


Detailed explanation of JavaScript function objects_javascript skills
Function
A function is an event-driven or reusable block of code that executes when it is called.
function One(leve , leve){ //code return leve+leve }
Note:
Formal parameters do not need to be typed;
The return statement is optional, and a function without a return statement returns undefined;
Local variables and global variables
Declaration within the function: local variable
Declaration outside the function: global variable
When assigning a value to a new variable name, var is not used: the variable will become a new global variable
Functions can be used as values
Form 1:
function init(){ alert("One") } window.onload = init;
Format:
window.onload = function(){ alert("One"); }
Note: The above two methods can make the browser prompt: One.
Object
Everything in JavaScript is an object: strings, numbers, arrays, functions, and in addition, JavaScript allows custom objects.
Object reference
When assigning an object to a variable, the variable will contain a reference to the object, not the object itself.
When calling a function and passing in an object, only the object reference is actually passed (copy a copy of the reference, pass it to the formal parameter, and point to the object, that is, the two references point to the same object)
Create object
var dog = { name : "myDog", weight : , bark :function(){ alert("woof!"); } } dog.bark();
Note: There must be "," after each attribute (except the last one).
Constructor constructs object
function Dog(name,weight){ this.name = name; this.weight = weight; this.bark = function(){ if(this.weight > ){ alert(this.name + "Woof!"); }else{ alert(this.name + "Yip!"); } };//这里也不能忘了分号 } var myDog = new Dog("hello",""); myDog.bark();
PS:
1. What is a constructor
Constructor is a special method. It is mainly used to initialize the object when creating the object, that is, to assign initial values to the object member variables. It is always used together with the new operator in the statement to create the object.
This is the explanation I gave after consulting relevant information. The explanation is very book-like but the meaning is still very clear. Please see a small example below:
The code is as follows:
var request = new XMLHttpRequest();
This expression is often used when we create request objects when using AJAX technology. Then we can clearly see that "new XMLHttpRequest();" is a standard constructor! We declare a "request" object in "var" and use the constructor "new XMLHttpRequest();" to initialize this " request" object and assign it an initial value. So we can know: "The 'function' used with the 'new' operator to create and initialize the object is the constructor".
For example, our common way to declare an array is the standard constructor: var array = new Array();
2. What is an instantiated object
The code is as follows:
var request = new XMLHttpRequest();
In object-oriented programming, the process of creating objects using classes is usually called instantiation.
I highlighted the key points of the explanation in red and blue above. To put it bluntly, instantiating an object is the process of creating an object!
So what is a "class"? According to the literal understanding, we can understand it as a "type". For example, "cake" is a category of desserts, that is, a type; then cheesecake is a specific individual of the category of cake in desserts, that is, an object.
We know that in programming language, "class" is abstract. We have no way to operate it or use its methods and properties. Only by instantiating this class into an object can we call a series of it. methods and properties. In fact, this is also easy to understand. In life, we have no way to see or capture abstract things, so naturally we have no way to use some of its functions. We can only concrete the abstract things into each individual, individual or actual situation. Only with the object can we clearly understand or recognize it; the same is true for programming. Therefore, instantiating an object is a process from abstract to concrete, and this process is called instantiation.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










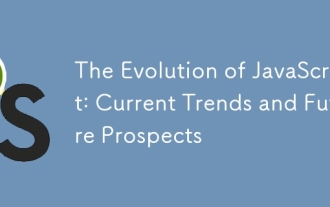
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
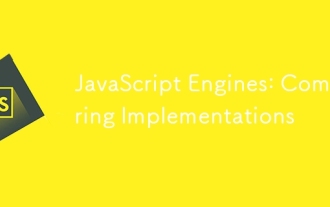
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
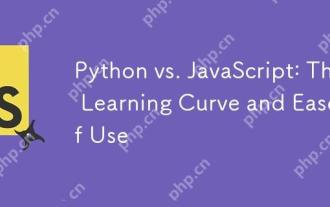
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
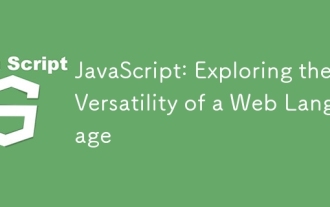
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
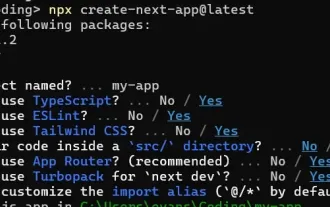
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
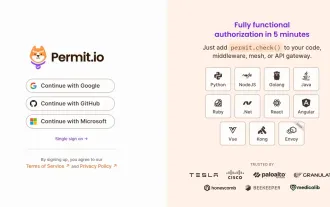
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
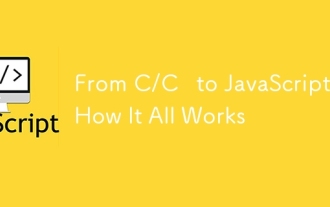
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
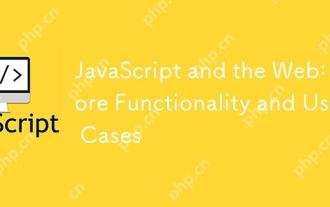
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
