


Detailed explanation of decimal to binary, hexadecimal and octal conversion in C#
1. Convert decimal to binary
Continuously divide the decimal number by 2 and fill in all the remainders backwards to get the required binary data.
public static string DecimalToBinary(int vDecimal) {/* 将十进制的数 vDecimal 不断地除 2,取余数 * 然后将余数 倒序 填写 */List<int> vYuShu = new List<int>(); // 除 2 过程中产生的余数集int vTempValue= vDecimal; // 除 2 过程中产生的商数for (; ; ) {int tempYS = vTempValue % 2; vYuShu.Add(tempYS); // 记住余数vTempValue = vTempValue / 2;if (vTempValue == 0) // 商数等于0时,结束运算break; }// 倒序输出string strBinary = "";for (int i = vYuShu.Count - 1; i >= 0; i--) { strBinary += vYuShu[i]; } Console.WriteLine("Input decimal value:{0}, output binary value:{1}.", vDecimal, strBinary);return strBinary; }
##2. Convert binary to decimal
Multiply the value (0 or 1) in each binary digit by 2 raised to the (n-1) power, and convert each bit The results are added. Among them, n represents the number of digits from right to left in binary (counting from 1);public static int BinaryToDecimal(string vBinary) {// 首先判断是否满足输入要求int[] vInput = new int[vBinary.Length];for (int i = 0; i < vBinary.Length; i++) {var tempNum = vBinary[i].ToString();if (tempNum == "0") { vInput[i] = 0; }else if (tempNum == "1") { vInput[i] = 1; }else{throw new Exception("输入参数不正确,二进制数应仅由:0和1组成"); } }/* * 依次乘2的(n-1)次方,再求和 */int vDecimal = 0;for (int i = 1; i <= vInput.Length; i++) { vDecimal += (int)(Math.Pow(2, i - 1) * vInput[vInput.Length-i]); } Console.WriteLine("Input binary value:{0}, output decimal value:{1}.", vBinary, vDecimal);return vDecimal; }
3. Built-in conversion method
C#.Net’s built-in base conversion method:int vDecimal = 99;// 【10】 → 【2】string vBinary = Convert.ToString(vDecimal, 2); Console.WriteLine("十进制数:{0},转换成二进制:{1}", vDecimal, vBinary);// 【2】 → 【10】int tempDecimal = Convert.ToInt32(vBinary, 2); Console.WriteLine("二进制数:{0},转换成十进制:{1}", vBinary, tempDecimal);
#4. Decimal <=> Hexadecimal
int vDecimal = 127;// 【10】 → 【16】string vStrHex = "0x" + Convert.ToString(vDecimal, 16); Console.WriteLine("十进制数:{0},转换成十六进制:{1}", vDecimal, vStrHex);// 【16】 → 【10】int tempDecimal = Convert.ToInt32(vStrHex, 16); Console.WriteLine("十六进制数:{0},转换成十进制:{1}", vStrHex, tempDecimal);
## Or you can:
##5. Decimal<=> Octal
##6. Other conversions
For data with positive and negative signs, the conversion is slightly different from the appeal.
1 byte (8 bits) can only represent 256 numbers anyway. Because it is signed, we express it as a range: -128 → 127.Use the highest bit to represent the sign bit, 0 represents a positive number, and 1 represents a negative number.
10000000 represents the smallest negative integer in the computer. From 10000001 to 11111111, it means -127 to -1.
Negative integers are stored in two's complement form in computers.
public static int BinaryToDecimalWithSign(string vBinary)
{// 首先判断是否满足输入要求int[] vInput = new int[vBinary.Length];for (int i = 0; i < vBinary.Length; i++)
{var tempNum = vBinary[i].ToString();if (tempNum == "0")
{
vInput[i] = 0;
}else if (tempNum == "1")
{
vInput[i] = 1;
}else{throw new Exception("输入参数不正确,二进制数应仅由:0和1组成");
}
}// -------- 不足8bits,补足 --------(非必需)if (vInput.Length % 8 != 0) // 补足8b、16b、、、 {int nLen = (vInput.Length / 8 + 1) * 8;int[] nInput = new int[nLen];for (int i = 0; i < nLen - vInput.Length; i++)
{
nInput[i] = vInput[0];
}
vInput.CopyTo(nInput, nLen - vInput.Length);
vInput = nInput;
}// ---------------------------------// 第1步:首位为1,则为负值int vFH = vInput[0];if (vFH == 1)
{// ---------- 第2步:减去一 ----------for (int i = 1; i <= vInput.Length; i++)
{if (vInput[vInput.Length - i] == 1)
{
vInput[vInput.Length - i] = 0;break;
}else{
vInput[vInput.Length - i] = 1;
}
}// ---------- 第3步:取反 ----------for (int i = 0; i < vInput.Length; i++)
{
vInput[i] = 1 - vInput[i];
}
}// ---------- 第4步:转成10进制数 ----------int vDecimal = 0;for (int i = 1; i <= vInput.Length; i++)
{
vDecimal += (int)(Math.Pow(2, i - 1) * vInput[vInput.Length - i]);
}if (vFH == 1) // 为负数 {
vDecimal = 0 - vDecimal;
}
Console.WriteLine("Input binary value:{0}, output decimal value:{1}.", vBinary, vDecimal);return vDecimal;
}
[]
The above is the detailed content of Detailed explanation of decimal to binary, hexadecimal and octal conversion in C#. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
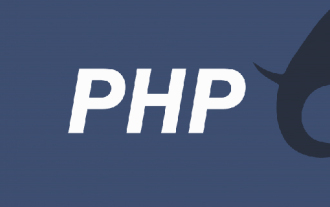
How to convert a hexadecimal string to a number in PHP: 1. Use the hexdec() function with the syntax "hexdec(hexdecimal string)"; 2. Use the base_convert() function with the syntax "bindec(hexadecimal string)" String, 16, 10)".

EDVAC has two major improvements: one is the use of binary, and the other is the completion of stored programs, which can automatically advance from one program instruction to the next, and its operations can be automatically completed through instructions. "Instructions" include data and programs, which are input into the memory device of the machine in the form of codes, that is, the same memory device that stores data is used to store instructions for performing operations. This is the new concept of so-called stored programs.
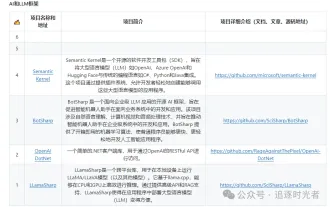
The development of artificial intelligence (AI) technologies is in full swing today, and they have shown great potential and influence in various fields. Today Dayao will share with you 4 .NET open source AI model LLM related project frameworks, hoping to provide you with some reference. https://github.com/YSGStudyHards/DotNetGuide/blob/main/docs/DotNet/DotNetProjectPicks.mdSemanticKernelSemanticKernel is an open source software development kit (SDK) designed to integrate large language models (LLM) such as OpenAI, Azure

Binary arithmetic is an operation method based on binary numbers. Its basic operations include addition, subtraction, multiplication and division. In addition to basic operations, binary arithmetic also includes logical operations, displacement operations and other operations. Logical operations include AND, OR, NOT and other operations, and displacement operations include left shift and right shift operations. These operations have corresponding rules and operand requirements.
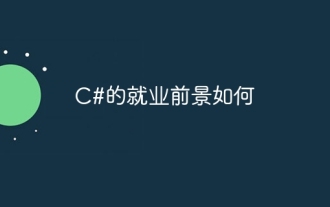
Whether you are a beginner or an experienced professional, mastering C# will pave the way for your career.

Binary numbers are represented by 1s and 0s. The 16-bit hexadecimal number system is {0,1,2,3…..9,A(10),B(11),…F(15)} in order to convert from binary representation to hexadecimal Represents that the bit string ID is grouped into 4-bit chunks, called nibbles starting from the least significant side. Each block is replaced with the corresponding hexadecimal number. Let us see an example to get a clear understanding of hexadecimal and binary number representation. 001111100101101100011101 3 E 5 B&nb
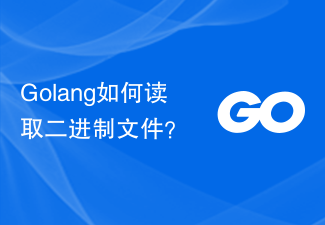
How to read binary files in Golang? Binary files are files stored in binary form that contain data that a computer can recognize and process. In Golang, we can use some methods to read binary files and parse them into the data format we want. The following will introduce how to read binary files in Golang and give specific code examples. First, we need to open a binary file using the Open function from the os package, which will return a file object. Then we can make

The main reasons why computers use binary systems: 1. Computers are composed of logic circuits. Logic circuits usually only have two states, the switch is on and off, and these two states can be represented by "1" and "0"; 2. Only two numbers, 0 and 1, are used in the binary system, which is less error-prone during transmission and processing, thus ensuring high reliability of the computer.
