What is laravel queue
In laravel, a queue is a linear table with restricted operations. It only allows deletion operations at the front end of the table (queue head), and insertion operations at the back end of the table (queue tail); Through queues, developers can postpone the processing of time-consuming tasks, which can greatly improve the response speed of web requests.
The operating environment of this tutorial: Windows 7 system, Laravel 6 version, Dell G3 computer.
The use of queues in laravel
1. What is a queue
Queue It is a linear table with restricted operations. The special feature is that it only allows deletion operations at the front end of the table and insertion operations at the back end of the table. The end that performs the insertion operation is called the tail of the queue, and the end that performs the deletion operation is called the head of the queue.
With queues, you can postpone the processing of time-consuming tasks (such as sending emails) until later. Delaying these time-consuming tasks can greatly improve web request response speed.
2. Advantages
Decoupling: The message queue can decouple the system and improve the response speed. Functions are aggregated inward and open to the outside;
Asynchronous: Message queue can strip off the asynchronous functions of the system, reduce functional coupling, and improve development efficiency;
Peak clipping: The message queue can clip peak and flow to ensure stable operation of downstream consumers;
3. Configuration
The queue configuration file is stored in config/queue.php. In this file, you can find the connection configuration for each queue driver included in the framework, which includes database, Beanstalkd, Amazon SQS, Redis, and a synchronization driver (sync - for local use).
Redis is used as the driver here, and Redis and related extensions need to be installed.
4. Task
We need to put something into the queue, we can call it a task. Creating tasks in the Laravel framework provides us with the following commands:
php artisan make:job TestJob
TestJob.php
namespace App\Jobs;use Illuminate\Bus\Queueable;use Illuminate\Contracts\Queue\ShouldBeUnique;use Illuminate\Contracts\Queue\ShouldQueue;use Illuminate\Foundation\Bus\Dispatchable;use Illuminate\Queue\InteractsWithQueue;use Illuminate\Queue\SerializesModels;use Illuminate\Support\Facades\DB;use Symfony\Polyfill\Intl\Idn\Info;class TestJob implements ShouldQueue{ use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; /** * Create a new job instance. * * @return void */ public function __construct() { // } /** * Execute the job. * * @return void */ public function handle() {// \Log::info('hhh'); DB::connection('test') ->table('master') ->insert([ 'name'=>'小白', 'email'=>'123@qq.com' ]); }}
5. Distribution
Once you write a task class, you can dispatch it using the dispatch method of the task itself. The parameters passed to the dispatch method will be passed to the task's constructor.
onQueue: Specified queue;
onConnection: Specified connection;
delay: Delay queue;
dispatchNow: Synchronous scheduling;
#在路由中简单调用 Route::get('queue',function(){ \App\Jobs\TestJob::dispatch(); // \App\Jobs\TestJob::dispatch()->onQueue('qq'); });
Run two This route can be seen to generate a queue named qq. Later we will consume the queue
6. Queue consumption
Laravel has a queue processor to process newly pushed tasks into the queue. Start the queue processor with the Artisan command queue:work. It should be noted that once the queue:work command is started, it will keep running until it is manually stopped or you close your terminal:
php artisan queue:work
php artisan queue: work --once Add parameters and consume the specified queue
#消费qq队列 php artisan queue:work --queue=qq
You can see that two new pieces of data have been added to the database, and the data in redis has been consumed
We execute routing again
7, event queue
Queue is usually used to handle delayed tasks, and events are processed by business logic. Event triggers in Laravel are distributed to queues for asynchronous business processing, so that you can quickly respond without having to wait for the execution results in real time before giving prompt messages to users.
If we need to store the business in the event into the queue, we do not need to re-distribute the queue. We can directly implement the Illuminate\Contracts\Queue\ShouldQueue interface in the corresponding listener.
Create events and listeners
php artisan make:event TestEvent php artisan make:listener TestListener
Register in app\providers\EventServiceProvider.php
TestListener.php
namespace App\Listeners; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Queue\InteractsWithQueue; use Illuminate\Support\Facades\DB; class TestListener implements ShouldQueue { /** * Create the event listener. * * @return void */ public function __construct() { // } /** * Handle the event. * * @param object $event * @return void */ public function handle() { DB::connection('test') ->table('master') ->insert([ 'name'=>'小黑', 'email'=>'234@qq.com' ]); } }
Modify routing
Route::get('queue',function(){ //\App\Jobs\TestJob::dispatch(); //指定队列名称 //\App\Jobs\TestJob::dispatch()->onQueue('qq'); return event(new \App\Events\TestEvent()); });
Run routing
Consumption queue
php artisan queue:work
【Related recommendations: laravel video tutorial】
The above is the detailed content of What is laravel queue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
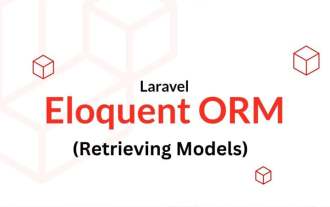
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
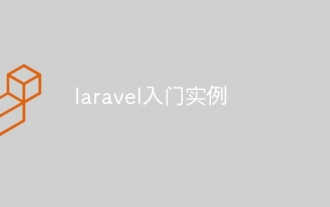
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
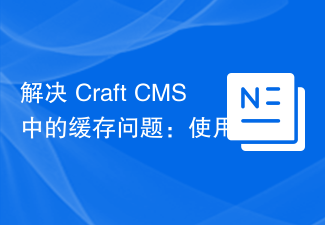
When developing websites using CraftCMS, you often encounter resource file caching problems, especially when you frequently update CSS and JavaScript files, old versions of files may still be cached by the browser, causing users to not see the latest changes in time. This problem not only affects the user experience, but also increases the difficulty of development and debugging. Recently, I encountered similar troubles in my project, and after some exploration, I found the plugin wiejeben/craft-laravel-mix, which perfectly solved my caching problem.
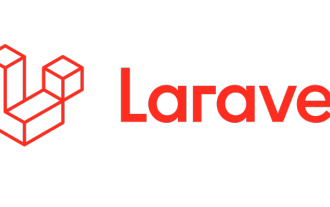
Efficiently process 7 million records and create interactive maps with geospatial technology. This article explores how to efficiently process over 7 million records using Laravel and MySQL and convert them into interactive map visualizations. Initial challenge project requirements: Extract valuable insights using 7 million records in MySQL database. Many people first consider programming languages, but ignore the database itself: Can it meet the needs? Is data migration or structural adjustment required? Can MySQL withstand such a large data load? Preliminary analysis: Key filters and properties need to be identified. After analysis, it was found that only a few attributes were related to the solution. We verified the feasibility of the filter and set some restrictions to optimize the search. Map search based on city
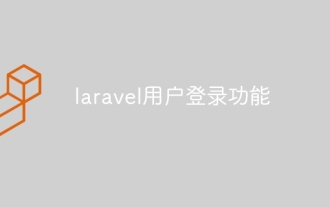
Laravel provides a comprehensive Auth framework for implementing user login functions, including: Defining user models (Eloquent model), creating login forms (Blade template engine), writing login controllers (inheriting Auth\LoginController), verifying login requests (Auth::attempt) Redirecting after login is successful (redirect) considering security factors: hash passwords, anti-CSRF protection, rate limiting and security headers. In addition, the Auth framework also provides functions such as resetting passwords, registering and verifying emails. For details, please refer to the Laravel documentation: https://laravel.com/doc

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.
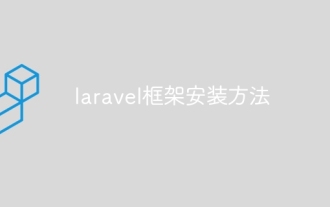
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.
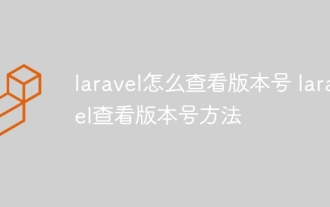
The Laravel framework has built-in methods to easily view its version number to meet the different needs of developers. This article will explore these methods, including using the Composer command line tool, accessing .env files, or obtaining version information through PHP code. These methods are essential for maintaining and managing versioning of Laravel applications.
