComment(comment); return mv; } }
We also need to implement the corresponding Service and DAO classes to handle business logic and database access.
- Integrate third-party API
We can use third-party APIs to improve our brand management system. For example, we can get information about certain regions from the Google Maps API and display the brand's relevant locations on the web interface. You can also use social media APIs, such as the Twitter API, to get more data about your brand.
The following is a simple example:
//Get the brand's coordinates from Google Maps String url = "https://maps.googleapis.com/maps/api/geocode/json?address=" + brand.getAddress() + "&key=" + apiKey; JsonReader jsonReader = Json.createReader(new URL(url).openStream()); JsonObject jsonObject = jsonReader.readObject(); JsonArray results = jsonObject.getJsonArray("results"); JsonObject location = results.getJsonObject(0).getJsonObject("geometry").getJsonObject("location"); double lat = location.getJsonNumber("lat").doubleValue(); double lng = location.getJsonNumber("lng").doubleValue(); //Save the coordinates to the Brand table
In short, sentiment analysis is one of the important methods for managing brand reputation. Using Java to write a brand management system based on sentiment analysis can help companies understand how customers think about their brands and take appropriate actions. This is just a simple example, you can change and extend it according to your actual situation.
The above is the detailed content of How to write a brand management system based on sentiment analysis using Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










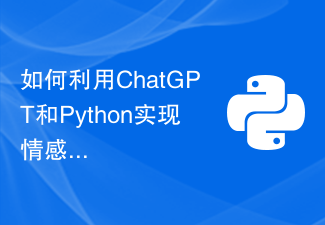
How to use ChatGPT and Python to implement sentiment analysis function Introduction ChatGPTCChatGPT is a generative pre-training model based on reinforcement learning released by OpenAI in 2021. It uses a powerful language model to generate coherent dialogue. ChatGPT can be used for a variety of tasks, including sentiment analysis. Importing libraries and models First, you need to install Python’s relevant libraries and import them, including OpenAI’s GPT library. Then you need to use OpenAI's Ch
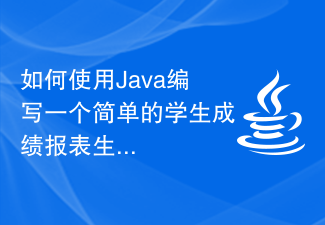
How to write a simple student performance report generator using Java? Student Performance Report Generator is a tool that helps teachers or educators quickly generate student performance reports. This article will introduce how to use Java to write a simple student performance report generator. First, we need to define the student object and student grade object. The student object contains basic information such as the student's name and student number, while the student score object contains information such as the student's subject scores and average grade. The following is the definition of a simple student object: public
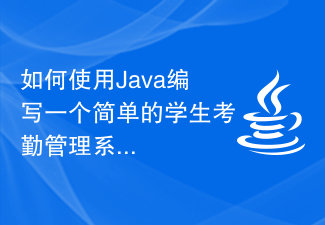
How to write a simple student attendance management system using Java? With the continuous development of technology, school management systems are also constantly updated and upgraded. The student attendance management system is an important part of it. It can help the school track students' attendance and provide data analysis and reports. This article will introduce how to write a simple student attendance management system using Java. 1. Requirements Analysis Before starting to write, we need to determine the functions and requirements of the system. Basic functions include registration and management of student information, recording of student attendance data and
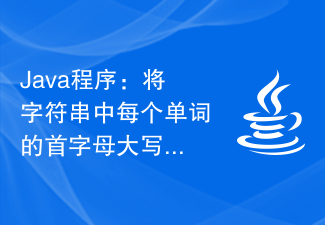
Astringisaclassof'java.lang'packagethatstoresaseriesofcharacters.ThosecharactersareactuallyString-typeobjects.Wemustenclosethevalueofstringwithindoublequotes.Generally,wecanrepresentcharactersinlowercaseanduppercaseinJava.And,itisalsopossibletoconver
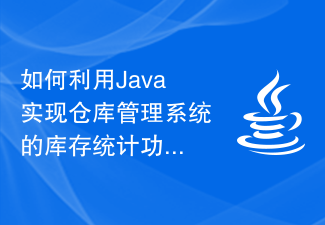
How to use Java to implement the inventory statistics function of the warehouse management system. With the development of e-commerce and the increasing importance of warehousing management, the inventory statistics function has become an indispensable part of the warehouse management system. Warehouse management systems written in the Java language can implement inventory statistics functions through concise and efficient code, helping companies better manage warehouse storage and improve operational efficiency. 1. Background introduction Warehouse management system refers to a management method that uses computer technology to perform data management, information processing and decision-making analysis on an enterprise's warehouse. Inventory statistics are
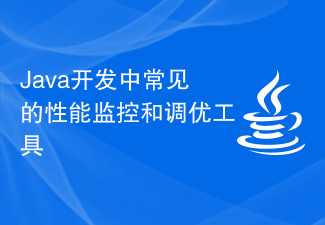
Common performance monitoring and tuning tools in Java development require specific code examples Introduction: With the continuous development of Internet technology, Java, as a stable and efficient programming language, is widely used in the development process. However, due to the cross-platform nature of Java and the complexity of the running environment, performance issues have become a factor that cannot be ignored in development. In order to ensure high availability and fast response of Java applications, developers need to monitor and tune performance. This article will introduce some common Java performance monitoring and tuning
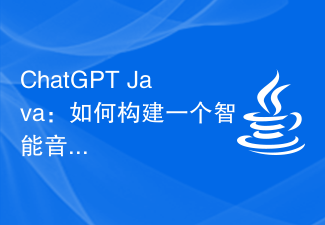
ChatGPTJava: How to build an intelligent music recommendation system, specific code examples are needed. Introduction: With the rapid development of the Internet, music has become an indispensable part of people's daily lives. As music platforms continue to emerge, users often face a common problem: how to find music that suits their tastes? In order to solve this problem, the intelligent music recommendation system came into being. This article will introduce how to use ChatGPTJava to build an intelligent music recommendation system and provide specific code examples. No.
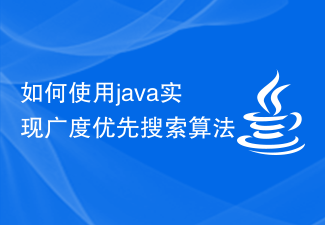
How to use Java to implement breadth-first search algorithm Breadth-First Search algorithm (Breadth-FirstSearch, BFS) is a commonly used search algorithm in graph theory, which can find the shortest path between two nodes in the graph. BFS is widely used in many applications, such as finding the shortest path in a maze, web crawlers, etc. This article will introduce how to use Java language to implement the BFS algorithm, and attach specific code examples. First, we need to define a class for storing graph nodes. This class contains nodes
