What are the common API knowledge points for vue3 component development?
Component-based thinking
Why use component-based development?
Currently popular front-end frameworks such as Vue React will complete business requirements by writing components, which is component-based development. Including small program development, the idea of component development will also be used.
Analyze componentized ideas to develop applications:
Split a complete page into many small components
Each Each component is used to complete a functional module of the page
Each component can also be subdivided (parent and child components)
General components can be duplicated Use different pages
A Vue page should be organized like a nested component tree:
vue3 Entry file:
import { createApp } from 'vue'; import App from './App.vue'; createApp(App).mount('#app');
createApp()
The function passes in an App
, App
is a component and is the root component of the project.
The following will analyze the common methods of component development in Vue3.
Component communication
$props
##$props
Used to pass data to sub-components
<p> $props: {{$props}} </p>
<script setup>
You need to use
definePropsApi to get props
const props = defineProps({ num: Number, })
- ##$emit
- Method used to call the parent component
<button @click="$emit('add')"> add </button>
Copy after login
- <script setup>
- You need to use
defineEmits
Api statement emits
##<div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'><button @click="add">{{ num }}</button> const emits = defineEmits([&#39;add&#39;]) function add() { emits(&#39;add&#39;) }</pre><div class="contentsignin">Copy after login</div></div>
$parent
- is used to obtain the parent component instance object.
<script setup> The component instance will not be exposed, and using
directly in the template will return an empty object. In order to specify the properties to be exposed in the
<script setup>
component, use the
compiler macro: <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>const parData = ref("父组件数据");
defineExpose({
parData,
})</pre><div class="contentsignin">Copy after login</div></div>
Subcomponent: <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'><p>父组件 parData: {{$parent.parData}}</p></pre><div class="contentsignin">Copy after login</div></div>
$attrs
Contains attribute bindings and events in the parent scope that are not components
props- or custom events.
Sub component:
<Foo a="a" b="b" :num="num" @add="add" />
Copy after loginIn the parent component, the value of
is
{ "a": "a", "b": "b" }. When a component does not declare any props, this will contain the bindings of all parent scopes and can be passed through
- Enter internal components - This is very useful when creating higher-order components, for example:
Parent component:
<Bar :age=18 sex="boy" />
Copy after loginChild component
<p v-bind="$attrs">将$attrs对象绑定到当前标签</p>
View the DOM in the browser, age and sex
are bound to the
tag as attributes. ##<script setup>
- useAttrs
import { useAttrs } from 'vue'; const attrs = useAttrs(); console.log(attrs.sex); // boy
Copy after loginproviede & inject
- The parent component has a
provide
option to provide data, Child components have an inject - option to start using this data.
Parent component:
Children component:provide("user", "kong"); provide("pass", 123456);
Copy after login
const user = inject("user"); const pass = inject("pass");
SlotComp Component
<template> <div :> <slot name="head"></slot> </div> <div :> <slot name="foot"></slot> </div> </template>
<SlotComp> <template v-slot:head> <p>head插槽</p> </template> <template v-slot:foot> <p>foot插槽</p> </template> </SlotComp>
SlotComp The component has the name
attribute The contents of theslot slot will be replaced. The replaced content needs to use the
v-slot directive in the parent component to provide the slot with the content you want to display.
Rendering scope
<template v-slot:foot> <p>foot插槽</p> {{msg}} {{items}} </template>
In the above example,
{{items}} will not display data. All content in the parent template is compiled in the parent scope; all content in the child template is compiled in the child scope.
Slot content:Let the slot content access the data only available in the subcomponent:
<slot name="head" :item="items"></slot>Copy after login
<template v-slot:head = "slotProps">
<p v-for="i in slotProps.item">{{i}}</p>
</template>
Copy after login
The attribute bound to the <template v-slot:head = "slotProps"> <p v-for="i in slotProps.item">{{i}}</p> </template>
slot prop
. Now, in the parent scope, we can usev-slot with a value to define the name of the slot prop we provide, in this case
slotProps. v-model
Form component
v-model
to the form to achieve two-way binding:<input v-model="text" />
自定义组件
将
v-model
应用在自定义组件上面:
父组件中使用自定义组件:
const msg = ref('hello world!'); <ModelComp v-model="msg"></ModelComp>
相当于:
<ModelComp :modelValue="msg" @update:modelValue="msg = $event" > </ModelComp>
自定义组件ModelComp.vue
中:
const props = defineProps({ modelValue: String }) const emits = defineEmits([ "update:modelValue" ]) const text = ref("请输入.."); // text改变时执行 watch(text,()=>{ emits("update:modelValue",text.value); })
改变默认参数
未设置参数时如上,默认绑定的参数是
modelValue
update:modelValue
设置v-model
参数:
<ModelComp v-model:show="show"></ModelComp>
相当于:
<ModelComp :show="showFlag" @update:show="showFlag = $event" > </ModelComp>
自定义组件ModelComp.vue
中:
const props = defineProps({ show: Boolean }) const emits = defineEmits([ "update:show", ])
样式绑定相关
class
class绑定:根据需求动态绑定class样式时可以使用一下几种方法
写法1:对象语法
<button @click="changeColor" :class="{ active: isActive }"> 点击切换我的文体颜色样式 </button> const isActive = ref(false); const changeColor = () => { isActive.value = !isActive.value; }
写法2:对象语法
<button @click="changeColor2" :class="classObj"> 点击切换颜色,根据计算属性 </button> const isActive2 = reactive({ active: false, }) const classObj = computed(() => { return { active: isActive2.active, 'font-30': true, } }) const changeColor2 = () => { isActive2.active = !isActive2.active }
写法3:数组语法
<div :class="[activeClass, errorClass]"></div>
三目运算符写法
<div :class="[isActive ? activeClass : '', errorClass]"></div>
数组语法中结合对象语法使用
<div :class="[{ active: isActive }, errorClass]"></div>
style
动态绑定行内style样式
三种方式:html代码
<p :>style绑定</p>
<p :>style对象绑定(直接绑定到一个对象,代码更清新)</p>
<p :>style数组绑定</p>
js 代码
const colorRed = ref('#f00') const styleObj = reactive({ fontSize: '30px', }) const styleObjRed = reactive({ color: '#f00', })
The above is the detailed content of What are the common API knowledge points for vue3 component development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










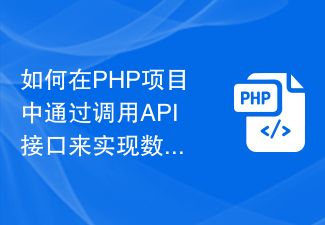
How to crawl and process data by calling API interface in PHP project? 1. Introduction In PHP projects, we often need to crawl data from other websites and process these data. Many websites provide API interfaces, and we can obtain data by calling these interfaces. This article will introduce how to use PHP to call the API interface to crawl and process data. 2. Obtain the URL and parameters of the API interface. Before starting, we need to obtain the URL of the target API interface and the required parameters.

Oracle is a world-renowned database management system provider, and its API (Application Programming Interface) is a powerful tool that helps developers easily interact and integrate with Oracle databases. In this article, we will delve into the Oracle API usage guide, show readers how to utilize data interface technology during the development process, and provide specific code examples. 1.Oracle
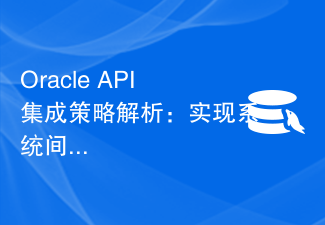
OracleAPI integration strategy analysis: To achieve seamless communication between systems, specific code examples are required. In today's digital era, internal enterprise systems need to communicate with each other and share data, and OracleAPI is one of the important tools to help achieve seamless communication between systems. This article will start with the basic concepts and principles of OracleAPI, explore API integration strategies, and finally give specific code examples to help readers better understand and apply OracleAPI. 1. Basic Oracle API
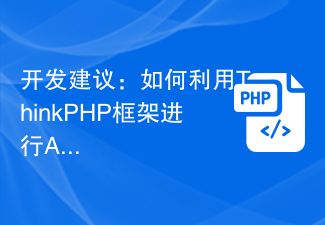
Development suggestions: How to use the ThinkPHP framework for API development. With the continuous development of the Internet, the importance of API (Application Programming Interface) has become increasingly prominent. API is a bridge for communication between different applications. It can realize data sharing, function calling and other operations, and provides developers with a relatively simple and fast development method. As an excellent PHP development framework, the ThinkPHP framework is efficient, scalable and easy to use.
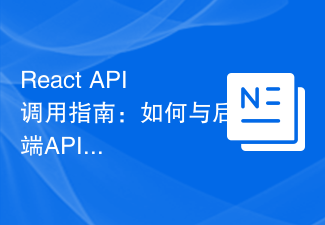
ReactAPI Call Guide: How to interact with and transfer data to the backend API Overview: In modern web development, interacting with and transferring data to the backend API is a common need. React, as a popular front-end framework, provides some powerful tools and features to simplify this process. This article will introduce how to use React to call the backend API, including basic GET and POST requests, and provide specific code examples. Install the required dependencies: First, make sure Axi is installed in the project
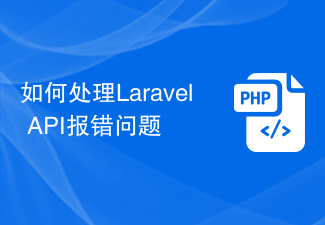
Title: How to deal with Laravel API error problems, specific code examples are needed. When developing Laravel, API errors are often encountered. These errors may come from various reasons such as program code logic errors, database query problems, or external API request failures. How to handle these error reports is a key issue. This article will use specific code examples to demonstrate how to effectively handle Laravel API error reports. 1. Error handling in Laravel
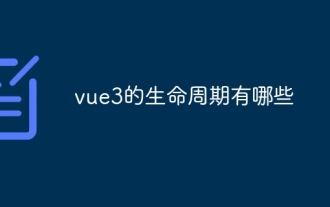
vue3的生命周期:1、beforeCreate;2、created;3、beforeMount;4、mounted;5、beforeUpdate;6、updated;7、beforeDestroy;8、destroyed;9、activated;10、deactivated;11、errorCaptured;12、getDerivedStateFromProps等等
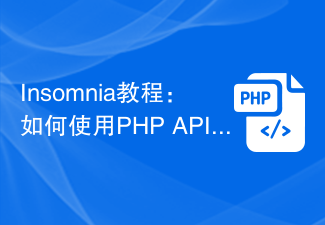
PHP API interface: How to use Insomnia Insomnia is a powerful API testing and debugging tool. It can help developers quickly and easily test and verify API interfaces. It supports multiple programming languages and protocols, including PHP. This article will introduce how to use Insomnia to test PHPAPI interface. Step 1: Install InsomniaInsomnia is a cross-platform application that supports Windows, MacOS, and Linux.
