


Laravel development: How to quickly integrate third-party login using Laravel Socialite?
Laravel is a popular PHP framework used for developing web applications. One of the most common features is the integration of third-party logins into the application. Doing so can provide users with a better user experience and reduce repetitive registration processes.
In this article, we will discuss how to integrate third-party login using Laravel Socialite library.
What is Laravel Socialite?
Laravel Socialite is an extension package for the Laravel framework that allows developers to easily implement third-party logins in their applications. It supports various social platforms such as Facebook, Twitter, LinkedIn, etc.
How does Socialite work?
Socialite follows the OAuth protocol. OAuth is an authorization framework that allows users to pass their account information from one site to another without sharing their credentials. OAuth requires user authorization, in which case the user authorizes the application to access their social media accounts.
Socialite authorization works as follows:
-
The user clicks the "Login" button, which will point to Socialite authorization:
Route::get('auth/{provider}', 'AuthSocialController@redirectToProvider');
Copy after login Socialite challenges the user to authorize access to their social media account; Socialite will redirect to the social media site;
Route::get('auth/{provider}/callback', 'AuthSocialController@handleProviderCallback');
Copy after login
3. The user authorizes their account and the social media site redirects to the callback URL;
public function redirectToProvider($provider) { return Socialite::driver($provider)->redirect(); }
Socialite will return the callback URL with the authorization code to the application, and the application will request the OAuth access token with the authorization code;
public function handleProviderCallback($provider) { $socialUser = Socialite::driver($provider)->user(); }
Copy after login- Upon completion of authentication, Socialite will return the user's authorized token and pass it to the application.
How to use Socialite in Laravel?
Using any social media platform supported by Socialite, you will need to set up application credentials or keys in two places:
'facebook' => [ 'client_id' => env('FB_CLIENT_ID'), 'client_secret' => env('FB_CLIENT_SECRET'), 'redirect' => env('FB_CALLBACK_URL'), ] 'twitter' => [ 'client_id' => env('TW_CLIENT_ID'), 'client_secret' => env('TW_CLIENT_SECRET'), 'redirect' => env('TW_CALLBACK_URL'), ], 'google' => [ 'client_id' => env('GOOGLE_CLIENT_ID'), 'client_secret' => env('GOOGLE_CLIENT_SECRET'), 'redirect' => env('GOOGLE_CALLBACK_URL') ]
Application credentials or keys are intended to authenticate the application's identity .
We will set this in the config/services.php file.
Step 1: Create Authorization Routes
Create the routes used to trigger Socialite authorization:
Route::get('auth/{provider}', 'AuthSocialController@redirectToProvider'); Route::get('auth/{provider}/callback', 'AuthSocialController@handleProviderCallback');
Typically these routes are placed in a controller called SocialController, which Controller is used to handle Socialite authorization and callbacks.
Step 2: Create a SocialController
Like all controllers, we need to create a SocialController that uses good coding practices.
Use the following command to create the controller:
php artisan make:controller AuthSocialController
Ultimately this will allow us to use the authorization provider for authorization and callbacks to our routes.
In SocialController, we define two methods, redirectToProvider and handleProviderCallback. The first is a redirect to the authorization provider. The subsequent callback function then provides the callback URL for the client with the authorization code to retrieve the information and complete the authentication.
The following is a sample code for SocialController:
namespace AppHttpControllersAuth; use AppHttpControllersController; use IlluminateSupportFacadesAuth; use IlluminateHttpRequest; use LaravelSocialiteFacadesSocialite; class SocialController extends Controller { /** * Redirect the user to the OAuth Provider. * * @return IlluminateHttpResponse */ public function redirectToProvider($provider) { return Socialite::driver($provider)->redirect(); } /** * Obtain the user information from OAuth Provider. * * @return IlluminateHttpResponse */ public function handleProviderCallback($provider) { $user = Socialite::driver($provider)->user(); // Do something with user data, for example: // $user->token; // $user->getId(); // $user->getEmail(); } }
Step 3: Using a view controller
Normally, we will use a view controller to manage the rendering of all our views. This makes our code easier to read and manage. Let’s create a simple view for our application using Laravel’s view controller.
Use the following command to create the view controller:
php artisan make:controller SocialAuthController
The following are the changes made in the controller.
namespace AppHttpControllers; use IlluminateHttpRequest; class SocialAuthController extends Controller { /** * Redirect the user to the OAuth Provider. * * @return IlluminateHttpResponse */ public function redirectToProvider($provider) { return Socialite::driver($provider)->redirect(); } /** * Obtain the user information from OAuth Provider. * * @return IlluminateHttpResponse */ public function handleProviderCallback($provider) { $user = Socialite::driver($provider)->user(); $existingUser = User::where('email', $user->getEmail())->first(); if ($existingUser) { // If user already exists, login the user auth()->login($existingUser, true); } else { // Register new user $newUser = new User(); $newUser->name = $user->getName(); $newUser->email = $user->getEmail(); $newUser->google_id = $user->getId(); $newUser->password = encrypt('amitthakur'); $newUser->save(); auth()->login($newUser, true); } return redirect()->to('/home'); } }
Step 4: Create the login view
Now our controller and routing are ready. Now we need to create the view for login.
Create a view file and name it social_login.blade.php. We need to display buttons that can trigger third-party logins. In this case we will show 3 buttons to support login with Google, Facebook and Twitter.
The following is the sample code for the view file:
@extends('layouts.app') @section('content') <main class="py-4"> <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">{{ __('Login') }}</div> <div class="card-body"> <a href="{{ route('social.oauth', ['provider' => 'google']) }}" class="btn btn-google">Sign in with google</a> <a href="{{ route('social.oauth', ['provider' => 'facebook']) }}" class="btn btn-facebook">Sign in with Facebook</a> <a href="{{ route('social.oauth', ['provider' => 'twitter']) }}" class="btn btn-twitter">Sign in with twitter</a> </div> </div> </div> </div> </div> </main> @endsection
Use the layout file at the top. When the user clicks on any button, we redirect them to the authorization provider. In SocialController respond we will get user data and complete authentication.
Step 5: Create a new route
Now we need to create a new route to handle the above view file.
Modify the web.php file as follows:
Route::get('social', 'SocialAuthController@index')->name('social.login'); Route::get('social/{provider}', 'SocialAuthController@redirectToProvider')->name('social.oauth'); Route::get('social/{provider}/callback', 'SocialAuthController@handleProviderCallback');
We should note that the routes above are identified by name so that they can be referenced in the controller code.
Step 6: Test
Now that we have our social media identities set up, before we can test the application, we need to configure our application through the .env file.
To configure the .env file, add the following code:
FACEBOOK_CLIENT_ID=xxxxxxxxxxxxxxxxx FACEBOOK_CLIENT_SECRET=xxxxxxxxxxxxxxxxxxxx FACEBOOK_CALLBACK_URL=http://www.example.com/auth/facebook/callback GOOGLE_CLIENT_ID=xxxxxxxxxxxxxxxxx GOOGLE_CLIENT_SECRET=xxxxxxxxxxxxxxxxxxxx GOOGLE_CALLBACK_URL=http://www.example.com/auth/google/callback TWITTER_CLIENT_ID=xxxxxxxxxxxxxxxxx TWITTER_CLIENT_SECRET=xxxxxxxxxxxxxxxxxxxx TWITTER_CALLBACK_URL=http://www.example.com/auth/twitter/callback
Replace the actual application ID with "xxxxxxxxxxxxxxxxxxxx" in this line. Afterwards, we can launch our application via the command line and access the view file above. Once we click any button and authorize our account via OAuth, we are authenticated with every known user.
Conclusion
In this article, we learned how to integrate third-party login using Laravel Socialite library. The main purpose of Socialite is to simplify the social media login process to improve user experience. We learned how to set up Socialite to support Google, Facebook, and Twitter. We also retrieve the authorization code in the controller and complete the OAuth authentication using the requested data. The process will actually create a new account or update an existing account.
The above is the detailed content of Laravel development: How to quickly integrate third-party login using Laravel Socialite?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










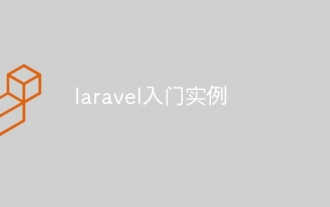
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
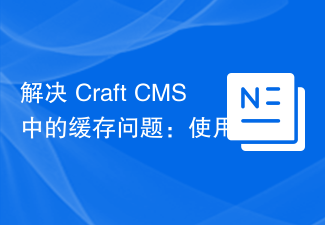
When developing websites using CraftCMS, you often encounter resource file caching problems, especially when you frequently update CSS and JavaScript files, old versions of files may still be cached by the browser, causing users to not see the latest changes in time. This problem not only affects the user experience, but also increases the difficulty of development and debugging. Recently, I encountered similar troubles in my project, and after some exploration, I found the plugin wiejeben/craft-laravel-mix, which perfectly solved my caching problem.
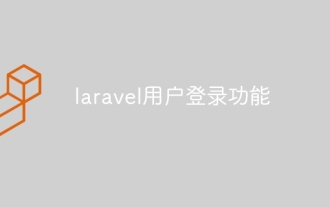
Laravel provides a comprehensive Auth framework for implementing user login functions, including: Defining user models (Eloquent model), creating login forms (Blade template engine), writing login controllers (inheriting Auth\LoginController), verifying login requests (Auth::attempt) Redirecting after login is successful (redirect) considering security factors: hash passwords, anti-CSRF protection, rate limiting and security headers. In addition, the Auth framework also provides functions such as resetting passwords, registering and verifying emails. For details, please refer to the Laravel documentation: https://laravel.com/doc
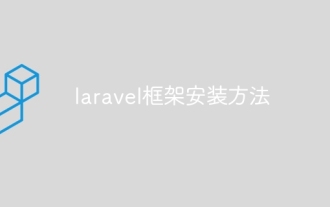
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.
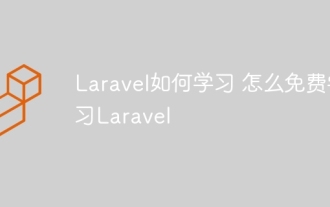
Want to learn the Laravel framework, but suffer from no resources or economic pressure? This article provides you with free learning of Laravel, teaching you how to use resources such as online platforms, documents and community forums to lay a solid foundation for your PHP development journey from getting started to master.
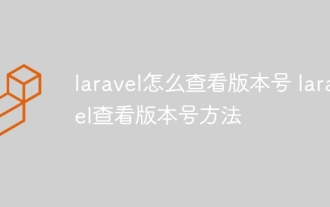
The Laravel framework has built-in methods to easily view its version number to meet the different needs of developers. This article will explore these methods, including using the Composer command line tool, accessing .env files, or obtaining version information through PHP code. These methods are essential for maintaining and managing versioning of Laravel applications.
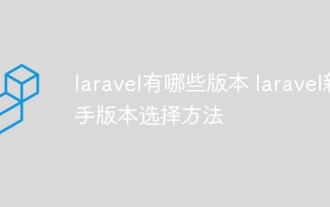
In the Laravel framework version selection guide for beginners, this article dives into the version differences of Laravel, designed to assist beginners in making informed choices among many versions. We will focus on the key features of each release, compare their pros and cons, and provide useful advice to help beginners choose the most suitable version of Laravel based on their skill level and project requirements. For beginners, choosing a suitable version of Laravel is crucial because it can significantly impact their learning curve and overall development experience.
