What are laravel functions
Laravel functions include: 1. array_add(), which can add a given key-value pair to an array; 2. array_collapse(), which can collapse each array of the array into a single array; 3. array_dot (), which can convert a multi-dimensional array into a one-dimensional array; 4. array_except(), etc.
The operating environment of this tutorial: Windows 7 system, Laravel 6 version, DELL G3 computer.
laravel common functions
Array type function
1, array_add function
If the given The key does not exist in the array. The array_add function adds the given key-value pair to the array.
array_add($array, 'key', 'value');
2. array_collapse
Collapses each array of the array into a single array
array_collapse($array);
3. array_divide
The function returns two arrays, one containing the keys of the original array and the other containing the values of the original array
array_divide($array);
4. array_dot
Flatten the multi-dimensional array into a one-dimensional array, and use "dot" syntax to express the depth
array_dot($array);
5, array_except
Remove the given key-value pair from the array
array_except($array, array('key'));
6, array_first
Return the first element in the array that passes the true test
array_first($array, function($key, $value){}, $default);
7, array_flatten
Flatten the multi-dimensional array into one dimension
['Joe', 'PHP', 'Ruby']; array_flatten(['name' => 'Joe', 'languages' => ['PHP', 'Ruby']]);
8, array_forget
Use "dot" syntax to remove a given key-value pair from a deeply nested array
array_forget($array, 'foo'); array_forget($array, 'foo.bar');
9, array_get
Use "Dot" syntax retrieves a given value from a deeply nested array
array_get($array, 'foo', 'default'); array_get($array, 'foo.bar', 'default');
10. array_has
Use "dot" syntax to check whether a given item exists in the array
array_has($array, 'products.desk');
11, array_only
Return the given key-value pair from the array
array_only($array, array('key'));
12, array_pluck
Pull out a given key-value pair from the array
array_pluck($array, 'key');
13, array_pull
Removes and returns the given key-value pair from the array
array_pull($array, 'key');
14, array_set
Use "dot" syntax Write a value in a deeply nested array
array_set($array, 'key', 'value'); array_set($array, 'key.subkey', 'value');
15, array_sort
Sort the array by the result of the given closure
array_sort($array, function(){});
16, array_sort_recursive
Use the sort function to recursively sort the array
array_sort_recursive();
17, array_where
Use the given closure to filter the array
array_where();
18, head
Return the given array The first element
head($array);
19, last
Returns the last element of the given array
last($array);
Path function
1. app_path
Get the full path of the app folder
app_path();
2. base_path
Get the full path of the project root directory
base_path();
3.config_path
Get the full path of the application configuration directory
config_path();
4. database_path
Get the full path of the application database directory
database_path();
5.elixir
Get the Elixir file path plus the version number
elixir();
6, public_path
Get the full path of the public directory
public_path();
7, storage_path
Get storage The full path of the directory
storage_path();
String function
1, camel_case
Convert the given string into camel case naming
camel_case($value);
2. class_basename
Returns the class name without namespace
class_basename($class); class_basename($object);
3. e
Run htmlentities on the given string
e('<html>');
4 , starts_with
Determine whether the beginning of the string is the given content
starts_with('Foo bar.', 'Foo');
5, ends_with
Determine whether the end of the given string is the specified content
ends_with('Foo bar.', 'bar.');
6. snake_case
Convert the given string into a snake name
snake_case('fooBar');
7. str_limit
Limit the number of characters in the string
str_limit();
8 , str_contains
Determine whether the given string contains the specified content
str_contains('Hello foo bar.', 'foo');
9, str_finish
Add the given content to the end of the string, foo/bar/
str_finish('foo/bar', '/');
10. str_is
Determine whether the given string matches the given format
str_is('foo*', 'foobar');
11. str_plural
Convert the string into plural form
str_plural('car');
12, str_random
Generate a random string of a given length
str_random(25);
13, str_singular
Convert the string into singular form. This function currently only supports English
str_singular('cars');
14, str_slug
Generate a URL-friendly "slug" from a given string
str_slug("Laravel 5 Framework", "-");
15, studly_case
Convert the given string to "capitalization": FooBar
studly_case('foo_bar');
16, trans
Translate the given statement according to your localization file
trans('foo.bar');
17 , trans_choice
Translate the given statement according to the suffix change
trans_choice('foo.bar', $count);
URLs and Links function
1、action
generated to Define the controller behavior URL
action('FooController@method', $parameters);
2, asset
Generate the resource file URL based on the current request protocol (HTTP or HTTPS)
asset('img/photo.jpg', $title, $attributes);
3, secure_asset
Generate resource file URL according to HTTPS
secure_asset('img/photo.jpg', $title, $attributes);
4, route
Generate URL of given route name
route($route, $parameters, $absolute = true);
5, url
Generate URL of given path Full URL
url('path', $parameters = array(), $secure = null);
Miscellaneous function
1. auth()->user()
Returns an authenticator instance. You can use this instead of the Auth facade
auth()->user();
2, back
Generate a redirect response to return the user to the previous location
back();
3, bcrypt
Hash the given value using Bcrypt. You can use this instead of Hash facade
bcrypt('my-secret-password');
4, collect
Generate a collection instance from a given project
collect(['taylor', 'abigail']);
5, config
Get the setting value of the setting option
config('app.timezone', $default);
6、
产生包含 CSRF 令牌内容的 HTML 表单隐藏字段
{!! csrf_field() !!}
7、csrf_token
取得当前 CSRF 令牌的内容
$token = csrf_token();
8、dd
输出给定变量并结束脚本运行
dd($value);
9、env
取得环境变量值或返回默认值
$env = env('APP_ENV'); $env = env('APP_ENV', 'production');
10、
配送给定事件到所属的侦听器
event(new UserRegistered($user));
11、
根据给定类、名称以及总数产生模型工厂建构器
$user = factory(App\User::class)->make();
12、
产生拟造 HTTP 表单动作内容的 HTML 表单隐藏字段
{!! method_field('delete') !!}
13、old
取得快闪到 session 的旧有输入数值
$value = old('value'); $value = old('value', 'default');
14、redirect
返回重定向器实例以进行 重定向
return redirect('/home');
15、request
取得目前的请求实例或输入的项目
$value = request('key', $default = null)
16、response
创建一个回应实例或获取一个回应工厂实例
return response('Hello World', 200, $headers);
17、session
可被用于取得或设置单一 session 内容
$value = session('key');
18、
在没有传递参数时,将返回 session 实例
$value = session()->get('key'); session()->put('key', $value);
19、
返回给定数值
value(function(){ return 'bar'; });
20、view
取得视图 实例
return view('auth.login');
21、
返回给定的数值
$value = with(new Foo)->work();
【相关推荐:laravel视频教程】
The above is the detailed content of What are laravel functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










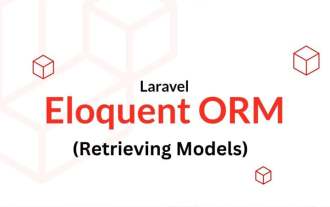
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
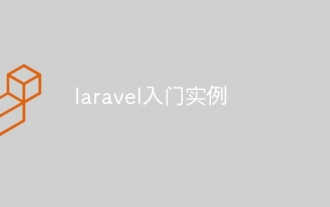
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
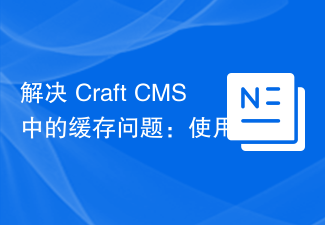
When developing websites using CraftCMS, you often encounter resource file caching problems, especially when you frequently update CSS and JavaScript files, old versions of files may still be cached by the browser, causing users to not see the latest changes in time. This problem not only affects the user experience, but also increases the difficulty of development and debugging. Recently, I encountered similar troubles in my project, and after some exploration, I found the plugin wiejeben/craft-laravel-mix, which perfectly solved my caching problem.
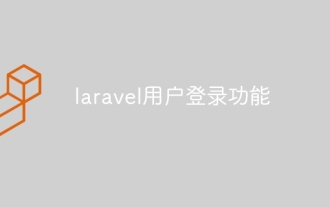
Laravel provides a comprehensive Auth framework for implementing user login functions, including: Defining user models (Eloquent model), creating login forms (Blade template engine), writing login controllers (inheriting Auth\LoginController), verifying login requests (Auth::attempt) Redirecting after login is successful (redirect) considering security factors: hash passwords, anti-CSRF protection, rate limiting and security headers. In addition, the Auth framework also provides functions such as resetting passwords, registering and verifying emails. For details, please refer to the Laravel documentation: https://laravel.com/doc
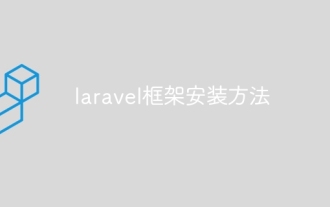
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.
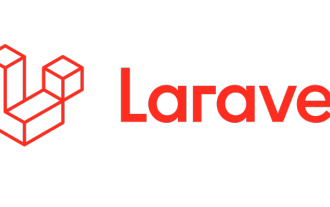
Efficiently process 7 million records and create interactive maps with geospatial technology. This article explores how to efficiently process over 7 million records using Laravel and MySQL and convert them into interactive map visualizations. Initial challenge project requirements: Extract valuable insights using 7 million records in MySQL database. Many people first consider programming languages, but ignore the database itself: Can it meet the needs? Is data migration or structural adjustment required? Can MySQL withstand such a large data load? Preliminary analysis: Key filters and properties need to be identified. After analysis, it was found that only a few attributes were related to the solution. We verified the feasibility of the filter and set some restrictions to optimize the search. Map search based on city

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.
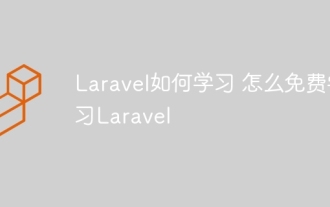
Want to learn the Laravel framework, but suffer from no resources or economic pressure? This article provides you with free learning of Laravel, teaching you how to use resources such as online platforms, documents and community forums to lay a solid foundation for your PHP development journey from getting started to master.
