Use of JavaScript scopes
This time I will bring you the use of JavaScript scope, What are the precautions for using JavaScriptscope, the following is a practical case, let’s take a look take a look.
What is scope?
Scope specifies where variables are available.
Function-level scope
1. Variables declared outside the function are global variables, and global variables can be directly accessed within the function:
var global_var = 10; //全局变量function a(){ alert(global_var); //全局变量在函数内可访问}a(); //10
2.JavaScriptvariables Scope is function level. Only functions can generate new scopes, not block level:
function a(){ //函数 if(true){ //块 var x = 1; } if(false){ var y = 2; } alert(x); //1 alert(y); //undefined}a();
Although variable x is declared and assigned in a block statement (if) , but its scope is function a, so it is accessible anywhere in function a.
What’s interesting is that although the declaration and assignment of the y variable are in the false block statement, it still prints undefined instead of reporting an error, because JavaScript will advance all variable declarations to the beginning of the function, which is called Variable promotion :
function a(){ alert(x); var x = 1; alert(x);}a(); //undefined 1//以上代码经过JavaScript变量提升后实际上是这个样子的:function a(){ var x; alert(x); x = 1; alert(x);}
It should be noted that when declaring variables within a function, you must use var, otherwise a global variable is declared:
function test(){ a = 1;}test();alert(a); //1
3. Nested functions can access peripheral functions Variables
function a(){ var x = 1; function b(){ alert(x); var y = 2; function c(){ alert(x); alert(y); } c(); } b();}a(); // 1 1 2
It should be noted that if there is a variable with the same name in a nested function, then the variable in the nested function is accessed
function a(){ var x = 1; function b(){ var x = 2; alert(x); } b(); alert(x);}a(); //2 1
How to achieve block-level scope
Through the above example, we understand that JavaScript variable scope is function level, but sometimes we want to use temporary variables, what should we do?
Achieved through IIFE (immediate execution of function expression):
function a(){ if(true){ (function(){ //IIFE开始 var x = 1; alert(x); //1 }()); //IIFE结束 //alert(x); //这儿访问不到 } //alert(x); //这儿访问不到}a();
The advantage of this is that it will not cause variable pollution and will be used up. That’s it. We have agreed not to contact each other after tonight.
Scope chain
Every time the JavaScript interpreter enters a function, it will look at what local variables are nearby and save them into the function's variables object , and create a scope attribute to point to the external variables object
1. var x=1;2. function a() {3. var y = 2;4. function b() {5. var z = 3;6. alert(x+y+z);7. }8. b();9. }10. a();
Combined with the above code, see how the JavaScript engine handles the scope:
-
JavaScript puts all global objects (variable x and function a) into the global variables object
global variables: x, a()
-
Find that a is a Function, it requires a scope attribute to point to external variables (global) and save the variables
a.scope -> global variables
a.variables: y, b()
-
Coming to line 4, we found that b is a function, which needs a scope attribute to point to the scope in which it is located (a)
b.scope -> a.variables
b.variables: z
When looking for a variable, first check if there is a variable object. If not, look for the upper-level variable based on the scope. Just go up layer by layer in this way until you find it.
# I believe you have mastered the method after reading the case in this article. For more exciting information, please pay attention to other related articles on the php Chinese website!
Recommended reading:
How to extract numbers from strings using regular expressions
What class definition components are there in React
The above is the detailed content of Use of JavaScript scopes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
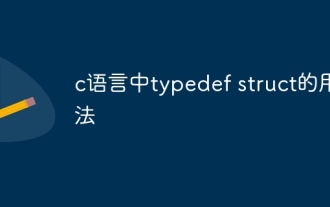
typedef struct is used in C language to create structure type aliases to simplify the use of structures. It aliases a new data type to an existing structure by specifying the structure alias. Benefits include enhanced readability, code reuse, and type checking. Note: The structure must be defined before using an alias. The alias must be unique in the program and only valid within the scope in which it is declared.
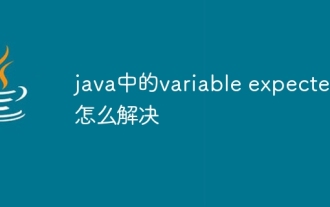
Variable expected value exceptions in Java can be solved by: initializing variables; using default values; using null values; using checks and assignments; and knowing the scope of local variables.
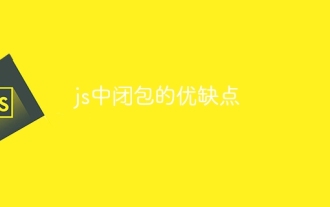
Advantages of JavaScript closures include maintaining variable scope, enabling modular code, deferred execution, and event handling; disadvantages include memory leaks, increased complexity, performance overhead, and scope chain effects.
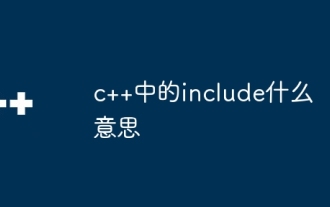
The #include preprocessor directive in C++ inserts the contents of an external source file into the current source file, copying its contents to the corresponding location in the current source file. Mainly used to include header files that contain declarations needed in the code, such as #include <iostream> to include standard input/output functions.
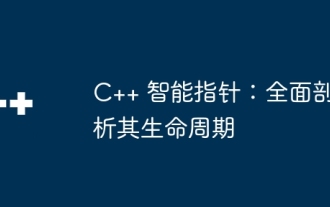
Life cycle of C++ smart pointers: Creation: Smart pointers are created when memory is allocated. Ownership transfer: Transfer ownership through a move operation. Release: Memory is released when a smart pointer goes out of scope or is explicitly released. Object destruction: When the pointed object is destroyed, the smart pointer becomes an invalid pointer.
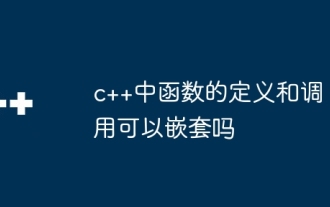
Can. C++ allows nested function definitions and calls. External functions can define built-in functions, and internal functions can be called directly within the scope. Nested functions enhance encapsulation, reusability, and scope control. However, internal functions cannot directly access local variables of external functions, and the return value type must be consistent with the external function declaration. Internal functions cannot be self-recursive.
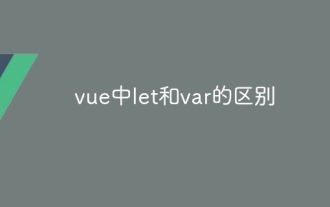
In Vue, there is a difference in scope when declaring variables between let and var: Scope: var has global scope and let has block-level scope. Block-level scope: var does not create a block-level scope, let creates a block-level scope. Redeclaration: var allows redeclaration of variables in the same scope, let does not.
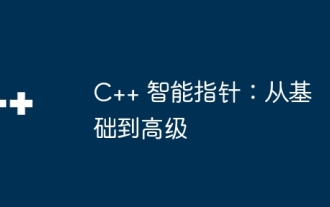
Smart pointers are C++-specific pointers that can automatically release heap memory objects and avoid memory errors. Types include: unique_ptr: exclusive ownership, pointing to a single object. shared_ptr: shared ownership, allowing multiple pointers to manage objects at the same time. weak_ptr: Weak reference, does not increase the reference count and avoid circular references. Usage: Use make_unique, make_shared and make_weak of the std namespace to create smart pointers. Smart pointers automatically release object memory when the scope ends. Advanced usage: You can use custom deleters to control how objects are released. Smart pointers can effectively manage dynamic arrays and prevent memory leaks.
