WeChat JSSDK upload pictures_javascript skills
Not long ago, WeChat released some interfaces, among which there is an uploadImage interface for uploading images, which is generally used in conjunction with the chooseImage interface. First call the chooseImage interface to let the user select one or more pictures. After the user completes the selection, WeChat will return the ID of the selected picture, and then pass the picture ID to the uploadImage interface to upload the picture.
A project I worked on recently happened to use JSSDK. Let’s sort out the things used.
First attach the link to the WeChat Developer Documentation: WeChat Developer Documentation
Mainly used:
Introduce JS files
Introduce the following JS file into the page that needs to call the JS interface (https is supported): http://res.wx.qq.com/open/js/jweixin-1.0.0.js
We need to get WeChat js-sdk parameters
/** * 获取access_token * * @param appid * 凭证 * @param appsecret * 密钥 * @return */ public static WxAccessToken getAccessToken() { WxAccessToken accessToken = null; String requestUrl = access_token_url.replace("APPID", Setting.getSetting("WX_PL_APP_ID")).replace( "APPSECRET", Setting.getSetting("APP_SECRET")); JSONObject jsonObject = httpRequest(requestUrl, "GET", null); // 如果请求成功 if (null != jsonObject) { try { accessToken = new WxAccessToken(); accessToken.setToken(jsonObject.getString("access_token")); accessToken.setExpiresIn(jsonObject.getInt("expires_in")); } catch (JSONException e) { accessToken = null; // 获取token失败 log.error("获取token失败 errcode:{} errmsg:{}", jsonObject.getInt("errcode"), jsonObject.getString("errmsg")); } } return accessToken; } public static String jsapiTicket = "https://api.weixin.qq.com/cgi-bin/ticket/getticket?access_token=ACCESS_TOKEN&type=jsapi"; /** * 获取JsTicket * * @param accessToken * accessToken * @return */ public static WxJsTicket getJsTicket(String accessToken) { WxJsTicket jsTicket = null; String url = jsapiTicket.replaceAll("ACCESS_TOKEN", accessToken); JSONObject jsonObject = httpRequest(url, "GET", null); // 如果请求成功 if (null != jsonObject) { try { jsTicket = new WxJsTicket(); jsTicket.setTicket(jsonObject.getString("ticket")); jsTicket.setExpiresIn(jsonObject.getInt("expires_in")); } catch (JSONException e) { jsTicket = null; // 获取token失败 log.error("获取jsapiTicket失败 errcode:{} errmsg:{}", jsonObject.getInt("errcode"), jsonObject.getString("errmsg")); } } return jsTicket; }
It should be noted that the number of calls to the interface is limited and needs to be controlled.
Page configuration
wx.config({ debug: false, // 开启调试模式,调用的所有api的返回值会在客户端alert出来,若要查看传入的参数,可以在pc端打开,参数信息会通过log打出,仅在pc端时才会打印。 appId: "$!{wxsign.get('appId')}", // 必填,公众号的唯一标识 timestamp: "$!{wxsign.get('timeStamp')}", // 必填,生成签名的时间戳 nonceStr: "$!{wxsign.get('nonceStr')}", // 必填,生成签名的随机串 signature: "$!{wxsign.get('signature')}",// 必填,签名,见附录1 jsApiList: ['checkJsApi', 'chooseImage', 'previewImage', 'uploadImage'] // 必填,需要使用的JS接口列表,所有JS接口列表见附录2 }); var images = { localId: [], serverId: [] };
Interface for taking pictures or selecting pictures from the mobile phone album
wx.chooseImage({ count: 1, // 默认9 sizeType: ['original', 'compressed'], // 可以指定是原图还是压缩图,默认二者都有 sourceType: ['album', 'camera'], // 可以指定来源是相册还是相机,默认二者都有 success: function (res) { var localIds = res.localIds; // 返回选定照片的本地ID列表,localId可以作为img标签的src属性显示图片 } });
Upload picture interface
wx.uploadImage({ localId: '', // 需要上传的图片的本地ID,由chooseImage接口获得 isShowProgressTips: 1, // 默认为1,显示进度提示 success: function (res) { var serverId = res.serverId; // 返回图片的服务器端ID } });
For the serverid returned by WeChat, we need to obtain the real image binary data through the WeChat API.
/** * 获取媒体文件 * * @param accessToken * 接口访问凭证 * @param media_id * 媒体文件id * */ public static String downloadMedia(String mediaId,HttpServletRequest request) { String requestUrl = "http://file.api.weixin.qq.com/cgi-bin/media/get?access_token=ACCESS_TOKEN&media_id=MEDIA_ID"; requestUrl = requestUrl.replace("ACCESS_TOKEN", WxTokenThread.accessToken.getToken()).replace( "MEDIA_ID", mediaId); HttpURLConnection conn = null; try { URL url = new URL(requestUrl); conn = (HttpURLConnection) url.openConnection(); conn.setDoInput(true); conn.setRequestMethod("GET"); conn.setConnectTimeout(30000); conn.setReadTimeout(30000); BufferedInputStream bis = new BufferedInputStream( conn.getInputStream()); ByteArrayOutputStream swapStream = new ByteArrayOutputStream(); byte[] buff = new byte[100]; int rc = 0; while ((rc = bis.read(buff, 0, 100)) > 0) { swapStream.write(buff, 0, rc); } byte[] filebyte = swapStream.toByteArray(); return PictureStore.getInstance().getImageServerUrl() + PictureStore.getInstance().store(filebyte); } catch (Exception e) { e.printStackTrace(); } finally { if(conn != null){ conn.disconnect(); } } return ""; }
Overall, it is relatively simple to implement this function, but I have never been exposed to the WeChat API before.
WeChat jssdk upload multiple pictures
The code is as follows:
jssdk
$('#filePicker').on('click', function () { wx.chooseImage({ success: function (res) { var localIds = res.localIds; syncUpload(localIds); } }); }); var syncUpload = function(localIds){ var localId = localIds.pop(); wx.uploadImage({ localId: localId, isShowProgressTips: 1, success: function (res) { var serverId = res.serverId; // 返回图片的服务器端ID //其他对serverId做处理的代码 if(localIds.length > 0){ syncUpload(localIds); } } }); };
This article shares with you the method of uploading images with WeChat JSSDK. I hope it will be helpful to you in your future work and study. Of course, there are more than just the above methods. There are many more. You are welcome to share your experience.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
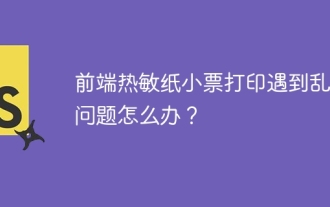
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
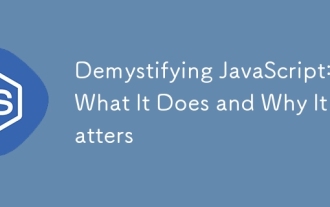
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
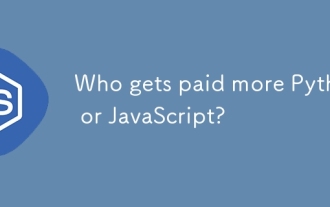
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
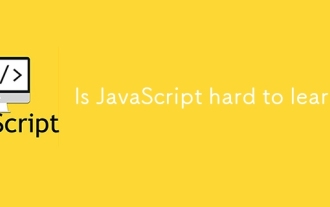
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
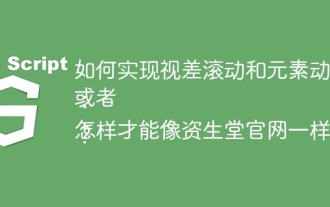
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
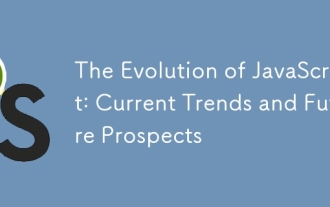
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
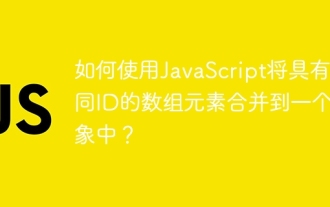
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
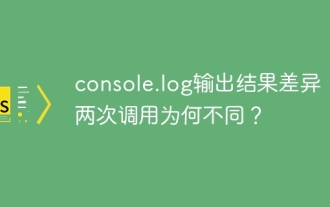
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
