PHP Basics Tutorial: From Beginner to Master
PHP is a widely used open source server-side scripting language that can handle all tasks in web development. PHP is widely used in web development, especially for its excellent performance in dynamic data processing, so it is loved and used by many developers. In this article, we will explain the basics of PHP step by step to help beginners from getting started to becoming proficient.
1. Basic syntax
PHP is an interpreted language whose code is similar to HTML, CSS and JavaScript. Every PHP statement ends with a semicolon ;
, and comments start and end with //
or /* */
. The following is a simple example:
<?php //这是注释 echo "Hello World!"; ?>
2. Variables and data types
In PHP, variables are used to store various types of data. Variable names begin with $
, followed by the name of the variable. The following is a simple example:
<?php $name = "John"; $age = 25; echo "My name is ".$name." and I am ".$age." years old."; ?>
PHP supports multiple types of data, including strings, integers, floating point numbers, Boolean values, arrays and objects, etc. The following are some common variables and data types:
$name = "John"; //字符串 $age = 25; //整数 $price = 1.99; //浮点数 $isMale = true; //布尔值 $cities = array("New York", "Los Angeles", "Chicago"); //数组
3. Operators
PHP supports a variety of operators, including arithmetic operators, comparison operators, logical operators and bitwise operators wait. The following are some common operators:
//算术运算符 $x = 10; $y = 5; echo $x + $y; //15 echo $x - $y; //5 echo $x * $y; //50 echo $x / $y; //2 //比较运算符 $x = 10; $y = "10"; var_dump($x == $y); //布尔值true,因为值相等 var_dump($x === $y); //布尔值false,因为类型不同 //逻辑运算符 $x = 10; $y = 5; echo $x > 5 && $y > 5; //true,因为$x大于5且$y大于5 echo $x > 5 || $y > 5; //true,因为$x大于5或$y大于5 //位运算符 $x = 2; //二进制为10 $y = 3; //二进制为11 echo $x & $y; //2,因为二进制10和11进行与运算后得到10 echo $x | $y; //3,因为二进制10和11进行或运算后得到11
4. Process control
PHP supports a variety of process control statements, including conditional statements, loop statements, and jump statements. The following are some common flow control statements:
//条件语句 $x = 10; if ($x > 5) { echo "x is greater than 5"; } elseif ($x < 5) { echo "x is less than 5"; } else { echo "x is equal to 5"; } //循环语句 $i = 0; while ($i < 10) { echo $i; $i++; } for ($i = 0; $i < 10; $i++) { echo $i; } //跳转语句 $x = 10; switch ($x) { case 10: echo "x is equal to 10"; break; case 20: echo "x is equal to 20"; break; default: echo "x is not equal to 10 or 20"; }
5. Functions and arrays
Functions and arrays are commonly used data structures in PHP programming. Functions are used to encapsulate reusable code, and arrays are used to store multiple values. The following are some commonly used functions and arrays:
//函数 function greeting($name) { echo "Hello ".$name; } greeting("John"); //数组 $cities = array("New York", "Los Angeles", "Chicago"); echo count($cities); //3 echo $cities[0]; //"New York"
6. File operations and databases
PHP can read and write files, and can connect to the database to store and read data. Pick. The following are some common file operations and database connections:
//文件操作 $filename = "example.txt"; $file = fopen($filename, "w"); fwrite($file, "This is an example"); fclose($file); //数据库连接 $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; $conn = mysqli_connect($servername, $username, $password, $dbname); if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } $sql = "SELECT * FROM customers"; $result = mysqli_query($conn, $sql); while ($row = mysqli_fetch_assoc($result)) { echo "Name: ".$row["name"]." - Email: ".$row["email"]; } mysqli_close($conn);
7. Error handling
In PHP programming, error handling is very important. PHP provides some built-in error handling functions and statements that can help us find and solve errors in the program. The following are some common error handling functions and statements:
//错误处理函数 function custom_error($errno, $errstr) { echo "<b>Error:</b> [$errno] $errstr"; } set_error_handler("custom_error"); echo($test); //出现错误 //错误处理语句 try { $conn = new PDO("mysql:host=localhost;dbname=myDB", "username", "password"); $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $sql = "SELECT * FROM customers"; $result = $conn->query($sql); foreach ($result as $row) { echo "Name: ".$row["name"]." - Email: ".$row["email"]; } } catch(PDOException $e) { echo "Error:".$e->getMessage(); } $conn = null;
Conclusion:
PHP has a wide range of application fields. It can be used in conjunction with languages such as HTML, CSS and JavaScript to create rich Web application. By studying this article, readers should be able to master the basic knowledge of the PHP language and be able to write simple PHP programs. To further improve PHP programming skills, readers can refer to more advanced tutorials to learn advanced technologies such as PHP object-oriented programming, frameworks, and libraries.
The above is the detailed content of PHP Basics Tutorial: From Beginner to Master. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
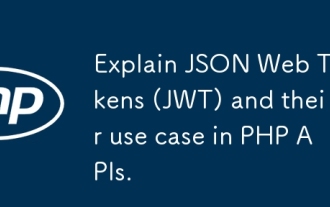
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
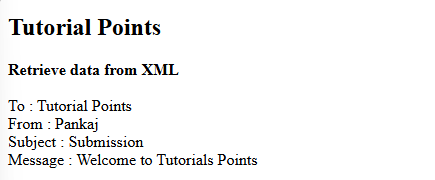
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
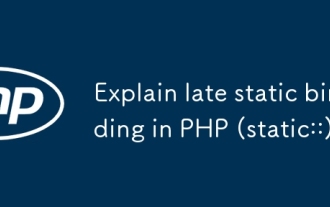
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
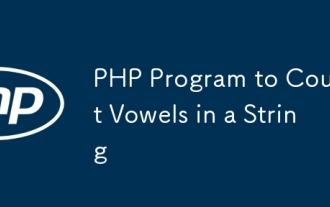
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
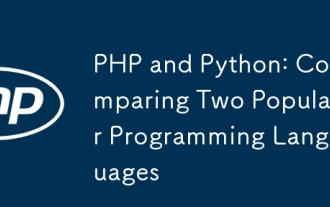
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
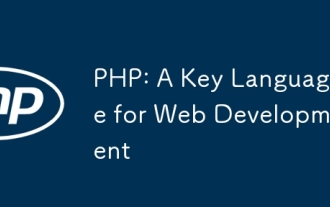
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
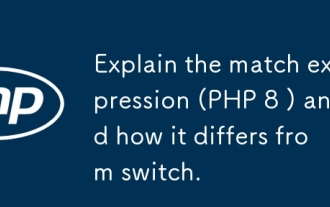
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.
