


In-depth understanding of inheritance and prototype chain in JavaScript
This article will explain the inheritance and prototype chain in JavaScript in detail, with a comprehensive analysis of the text and code, which has certain reference value. Friends who need it can refer to it. I hope it can help you.
Almost everything in Javascript is an object. Each object has an internal property that is linked to other objects, which we call prototype. The prototype object itself also has its own prototype object, and so on. At this time, the prototype chain comes out. If you trace the prototype chain, you will eventually reach the kernel Object whose prototype is null, which is the end of the prototype chain.
What is the role of the prototype chain? When we access a property that the object does not own, Javascript searches the prototype chain until it finds the property or the end of the prototype chain. This behavior ensures that we can create "classes" and implement inheritance.
If you don’t understand it yet, it doesn’t matter, let us understand it in practice. Now look at the simplest example of Javascript: a "class" created in the method object
function Animal() {} var animal = new Animal();
There are two ways for us to add properties to this Animal class: setting the properties of the instance or adding the Animal prototype.
function Animal(name) { // Instance properties can be set on each instance of the class this.name = name; } // Prototype properties are shared across all instances of the class. However, they can still be overwritten on a per-instance basis with the `this` keyword. Animal.prototype.speak = function() { console.log("My name is " + this.name); }; var animal = new Animal('Monty'); animal.speak(); // My name is Monty
The structure of the Animal object becomes clear when looking at the console. We can see that the name attribute belongs to the object itself, while speak belongs to the Animal prototype.
Now please take a look at how we extend the Animal class to create a Cat class:
function Cat(name) { Animal.call(this, name); } Cat.prototype = new Animal(); var cat = new Cat('Monty'); cat.speak(); // My name is Monty
What we have done is to set the prototype of Cat to an Animal instance, so Cat will inherit all the properties of Animal . Similarly, we use Animal.call to inherit the constructor of Animal. call is a special function that allows us to call a function and specify the value of this in the function. So when this.name is in Animal's constructor, Cat's name is set, not Animal's.
Let's take a look at the Cat object:
As we expected, the Cat object has its own name instance attribute. When we look at the object's prototype we see that it also inherits the name instance property of Animal, as does the speak prototype property. This is what the prototype chain looks like. When cat.name is accessed, JavaScript looks for the name instance property and does not search further down the prototype chain. Regardless, when we access cat.speak, JavaScript has to search further down the prototype chain until it finds the speak property inherited from Animal.
The above is the detailed content of In-depth understanding of inheritance and prototype chain in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










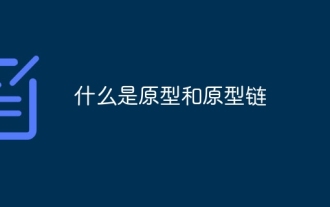
Prototype, an object in js, is used to define the properties and methods of other objects. Each constructor has a prototype attribute. This attribute is a pointer pointing to a prototype object. When a new object is created, the new object will be The prototype attribute of its constructor inherits properties and methods. Prototype chain, when trying to access the properties of an object, js will first check whether the object has this property. If not, then js will turn to the prototype of the object. If the prototype object does not have this property, it will continue to look for the prototype of the prototype.

Scope chain and prototype chain are two important concepts in JavaScript, corresponding to the two core features of scope and inheritance respectively: 1. Scope chain is a mechanism used to manage variable access and scope in JavaScript. It is formed by It is determined by the execution context and lexical scope in which the function is created; 2. The prototype chain is a mechanism for implementing inheritance in JavaScript. Based on the prototype relationship between objects, when accessing the properties or methods of an object, if the object itself does not Definition, will be searched up along the prototype chain.

The difference between prototype and prototype chain is: 1. Prototype is an attribute that each object has, including some shared attributes and methods, which is used to realize the sharing and inheritance of attributes and methods between objects, while prototype chain is a The inheritance mechanism is implemented through the prototype relationship between objects, which defines the inheritance relationship between objects so that objects can share the properties and methods of the prototype object; 2. The function of the prototype is to define the shared properties and methods of the object, so that multiple Objects can share the properties and methods of the same prototype object, and the function of the prototype chain is to realize the inheritance relationship between objects, etc.
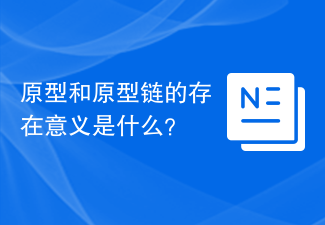
The reason why prototypes and prototype chains exist is to implement inheritance and sharing of object properties in the JavaScript language. In JavaScript, everything is an object, including functions. Every object has a property called a prototype that points to another object, which is called a prototype object. Objects can inherit properties and methods from prototype objects. The benefit of implementing shared properties and methods through prototypes is memory savings. Consider an object A, which has some properties and methods, then create object B and make
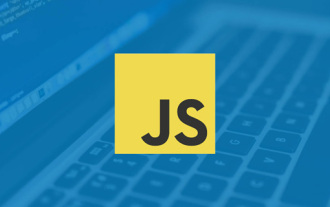
Prototype chain, simply understood is a chain composed of prototypes. When accessing an attribute of an object, it will first search on the attribute of the object itself. If it is not found, it will search on its __proto__ implicit prototype, that is, the prototype of its constructor. If it has not been found yet, It will then search in the __proto__ of the prototype of the constructor. In this way, searching upward layer by layer will form a chain structure, which is called a prototype chain.
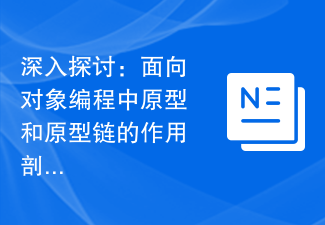
In-depth analysis: The role of prototype and prototype chain in object-oriented programming requires specific code examples. In object-oriented programming (OOP), prototype (Prototype) and prototype chain (PrototypeChain) are important concepts. They provide an object-based code reuse mechanism and play a key role in languages such as Javascript. In this article, we'll take a deep dive into the concepts of prototypes and prototype chains, explore their role in OOP, and illustrate with concrete code examples
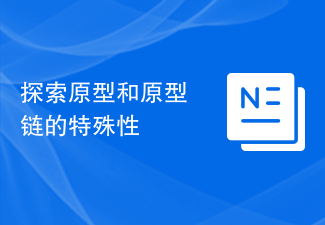
Exploring the unique features of prototype and prototype chain In JavaScript, prototype and prototype chain are very important concepts. Understanding the unique features of prototypes and prototype chains can help us better understand inheritance and object creation in JavaScript. A prototype is a property owned by every object in JavaScript that points to another object and is used to share properties and methods. Every JavaScript object has a prototype
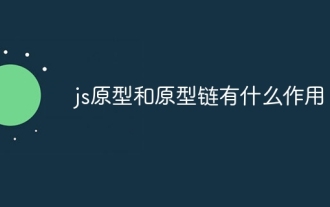
The function of js prototype and prototype chain is to realize the inheritance of objects, save memory space, and improve the performance and maintainability of the code. Detailed introduction: 1. Implement the inheritance of objects. The prototype and prototype chain allow you to create an object and inherit the properties and methods of another object. When you create a new object, you can point its prototype to another object, so that the new object The object can access the properties and methods on the prototype object; 2. Save memory and improve performance. In JavaScript, each object has a prototype. Through the prototype chain, objects can share prototypes, etc.
