Use JavaScript to create and display 3D models and visual effects
With the continuous advancement of science and technology, 3D technology is widely used in various fields. In the Internet era, website design and display also need to use 3D technology to attract users' attention. This article will introduce how to use JavaScript to create and display 3D models and visual effects.
1. Use Three.js library to create 3D models
Three.js is a JavaScript 3D graphics library based on WebGL. It allows developers to easily create various 3D scenes and models.
We can create a 3D scene by introducing the Three.js library, as shown below:
<script src="https://cdn.jsdelivr.net/npm/three@0.110.0/build/three.min.js"></script> <div id="scene"></div> <script> const scene = new THREE.Scene(); const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000); const renderer = new THREE.WebGLRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.getElementById('scene').appendChild(renderer.domElement); const geometry = new THREE.BoxGeometry(); const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 }); const cube = new THREE.Mesh(geometry, material); scene.add(cube); camera.position.z = 5; function animate() { requestAnimationFrame(animate); cube.rotation.x += 0.01; cube.rotation.y += 0.01; renderer.render(scene, camera); } animate(); </script>
The above code implements a simple 3D scene with a green cube in it. Can be dragged and rotated with the mouse.
When creating a 3D model, we need to design the shape and material of each object. Three.js provides a variety of basic geometries, such as cubes, spheres, cylinders, etc. You can also use computer-aided design (CAD) tools to create custom geometries.
At the same time, we also need to set the material of each object. Three.js provides many material options, including basic materials, glossy materials, particle materials, and more.
2. Use CSS3DRenderer to create CSS 3D visual effects
In addition to using WebGL to implement 3D models, you can also use CSS3DRenderer to create interactive CSS 3D effects.
When using CSS3DRenderer, we need to first create the corresponding HTML structure and then convert it into CSS 3D elements. For example, the following code creates a CSS 3D meteor shower effect:
<script src="https://cdn.jsdelivr.net/npm/three@0.110.0/build/three.min.js"></script> <div id="container"></div> <script> // 创建3D场景 const scene = new THREE.Scene(); // 创建相机 const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 1, 1000); camera.position.z = 300; // 创建渲染器 const renderer = new THREE.CSS3DRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.getElementById('container').appendChild(renderer.domElement); // 创建流星元素 const divs = []; for (let i = 0; i < 50; i++) { const div = document.createElement('div'); div.style.width = '10px'; div.style.height = '10px'; div.style.background = 'white'; div.style.borderRadius = '50%'; div.style.opacity = '0'; document.body.appendChild(div); const object = new THREE.CSS3DObject(div); object.position.set(Math.random() * window.innerWidth - window.innerWidth / 2, Math.random() * window.innerHeight - window.innerHeight / 2, -Math.random() * 500); scene.add(object); divs.push(object); } // 动画效果 const animate = () => { requestAnimationFrame(animate); divs.forEach((div) => { if (div.position.z > 0) { div.position.set(Math.random() * window.innerWidth - window.innerWidth / 2, Math.random() * window.innerHeight - window.innerHeight / 2, -Math.random() * 500); div.element.style.opacity = '0'; } div.position.z += 10; div.rotation.z += Math.PI / 50; div.element.style.opacity = Number(div.element.style.opacity) + 0.02; }); renderer.render(scene, camera); }; animate(); </script>
The above code implements a meteor shower effect. In CSS3DRenderer, we use CSS styles to design the visual effects of elements, and use CSS3DObject to convert them into 3D elements to achieve 3D effects.
3. Conclusion
Using JavaScript to implement 3D models and visual effects allows us to more easily implement personalized web design. Three.js and CSS3DRenderer are powerful tools for achieving 3D effects, and we can freely combine them to create more unique visual effects.
The above is the detailed content of Use JavaScript to create and display 3D models and visual effects. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










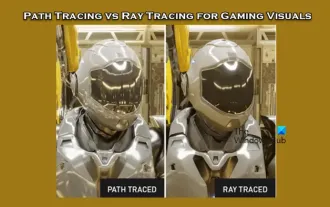
The decision to use path tracing or ray tracing is a critical choice for game developers. Although they both perform well visually, there are some differences in practical applications. Therefore, game enthusiasts need to carefully weigh the advantages and disadvantages of both to determine which technology is more suitable for achieving the visual effects they want. What is ray tracing? Ray tracing is a complex rendering technique used to simulate the propagation and interaction of light in virtual environments. Unlike traditional rasterization methods, ray tracing generates realistic lighting and shadow effects by tracing the path of light, providing a more realistic visual experience. This technology not only produces more realistic images, but also simulates more complex lighting effects, making scenes look more realistic and vivid. its main concepts
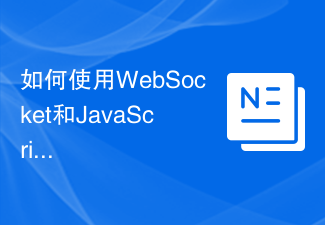
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
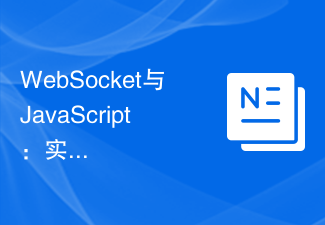
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
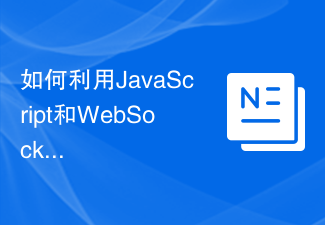
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
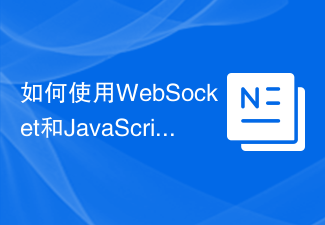
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
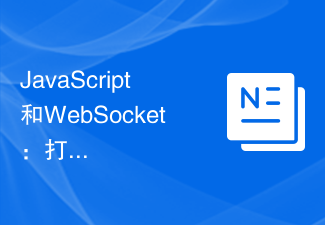
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
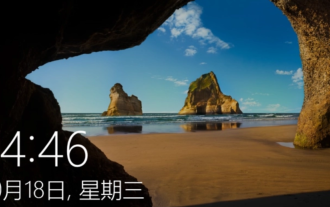
In the Win10 system, the frosted glass translucency effect is a visual special effect that is very beautiful and cool, but many users don’t know how to cancel it after setting it? Let’s take a look below! Method 1: Turn off the frosted glass translucency effect through system settings 1. First, click the "Start" button on the desktop, and then select "Settings". 2. In the settings window, select the "Personalization" option. 3. In the personalization options, select "Color". 4. In the color options, find the "Transparency Effect" item and turn it off. Method 2: Turn off the frosted glass translucency effect through the registry editor 1. First, press the Win+R key combination to open the run window. 2. Enter "regedit" in the run window and press Enter to open the registry editor.
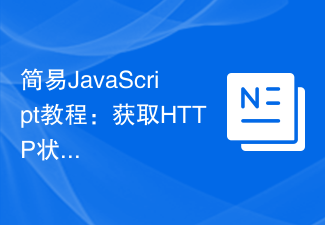
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
