


Reflection: Understanding the essence of collection generics through reflection
This article is continued from the above "Basic Operations of Reflection Method Reflection", using reflection to understand the nature of generics in java collections
1. Initialize two collections, one using generics type, one does not use
1 ArrayList list1 = new ArrayList();2 ArrayList<String> list2 = new ArrayList<String>();
2. If there is a defined type, adding the int type to list2 will report an error
1 list2.add("Hello");2 list2.add(20); //报错
3. Get two Compare the class types of objects
1 Class c1 = list1.getClass();2 Class c2 = list2.getClass();3 System.out.println(c1 == c2);
The c1==c2 result returns true, indicating that the generics of the collection are degenerated after compilation. The generics of the collection in Java are In order to prevent incorrect input, it is only valid during the compilation phase. If you bypass compilation, it will be invalid.
4. Verification: Bypass compilation through method reflection
1 try {2 Method m = c2.getMethod("add", Object.class);3 m.invoke(list2,20);4 System.out.println(list2);5 } catch (Exception e) {6 e.printStackTrace();7 }
5 , Output result
6, Complete code


1 package com.format.test; 2 3 import java.lang.reflect.Method; 4 import java.util.ArrayList; 5 6 /** 7 * Created by Format on 2017/6/4. 8 */ 9 public class Test2 {10 public static void main(String[] args) {11 ArrayList list1 = new ArrayList();12 ArrayList<String> list2 = new ArrayList<String>();13 list2.add("Hello");14 // list2.add(20); //报错15 Class c1 = list1.getClass();16 Class c2 = list2.getClass();17 System.out.println(c1 == c2);18 /**19 * 反射操作都是编译之后的操作20 * c1==c2结果返回true,说明编译之后集合的泛型是去泛型化的21 * java中集合的泛型是为了防止错误输入的,只在编译阶段有效,绕过编译就无效了22 * 验证:通过方法的反射来绕过编译23 */24 try {25 Method m = c2.getMethod("add", Object.class);26 m.invoke(list2,20);27 System.out.println(list2);28 } catch (Exception e) {29 e.printStackTrace();30 }31 }32 }
The above is the detailed content of Reflection: Understanding the essence of collection generics through reflection. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










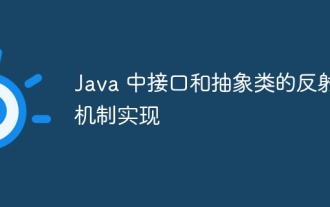
The reflection mechanism allows programs to obtain and modify class information at runtime. It can be used to implement reflection of interfaces and abstract classes: Interface reflection: obtain the interface reflection object through Class.forName() and access its metadata (name, method and field) . Reflection of abstract classes: Similar to interfaces, you can obtain the reflection object of an abstract class and access its metadata and non-abstract methods. Practical case: The reflection mechanism can be used to implement dynamic proxies, intercepting calls to interface methods at runtime by dynamically creating proxy classes.
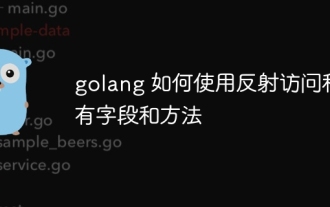
You can use reflection to access private fields and methods in Go language: To access private fields: obtain the reflection value of the value through reflect.ValueOf(), then use FieldByName() to obtain the reflection value of the field, and call the String() method to print the value of the field . Call a private method: also obtain the reflection value of the value through reflect.ValueOf(), then use MethodByName() to obtain the reflection value of the method, and finally call the Call() method to execute the method. Practical case: Modify private field values and call private methods through reflection to achieve object control and unit test coverage.
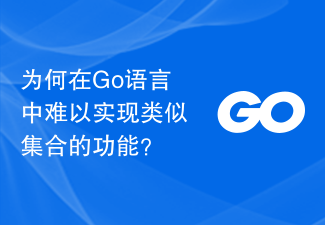
It is difficult to implement collection-like functions in the Go language, which is a problem that troubles many developers. Compared with other programming languages such as Python or Java, the Go language does not have built-in collection types, such as set, map, etc., which brings some challenges to developers when implementing collection functions. First, let's take a look at why it is difficult to implement collection-like functionality directly in the Go language. In the Go language, the most commonly used data structures are slice and map. They can complete collection-like functions, but
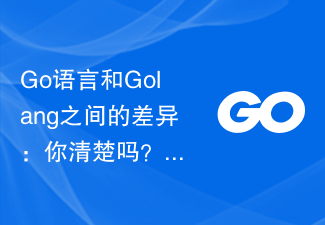
Go and Golang are the same programming language and there is no substantial difference between them. Go is the official name of the programming language, and Golang is the abbreviation commonly used by Go language developers in the Internet field. In this article, we will explore the characteristics, uses, and some specific code examples of the Go language to help readers better understand this powerful programming language. Go language is a statically compiled programming language developed by Google. It has the characteristics of efficiency, simplicity, and strong concurrency, and is designed to improve programmers' work efficiency.
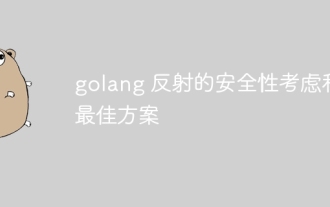
Reflection provides type checking and modification capabilities in Go, but it has security risks, including arbitrary code execution, type forgery, and data leakage. Best practices include limiting reflective permissions, operations, using whitelists or blacklists, validating input, and using security tools. In practice, reflection can be safely used to inspect type information.
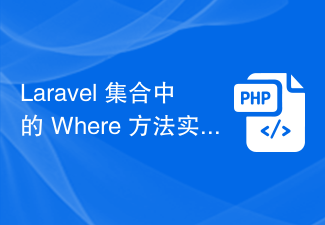
Practical Guide to Where Method in Laravel Collections During the development of the Laravel framework, collections are a very useful data structure that provide rich methods to manipulate data. Among them, the Where method is a commonly used filtering method that can filter elements in a collection based on specified conditions. This article will introduce the use of the Where method in Laravel collections and demonstrate its usage through specific code examples. 1. Basic usage of Where method
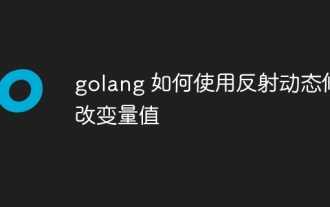
Go language reflection allows you to manipulate variable values at runtime, including modifying Boolean values, integers, floating point numbers, and strings. By getting the Value of a variable, you can call the SetBool, SetInt, SetFloat and SetString methods to modify it. For example, you can parse a JSON string into a structure and then use reflection to modify the values of the structure fields. It should be noted that the reflection operation is slow and unmodifiable fields cannot be modified. When modifying the structure field value, the related fields may not be automatically updated.
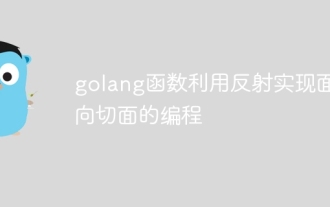
Answer: Yes, reflection in Go language can implement aspect-oriented programming. Detailed description: Reflection allows a program to modify and inspect its own types and values at runtime. Through reflection, we can create global aspects for the code, which are triggered before and after the function is executed. This allows us to easily add functionality such as logging without modifying existing code. Reflection provides the advantages of code decoupling, scalability, and flexible control, thereby improving application maintainability and reusability.
