How to break out of a loop in javascript
How to jump out of a loop in JavaScript: 1. Use the continue statement to terminate the current loop and immediately enter the next loop; 2. Use the break statement to exit the entire loop, the code following the break statement, and the following loops None will be executed.
The operating environment of this tutorial: windows7 system, javascript version 1.8.5, Dell G3 computer.
Javascript method 1 to jump out of the loop: use the continue statement
The continue statement is used to terminate the current loop and immediately enter the next loop. The basic syntax of the
continue statement is as follows:
continue;
The execution of the continue statement usually requires setting a certain condition. When the condition is met, the continue statement is executed.
Example: Application of continue statement
<script> var sum = 0; var str = "1~20之间的偶数有:"; //把1~20之间的偶数进行累加 for(var i = 1; i < 20; i++){ //判断i是否为偶数,如果模不等于0,为奇数,结束当前循环,进入下一次循环 if(i % 2 != 0) continue; sum += i; //如果执行continue语句,循环体内的该行以及后面的代码都不会被执行 str +=i + " "; } str += "\n这些偶数的和为:" + sum; alert(str); </script>
The above code uses i%2!=0 as the condition for the execution of the continue statement. If the value of the conditional expression is true, That is, when i is an odd number, the continue statement is executed to terminate the current loop. At this time, the code following the continue statement will not be executed, so the odd numbers will not be accumulated. It can be seen that by using the continue statement, you can ensure that only even numbers are accumulated.
The result of the dialog box that pops up after the above code is run in the Chrome browser is as shown below.
Javascript method two to jump out of the loop: use the break statement
The role of the break statement used alone There are two aspects:
One is to exit the switch in the switch statement;
The second is to exit the entire loop in the loop statement.
In actual application, break can also be followed by a label. At this time, the function of the break statement is to jump to the end of the statement block identified by the label. When you need to jump from an inner loop to the end of an outer loop, you need to use a labeled break statement. The basic syntax of the
break statement is as follows:
break; //单独使用,在循环语句中用于退出整个循环 break lablename; //带有标签,在多层循环语句中用于从内层循环跳转到 lablename 外层循环的结束处
The break statement is the same as the continue statement. A certain condition also needs to be set for execution. When the condition is met, the break statement is executed.
Example: Application of break statement
<script> var sum = 0; var str = "1~20之间的被累加的偶数有:"; //把1~20之间的偶数进行累加 for(var i = 2; i < 20;i += 2){ if(sum > 60) break; //执行break语句后,整个循环立刻停止结束执行 sum += i; str += i + " "; } str += "\n这些偶数的和为:" + sum; alert(str); </script>
The above code uses sum>60 as the condition for the execution of the break statement. If the value of the conditional expression is true, the break statement is executed. Exit the entire loop. At this time, the code following the break statement and the subsequent loop will not be executed.
The result of the dialog box that pops up after the above code is run in the Chrome browser is as shown below.
[Recommended learning: javascript advanced tutorial]
The above is the detailed content of How to break out of a loop in javascript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










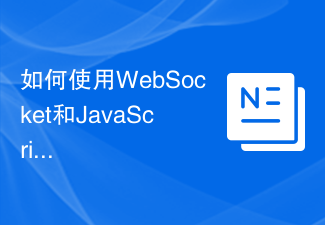
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
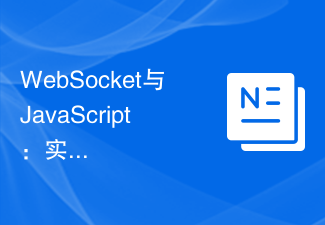
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
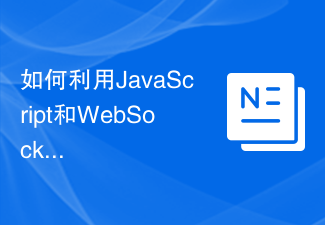
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
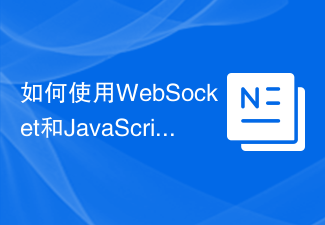
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
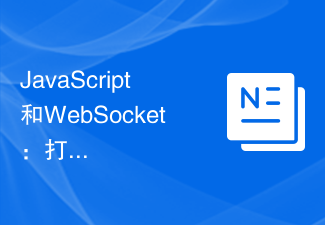
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
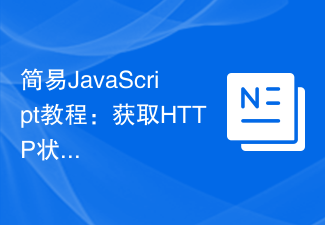
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
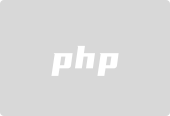
Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
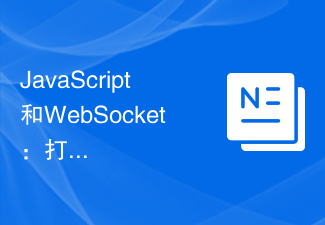
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
