How to quickly generate a MySQL database diagram
需求描述:
在公司老旧系统里,数据库表很多,但是在设计之初并没有建立好关系图,导致新人刚入职,面对N个库,每个库几百张表,很不方便。
例如:公司某一个系统的库有三百张表,在不熟悉项目的情况下,打开数据库看到一列列的表,很不清晰,对新入职同事很不友好。
需求分析:
我们一个系统里,可能有很多个模块,例如商城系统中有商品模块、券模块、店铺模块等,没个模块都有几十张表,每个模块需要生成如下关系图:(吐槽一下,Navicat逆向的图没PowerDesigner好看)
技术方案:
使用工具:Navicat
Navicat是国内的一款数据库客户端,内置有模型功能,可以实现需求中,选中一个模块的所有表,逆向表到模型,从而生成ER图,但如果选中表中,没有外键关联,生成出来的模型,并没有像需求中那样,有直观的线连接,所以需要给对应表生成外键SQL。
从Navicat中选中所有表导出为SQL时发现下图规律
解决方案:
1. 把每个表语句拆分出来
2. 主表与关联表,主表不需要生产外键,关联表可以通过小撇号判断是否需要外键
3. 说再多文字不如读一遍代码更清晰
package com.example.demo; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.nio.file.Files; import java.nio.file.Paths; import java.util.ArrayList; import java.util.Arrays; import java.util.HashMap; import java.util.List; import java.util.Map; import java.util.Set; import java.util.stream.Collectors; public class MysqlERGenerate { public static void main(String[] args) throws IOException { StringBuilder builder = new StringBuilder(); // 输入从Navicat导出的表结构sql文件 将文件读取出来 放入字符串中 InputStream is = Files.newInputStream(Paths.get("C:\\Users\\admin\\Desktop\\ddl.sql")); String line; BufferedReader reader = new BufferedReader(new InputStreamReader(is)); line = reader.readLine(); while (line != null) { builder.append(line); builder.append("\n"); line = reader.readLine(); } reader.close(); is.close(); String sql = builder.toString(); // 按照规律 使用CREATE TABLE进行分割 并删掉一个文件注释部分 String[] split = sql.split("CREATE TABLE"); List<String> list = new ArrayList<>(Arrays.asList(split)); list.remove(0); // 使用开头两个小撇号进行截取 得到表名 转为Map<表名, SQL> Map<String, String> collect = list.stream().collect(Collectors.toMap(k -> { int firstIndex = k.indexOf("`"); return k.substring(++firstIndex, k.indexOf("`", firstIndex)); }, v -> v)); // 需要创建外键的字段与对应的主表名称 Map<外键名, 外键主表名> Map<String, String> foreignKey = new HashMap<>(); foreignKey.put("ticket_no", "ticket"); foreignKey.put("ticket_define_no", "ticket_define"); foreignKey.put("pro_no", "pro_main"); // 循环判断,生成外键SQL Set<String> foreignKeyFields = foreignKey.keySet(); for (String mainTableName : collect.keySet()) { String val = collect.get(mainTableName); for (String field : foreignKeyFields) { if (!mainTableName.equals(foreignKey.get(field)) && val.indexOf("`" + field + "`") > 0) { String createForeignKeySql = String.format("alter table %s add foreign key %s(%s) references %s(%s);", mainTableName, mainTableName + field + System.currentTimeMillis(), field, foreignKey.get(field), field); System.out.println(createForeignKeySql); } } } } }
运行效果:只复制出部分,实际远比这个多
alter table pro_param add foreign key pro_parampro_no1650765563395(pro_no) references pro_main(pro_no); alter table pro_shop_priority_his_20200805 add foreign key pro_shop_priority_his_20200805pro_no1650765563423(pro_no) references pro_main(pro_no); alter table ticket_define_shop add foreign key ticket_define_shopticket_define_no1650765563423(ticket_define_no) references ticket_define(ticket_define_no); alter table ticket_define_item add foreign key ticket_define_itemticket_define_no1650765563425(ticket_define_no) references ticket_define(ticket_define_no); alter table ticket_his_2019 add foreign key ticket_his_2019ticket_no1650765563432(ticket_no) references ticket(ticket_no); alter table ticket_his_2018 add foreign key ticket_his_2018ticket_no1650765563433(ticket_no) references ticket(ticket_no);
外键语句不要去开发测试生产等环境执行,要自己在本地新建库
新建库时,只转结构就好,不然数据多了,外键语句执行特慢
结束后,想要那些表之间的关系图,只需要选中后逆向表到模型即可得到需求中的效果
如果资金充裕,可以打赏请我喝杯咖啡,谢谢 Thanks♪(・ω・)ノ
The above is the detailed content of How to quickly generate a MySQL database diagram. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










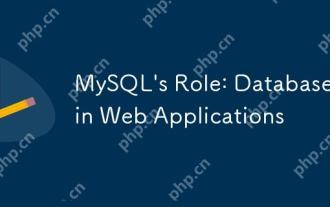
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
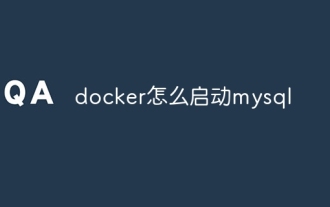
The process of starting MySQL in Docker consists of the following steps: Pull the MySQL image to create and start the container, set the root user password, and map the port verification connection Create the database and the user grants all permissions to the database
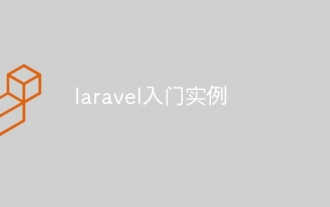
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
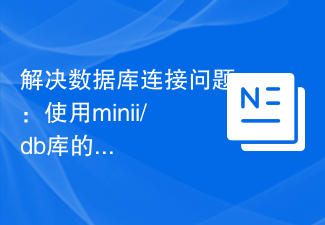
I encountered a tricky problem when developing a small application: the need to quickly integrate a lightweight database operation library. After trying multiple libraries, I found that they either have too much functionality or are not very compatible. Eventually, I found minii/db, a simplified version based on Yii2 that solved my problem perfectly.
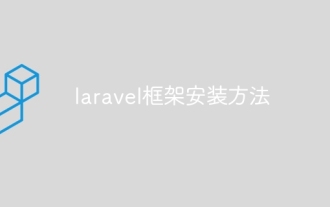
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.
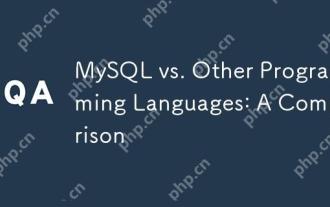
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.

MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
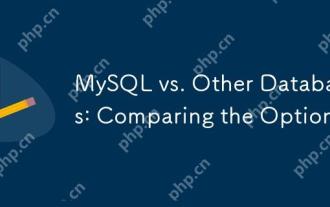
MySQL is suitable for web applications and content management systems and is popular for its open source, high performance and ease of use. 1) Compared with PostgreSQL, MySQL performs better in simple queries and high concurrent read operations. 2) Compared with Oracle, MySQL is more popular among small and medium-sized enterprises because of its open source and low cost. 3) Compared with Microsoft SQL Server, MySQL is more suitable for cross-platform applications. 4) Unlike MongoDB, MySQL is more suitable for structured data and transaction processing.
