


How to create a high-performance MySQL data processing pipeline using Go language
With the rapid development of the Internet field, a large amount of data needs to be processed and managed efficiently. In this process, the database has become an indispensable tool. As a high-performance, scalable, open source relational database, MySQL has received more and more attention and use. In order to better utilize the performance of MySQL, using Go language for data processing has become a good choice. This article will introduce how to use Go language to create a high-performance MySQL data processing pipeline.
1. Why use Go language?
The Go language comes with powerful concurrency capabilities. Through the combination of coroutines and pipelines, efficient data processing can be achieved. When processing large amounts of data, using Go language consumes more CPU and memory than other languages. In addition, the Go language is highly efficient in development and easy to maintain. Based on the above advantages, using Go language for MySQL data processing is a good choice.
2. Implementation ideas
- Enable MySQL
To operate MySQL in Go language, you need to install the corresponding driver first. Currently the most widely used one is go-sql-driver/mysql, which can be installed through the following command:
go get -u github.com/go-sql-driver/mysql
After the installation is completed, the driver needs to be introduced into the code:
import ( "database/sql" _ "github.com/go-sql-driver/mysql" )
- Connecting to MySQL
To connect to MySQL in Go language, you need to use the sql.Open function. The first parameter of this function is the driver name, and the second parameter is the database DSN string. The DSN string format is as follows:
user:password@tcp(host:port)/dbname
Among them, user and password are the username and password required to log in to MySQL, host and port are the address and port number of the MySQL server, and dbname is the name of the database that needs to be connected. MySQL connection can be achieved through the following code:
db, err := sql.Open("mysql", "user:password@tcp(host:port)/dbname") if err != nil { panic(err) }
- Processing data
In the process of MySQL data processing, the pipeline mechanism of the Go language can be used to realize the flow of data processing. . Specifically, the data can be read from MySQL, passed to the processing function through a pipeline, and finally the processed data can be written to MySQL through another pipeline. The following is a sample code:
func main() { db, err := sql.Open("mysql", "user:password@tcp(host:port)/dbname") if err != nil { panic(err) } defer db.Close() rows, err := db.Query("SELECT id, name FROM users") if err != nil { panic(err) } defer rows.Close() // 创建两个管道分别用于读取数据和写入数据 dataCh := make(chan User) writeCh := make(chan User) // 启动一个协程用于读取数据并将其发送到dataCh管道中 go func() { for rows.Next() { var u User if err := rows.Scan(&u.ID, &u.Name); err != nil { panic(err) } dataCh <- u } close(dataCh) }() // 启动3个协程用于处理数据,并将处理后的结果发送到writeCh管道中 for i := 0; i < 3; i++ { go func() { for u := range dataCh { // 对数据进行处理 u.Age = getAge(u.Name) u.Gender = getGender(u.Name) writeCh <- u } }() } // 启动一个协程用于将处理后的结果写入到MySQL中 go func() { tx, err := db.Begin() if err != nil { panic(err) } defer tx.Rollback() stmt, err := tx.Prepare("INSERT INTO users(id, name, age, gender) VALUES(?, ?, ?, ?)") if err != nil { panic(err) } defer stmt.Close() for u := range writeCh { _, err := stmt.Exec(u.ID, u.Name, u.Age, u.Gender) if err != nil { panic(err) } } tx.Commit() }() // 等待所有协程执行完毕 wg := &sync.WaitGroup{} wg.Add(4) go func() { defer wg.Done() for range writeCh { } }() go func() { defer wg.Done() for range dataCh { } }() wg.Done() } type User struct { ID int Name string Age int Gender string } func getAge(name string) int { return len(name) % 50 } func getGender(name string) string { if len(name)%2 == 0 { return "Female" } else { return "Male" } }
In the above sample code, we first queried the data in the users table through the db.Query function, and then created two pipelines, dataCh and writeCh, for reading and writing. Enter data. At the same time, we also created three coroutines for processing data. The processing function here is relatively simple, just calculating the user's age and gender through the string length and odd and even numbers. Finally, we started a coroutine that writes to MySQL and writes the processed results to MySQL.
3. Summary
Through the above implementation ideas, we can use Go language to create a high-performance MySQL data processing pipeline. Among them, the concurrency capabilities and pipeline mechanism of the Go language have greatly improved the efficiency of data processing, and also brought higher flexibility and maintainability to data processing. I hope this article can be helpful to you, and everyone is welcome to actively discuss it.
The above is the detailed content of How to create a high-performance MySQL data processing pipeline using Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
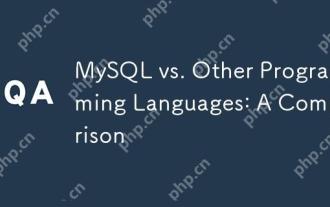
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
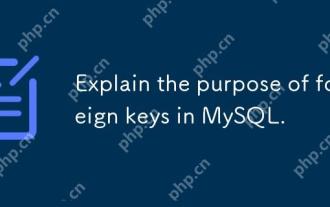
In MySQL, the function of foreign keys is to establish the relationship between tables and ensure the consistency and integrity of the data. Foreign keys maintain the effectiveness of data through reference integrity checks and cascading operations. Pay attention to performance optimization and avoid common errors when using them.
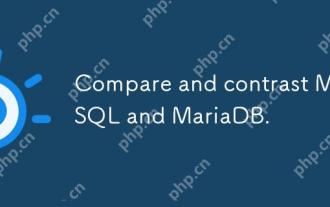
The main difference between MySQL and MariaDB is performance, functionality and license: 1. MySQL is developed by Oracle, and MariaDB is its fork. 2. MariaDB may perform better in high load environments. 3.MariaDB provides more storage engines and functions. 4.MySQL adopts a dual license, and MariaDB is completely open source. The existing infrastructure, performance requirements, functional requirements and license costs should be taken into account when choosing.

SQL is a standard language for managing relational databases, while MySQL is a database management system that uses SQL. SQL defines ways to interact with a database, including CRUD operations, while MySQL implements the SQL standard and provides additional features such as stored procedures and triggers.
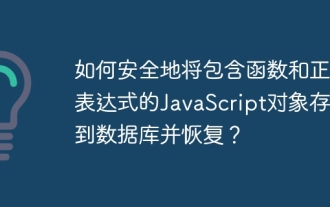
Safely handle functions and regular expressions in JSON In front-end development, JavaScript is often required...
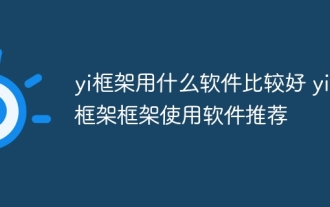
Abstract of the first paragraph of the article: When choosing software to develop Yi framework applications, multiple factors need to be considered. While native mobile application development tools such as XCode and Android Studio can provide strong control and flexibility, cross-platform frameworks such as React Native and Flutter are becoming increasingly popular with the benefits of being able to deploy to multiple platforms at once. For developers new to mobile development, low-code or no-code platforms such as AppSheet and Glide can quickly and easily build applications. Additionally, cloud service providers such as AWS Amplify and Firebase provide comprehensive tools
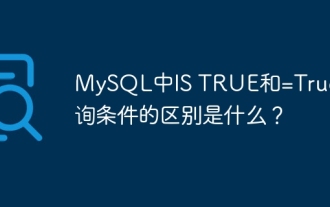
The difference between ISTRUE and =True query conditions in MySQL In MySQL database, when processing Boolean values (Booleans), ISTRUE and =TRUE...
