Discuss string replacement operations in Golang
Golang is an efficient programming language, which is characterized by strong readability, simple code writing, and extremely high execution speed. As a Golang developer, we often encounter situations where we need to operate on strings, and Golang provides many convenient functions and methods to meet this need. One of the common needs is to replace the content in a string. In this article, we will discuss string replacement operations in Golang.
First, let’s take a look at the most basic string replacement operation. The strings package in Golang provides the Replace() function to perform string replacement. The Replace() function accepts three parameters: the source string, the string to be replaced, and the replaced string. The following is a sample code implementation:
package main import ( "fmt" "strings" ) func main() { str := "Hello,World!" newStr := strings.Replace(str, "World", "Golang", -1) fmt.Println(newStr) }
In the above example, we use the strings.Replace() function to replace the string "World" with "Golang". The third parameter of the function -1 means to replace all matching strings. If we want to replace only the first matching string, we can set the third parameter to 1:
newStr := strings.Replace(str, "World", "Golang", 1)
Next let's see how to implement a more flexible and complex string replacement.
Regular expression replacement
Regular expression is a powerful string matching tool. In Golang, the regexp package provides support for regular expressions. We can use regular expressions to achieve more flexible and complex string replacement operations. The following is a sample code that implements replacing numbers with letters:
package main import ( "fmt" "regexp" ) func main() { str := "123abc" re := regexp.MustCompile(`\d+`) newStr := re.ReplaceAllStringFunc(str, func(s string) string { r := []rune(s) return string(r[0] + 49) }) fmt.Println(newStr) }
In the above example, we use the ReplaceAllStringFunc() function provided by the regexp package to replace strings. The ReplaceAllStringFunc() function accepts two parameters: the source string and the callback function. The callback function accepts a parameter string and returns the replaced string. In this example, we use the regular expression \d
to match numbers, and then replace each number with the letter after it in the callback function, such as 0 is replaced with a, 1 is replaced with b, to And so on.
Custom replacement function
In the above example, we used regular expressions to match strings. But sometimes we don't want to use regular expressions, but need to write a custom replacement function to complete the replacement operation. The following is a sample code that converts uppercase letters to lowercase letters:
package main import ( "fmt" "unicode" ) func main() { str := "Hello,World!" newStr := ReplaceFunc(str, func(r rune) rune { if unicode.IsUpper(r) { return unicode.ToLower(r) } return r }) fmt.Println(newStr) } func ReplaceFunc(str string, f func(rune) rune) string { runes := []rune(str) for i, c := range runes { runes[i] = f(c) } return string(runes) }
In the above example, we wrote a custom replacement function ReplaceFunc(). The ReplaceFunc() function accepts two parameters: a string and a callback function. The callback function accepts a rune type parameter and returns the replaced rune type, and then uses this callback function to replace each character in the string.
Summary
In Golang, we can use the strings package, regexp package and custom replacement functions to implement flexible and complex string replacement operations. These functions and methods provide many practical functions to efficiently complete string replacement operations. Hope this article can be helpful to you.
The above is the detailed content of Discuss string replacement operations in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










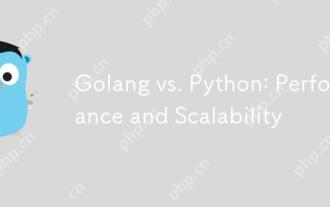
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
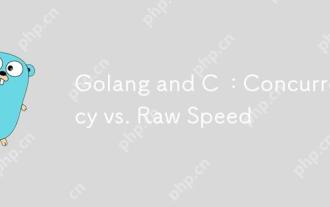
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
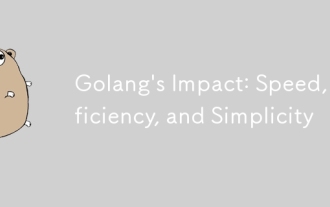
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
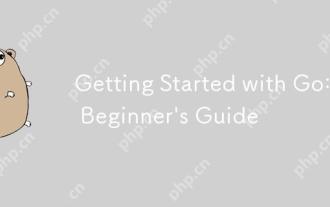
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
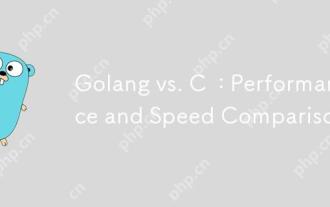
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
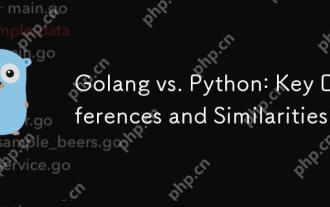
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
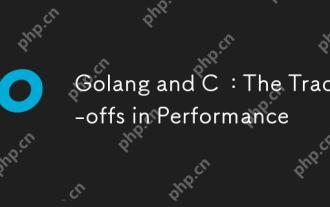
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
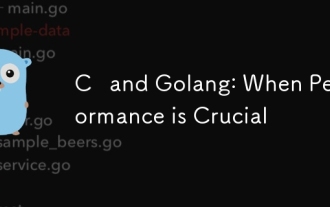
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
