


How to deploy Golang services on Linux
1. Install Golang and necessary tools
Before deploying Golang services, you first need to install Golang and necessary tools. It can be installed through the following command:
sudo apt-get update sudo apt-get upgrade sudo apt-get install golang sudo apt-get install git
The last line installs the Git tool. Because Golang projects are usually version managed through Git. After the installation is complete, you can check whether the installation is successful by running the following command:
go version git version
If both the above two commands output the version number, it means the installation is successful.
2. Writing Golang services
After installing Golang and the necessary tools, you can start writing Golang services. Taking a simple HTTP service as an example, the following is a code example:
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", helloHandler) fmt.Println("Server started on port 8080") http.ListenAndServe(":8080", nil) } func helloHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") }
The service code has a simple function, listens to the local port 8080, and returns the string "Hello, World!" for each request. Use the http.HandleFunc
method to specify a route and a handler function for the route.
3. Build and test the Golang service
After writing the Golang service code, you need to build and test it. The service can be built using the following command: go build
. This command will generate an executable file in the current directory. Before testing, you need to modify the current user's firewall rules to allow the service to listen on port 8080. You can use the following command:
sudo ufw allow 8080/tcp
After the modification is completed, you can start the service for testing. You can use the following command to start the service:
./<可执行文件名> &
The &
symbol means running the service in the background. After successful startup, you can use a browser or the curl
command to access the service.
4. Use PM2 for Golang service deployment
Manually starting the Golang service is obviously not very friendly, especially when the service needs to be restarted or monitored, it is even more inconvenient. Therefore, PM2 tools can be used for service management and deployment. PM2 is a process management tool in the Node.js ecosystem, but it also supports managing processes written in other languages, including Golang. The following is an example of using PM2 for Golang service deployment:
Install PM2
sudo npm install -g pm2
Enter the directory where the service program is located, And use the following command to start the service:
pm2 start <可执行文件名> --name=<服务名称>
Among them, the --name
parameter is used to specify the name of the service.
You can use the following command to view the status of the service:
pm2 list
For processes that have been managed by PM2, you can Use the following command to operate the service:
pm2 restart <服务名称> pm2 stop <服务名称> pm2 delete <服务名称>
The above is the detailed content of How to deploy Golang services on Linux. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










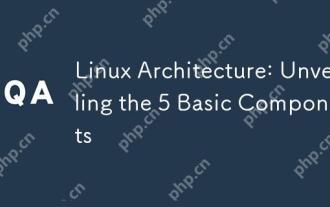
The five basic components of the Linux system are: 1. Kernel, 2. System library, 3. System utilities, 4. Graphical user interface, 5. Applications. The kernel manages hardware resources, the system library provides precompiled functions, system utilities are used for system management, the GUI provides visual interaction, and applications use these components to implement functions.
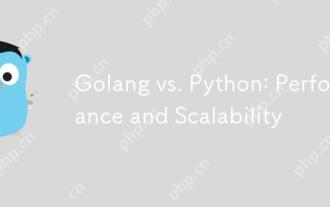
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
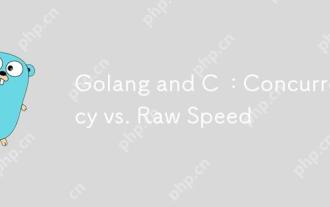
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
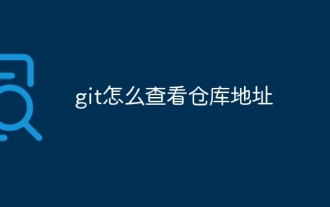
To view the Git repository address, perform the following steps: 1. Open the command line and navigate to the repository directory; 2. Run the "git remote -v" command; 3. View the repository name in the output and its corresponding address.
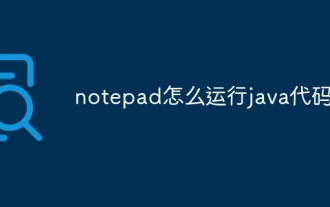
Although Notepad cannot run Java code directly, it can be achieved by using other tools: using the command line compiler (javac) to generate a bytecode file (filename.class). Use the Java interpreter (java) to interpret bytecode, execute the code, and output the result.
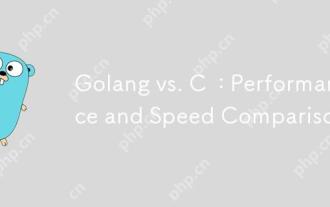
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
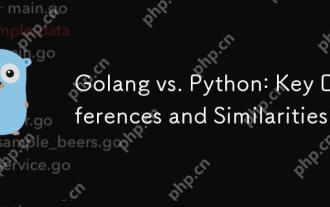
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
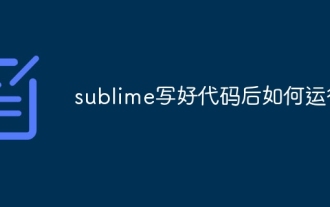
There are six ways to run code in Sublime: through hotkeys, menus, build systems, command lines, set default build systems, and custom build commands, and run individual files/projects by right-clicking on projects/files. The build system availability depends on the installation of Sublime Text.
