


Detailed explanation of steps to implement WeChat public account menu editor with Vue.js (Part 1)
This time I will bring you Vue.js to implement the WeChat public account menuEditorDetailed steps (Part 1), Vue.js to implement the WeChat public account menu editorNotes What are they? Here are actual cases. Let’s take a look.
I have been studying Vue.js for a while, so I want to try to make a menu editor like the one on the WeChat platform. I will share it here
Check the project github for the specific style code
Create a vue instance
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title></title> <script src="https://cdn.bootcss.com/vue/2.5.9/vue.js"></script> </head> <body> <p class="content" style="width:900px;margin:0 auto;"> <!-- vue实例挂载的DOM元素 --> <p id="app-menu"> <!-- 菜单预览界面 --> <p class="weixin-preview"></p> <!-- 菜单编辑界面 --> <p class="weixin-menu-detail"></p> </p> </p> <script> var app = new Vue({ el: '#app-menu',//挂载到对应的DOM元素 data: { weixinTitle: 'Vue.js公众号菜单', //菜单对象 menu: { "button": [ { "name": "主菜单1", "sub_button": [] }, { "name": "主菜单2", "sub_button": [] }, { "name": "主菜单3", "sub_button": [ { "name": "子菜单1" }] }] }, selectedMenuIndex:'',//当前选中菜单索引 selectedSubMenuIndex:'',//当前选中子菜单索引 }, methods: { } }) </script> </body> </html>
Render the menu data to On the template
Here v-if and v-for are used to render data to the template. There will be up to 3 main menus and each main menu can have up to 5 submenus.
<p class="weixin-preview"> <p class="weixin-hd"> <p class="weixin-title">{{weixinTitle}}</p> </p> <p class="weixin-bd"> <ul class="weixin-menu"> <!-- 这里使用v-for开始循环主菜单 --> <li v-for="(btn,i) in menu.button" class="menu-item"> <p class="menu-item-title"> <span>{{ btn.name }}</span> </p> <ul class="weixin-sub-menu"> <!-- 这里使用v-for开始循环主菜单下的子菜单 --> <li v-for="(sub,i2) in btn.sub_button" class="menu-sub-item"> <p class="menu-item-title"> <span>{{sub.name}}</span> </p> </li> <!-- 这里使用v-if 判断子菜单小于5个,则添加按钮来添加子菜单 --> <li v-if="btn.sub_button.length<5" class="menu-sub-item"> <p class="menu-item-title"> <i class="icon14_menu_add"></i> </p> </li> </ul> </li> <!-- 这里使用v-if 判断主菜单小于3个,则添加按钮来添加主菜单 --> <li class="menu-item" v-if="menu.button.length<3"> <i class="icon14_menu_add"></i></li> </ul> </p> </p>
Add a method to the vue instance
In the vue instance give Add our custom method to the methods object
methods: { //选中主菜单 selectedMenu:function (i) { this.selectedSubMenuIndex = '' this.selectedMenuIndex = i }, //选中子菜单 selectedSubMenu:function (i) { this.selectedSubMenuIndex = i }, //选中菜单级别 selectedMenuLevel: function () { if (this.selectedMenuIndex !== '' && this.selectedSubMenuIndex === '') { //主菜单 return 1; } else if (this.selectedMenuIndex !== '' && this.selectedSubMenuIndex !== '') { //子菜单 return 2; } else { //未选中任何菜单 return 0; } }, //添加菜单 //参数level为菜单级别,1为主菜单、2为子菜单 addMenu:function (level) { if (level == 1 && this.menu.button.length < 3) { this.menu.button.push({"name": "菜单名称", "sub_button": [] }) this.selectedMenuIndex = this.menu.button.length - 1 this.selectedSubMenuIndex = '' } if (level == 2 && this.menu.button[this.selectedMenuIndex].sub_button.length < 5) { this.menu.button[this.selectedMenuIndex].sub_button.push({ "name": "子菜单名称" }) this.selectedSubMenuIndex = this.menu.button[this.selectedMenuIndex].sub_button.length - 1 } } }
Bind the method to the menu
When the menu is clicked, the selectedMenu method is triggered, and when the add button is clicked, the selectedMenu method is triggered. Add addMenu method. Use v-on to listen to the event , its abbreviation is @
to listen to the click event @click. In order to prevent the submenu click event from bubbling up the main menu, use the .stop event modifier To prevent bubbling @click.stop
Use v-bind:class to add the class when the switching menu is selected. :class is the abbreviation
<ul class="weixin-menu" id="weixin-menu" > <!-- 判断如果selectedMenuIndex是当前点击的主菜单索引则添加current样式 --> <li v-for="(btn,i) in menu.button" class="menu-item" :class="{current:selectedMenuIndex===i&&selectedMenuLevel()==1}" @click="selectedMenu(i)"> <p class="menu-item-title"> <span>{{ btn.name }}</span> </p> <!-- v-show来切换是否显示 这里如果选中了主菜单则子菜单弹出 --> <ul class="weixin-sub-menu" v-show="selectedMenuIndex===i"> <li v-for="(sub,i2) in btn.sub_button" class="menu-sub-item" :class="{current:selectedSubMenuIndex===i2&&selectedMenuLevel()==2}" @click.stop="selectedSubMenu(i2)"> <p class="menu-item-title"> <span>{{sub.name}}</span> </p> </li> <li v-if="btn.sub_button.length<5" class="menu-sub-item" @click.stop="addMenu(2)"> <p class="menu-item-title"> <i class="icon14_menu_add"></i> </p> </li> </ul> </li> <li class="menu-item" v-if="menu.button.length<3" @click="addMenu(1)"> <i class="icon14_menu_add"></i> </li> </ul>
I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related articles on the PHP Chinese website!
Recommended reading:
JS calculation of pi to 100 decimal places implementation steps detailed explanation
The above is the detailed content of Detailed explanation of steps to implement WeChat public account menu editor with Vue.js (Part 1). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










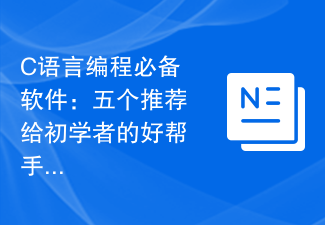
C language is a basic and important programming language. For beginners, it is very important to choose appropriate programming software. There are many different C programming software options on the market, but for beginners, it can be a bit confusing to choose which one is right for you. This article will recommend five C language programming software to beginners to help them get started quickly and improve their programming skills. Dev-C++Dev-C++ is a free and open source integrated development environment (IDE), especially suitable for beginners. It is simple and easy to use, integrating editor,
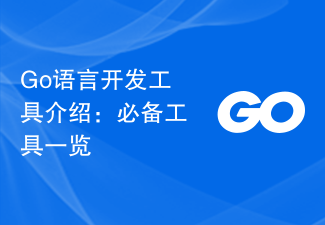
Title: Introduction to Go language development tools: List of essential tools In the development process of Go language, using appropriate development tools can improve development efficiency and code quality. This article will introduce several essential tools commonly used in Go language development, and attach specific code examples to allow readers to understand their usage and functions more intuitively. 1.VisualStudioCodeVisualStudioCode is a lightweight and powerful cross-platform development tool with rich plug-ins and functions.
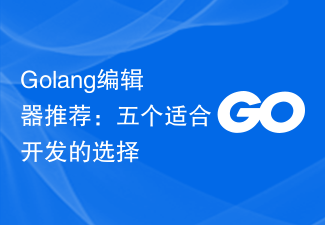
With the popularity and popularity of Golang, more and more developers are beginning to use this programming language. However, like other popular programming languages, Golang development requires choosing a suitable editor to improve development efficiency. In this article, we will introduce five editors suitable for Golang development. VisualStudioCodeVisualStudioCode (VSCode for short) is a free cross-platform editor developed by Microsoft. It is based on Elect
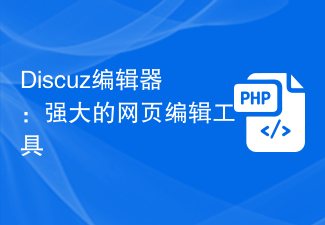
Discuz Editor: A powerful web page editing tool that requires specific code examples. With the development of the Internet, website construction and content editing have become more and more important. As a common web page editing tool, Discuz editor plays an important role in website construction. It not only provides a wealth of functions and tools, but also helps users edit and publish content more conveniently. In this article, we will introduce the features and usage of the Discuz editor, and provide some specific code examples to help readers better understand and use
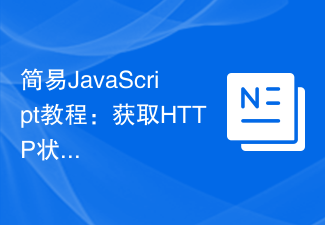
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
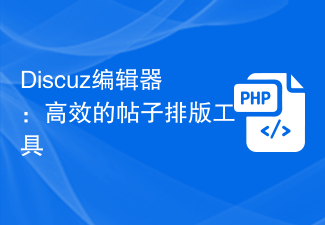
Discuz Editor: An efficient post layout tool. With the development of the Internet, online forums have become an important platform for people to communicate and share information. In the forum, users can not only express their opinions and ideas, but also discuss and interact with others. When publishing a post, a clear and beautiful format can often attract more readers and convey more accurate information. In order to facilitate users to quickly type and edit posts, the Discuz editor came into being and became an efficient post typesetting tool. Discu
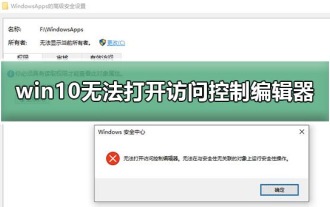
The inability to open the access control editor in win10 is an uncommon problem. This problem usually occurs in external hard drives and USB flash drives. In fact, the solution is very simple. Just open it in safe mode and take a look. Let’s take a look at the details below. tutorial. Win10 cannot open the access control editor 1. In the login interface, hold down shift, click the button, click 2.--, click 3. After restarting, press F5 to try to enter and see if you can enter. Articles related to win10 safe mode>>>How to enter win10 safe mode<<<>>>How to repair the system in win10 safe mode<<<
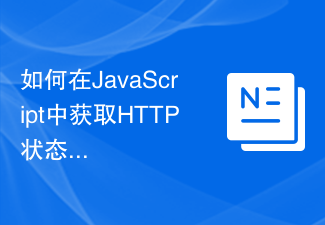
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
