


Detailed explanation of php connecting mysql data through Mysqli and PDO
Preface
In actual development, database operation classes are rarely written by themselves, and are mostly implemented through some frameworks. Suddenly I still need to borrow manuals to write it myself, so I think it is necessary to summarize the method of connecting php to mysql. php can connect to mysql through mysql extension, mysqli extension, and pdo extension, because higher versions of php will be removed. mysql_ series of methods, so only the other two connection methods are summarized here. First we have to make sure that these two extensions of php are turned on. Check the php.ini configuration file as follows:
Tips, if anyone says that I opened the extension library (that is, removed the ';' in front), but still keeps prompting that the mysqli_ or pdo series methods cannot be found, this is probably because you The directory where the extension library is located is not specified. Find the extension_dir parameter and specify the directory where the extension is located
extension_dir = "D:/wamp/bin/php/php5.5.12/ext/"
Configuration file
First We will separate the configuration files needed to connect to the database, so that we don’t need to write them every time. When needed, just include or require them directly. If you are not clear about include and require, you can check http://www.php.cn/
File name conf.php
##
return array( 'host'=>'127.0.0.1', 'user'=>'root', 'password'=>'',//因为测试,我就不设置密码,实际开发中,必须建立新的用户并设置密码 'dbName'=>'xxpt', 'charSet'=>'utf8', 'port'=>'3306' );
Connect through mysqli extension
mysqli has two ways to connect to mysql and supports preprocessing. One One is object-oriented and the other is process-oriented.
1. Process-oriented connection mysql
##
$dbConf=include 'conf.php'; function openDb($dbConf){ $conn=mysqli_connect($dbConf['host'],$dbConf['user'],$dbConf['password'],$dbConf['dbName'],$dbConf['port']) or die('打开失败'); //当然如上面不填写数据库也可通过mysqli_select($conn,$dbConf['dbName'])来选择数据库 mysqli_set_charset($conn,$dbConf['charSet']);//设置编码 return $conn; } function closeDb($conn){ mysqli_close($conn); } //1.打开连接 $conn=openDb($dbConf); //2query方法执行增、查、删、改 $sql='SELECT t.`id1` from `t1` as t'; /*************数据查询***************************/ $rs=$conn->query($sql); //从结果集中读取数据 //fetch_assoc:返回键值对形式,键位字段名、fetch_row:返回键值对形式,键值为数值、fetch_array:返回1和2两种形式的组合 $data=array();//保存数据 while($tmp=mysqli_fetch_assoc($rs)){//每次从结果集中取出一行数据 $data[]=$tmp; } //对数据进行相应的操作 print_r($data);//输出数据 /*************数据插入***************************/ $sql='INSERT INTO `t1`(`id1`,`id2`) VALUES(3,4);'; $rs=$conn->query($sql); //3.关闭连接 closeDb($conn);
2. Object-oriented connection to mysql
$dbConf=include 'conf.php'; //打开 $conn=new mysqli($dbConf['host'],$dbConf['user'],$dbConf['password'],$dbConf['dbName'],$dbConf['port']); if(!$conn){ die('数据库打开失败'); } //执行增删改查 /*************数据查询***************************/ $sql='SELECT t.`id1` from `t1` as t'; $rs=$conn->query($sql);//获取结果集 //通过fetch_assoc、fetch_array、fetch_row从结果集中获取数据 while ($tmp=$rs->fetch_assoc()) { print_r($tmp); } /*************数据删除***************************/ $sql='DELETE FROM `t1` WHERE `id1`=3'; $rs=$conn->query($sql);//获取结果集 print_r($rs);$conn->close();
3.
PreprocessingMain explanation Mysli object programming preprocessing, as for process-oriented preprocessing using mysqli_prepare, I will not introduce it
$dbConf=include 'conf.php'; //打开 $conn=new mysqli($dbConf['host'],$dbConf['user'],$dbConf['password'],$dbConf['dbName'],$dbConf['port']); if(!$conn){ die('数据库打开失败'); } //执行增删改查 /*************数据查询***************************/ $sql='SELECT * from `t1` as t WHERE id2>?'; $stmt=$conn->prepare($sql); if(!$stmt){ die('sql语句有问题'); } //绑定参数 $id2=2; $stmt->bind_param('i',$id2);//不能写成bind_param('i',2) //执行 $stmt->execute(); //将结果绑定发到指定的参数上 $stmt->bind_result($id1, $id2); //获取结果 while ($tmp=$stmt->fetch()) { print_r('id1='.$id1.',id2='.$id2); echo '</br>'; } //关闭 $stmt->free_result();//释放结果 $stmt->close();//关闭预编译的指令. $conn->close();//关闭连接
The parameter types in the preprocessing binding parameters are explained as follows
##php uses PDO to connect to mysql
$dbConf=include 'conf.php';
//打开
$pdo=myPDO::getInstance($dbConf);
/*************数据查询***************************/
$sql='SELECT t.`id1` from `t1` as t';
$rs=$pdo->query($sql);
$data=$rs->fetchAll();//取出所有结果
print_r($data);
/*************数据更新***************************/
$sql='UPDATE t1 SET t1.`id1`=11 WHERE t1.`id1`=1';
$rs=$pdo->query($sql);
/**
* 数据库pdo连接
*/
class myPDO{
private static $pdo;
private function __construct(){
//code
}
private function __clone(){
//code
}
/**
* 获取实例化的PDO,单例模式
* @return PDO
*/
public static function getInstance($dbConf){
if(!(self::$pdo instanceof PDO)){
$dsn ="mysql:host=".$dbConf['host'].";port=".$dbConf['port'].";dbname=".$dbConf['dbName'].";
charset=".$dbConf['charSet'];
try {
self::$pdo = new PDO($dsn,$dbConf['user'], $dbConf['password'],
array(PDO::ATTR_PERSISTENT => true,PDO::MYSQL_ATTR_INIT_COMMAND => "SET NAMES utf8")); //保持长连接
self::$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_WARNING);
} catch (PDOException $e) {
print "Error:".$e->getMessage()."<br/>";
die();
}
}
return self::$pdo;
}
}
Copy after loginpdo supports preprocessing, and it is recommended to use preprocessing to prevent sql injection.
$dbConf=include 'conf.php'; //打开 $pdo=myPDO::getInstance($dbConf); /*************数据查询***************************/ $sql='SELECT t.`id1` from `t1` as t'; $rs=$pdo->query($sql); $data=$rs->fetchAll();//取出所有结果 print_r($data); /*************数据更新***************************/ $sql='UPDATE t1 SET t1.`id1`=11 WHERE t1.`id1`=1'; $rs=$pdo->query($sql); /** * 数据库pdo连接 */ class myPDO{ private static $pdo; private function __construct(){ //code } private function __clone(){ //code } /** * 获取实例化的PDO,单例模式 * @return PDO */ public static function getInstance($dbConf){ if(!(self::$pdo instanceof PDO)){ $dsn ="mysql:host=".$dbConf['host'].";port=".$dbConf['port'].";dbname=".$dbConf['dbName']."; charset=".$dbConf['charSet']; try { self::$pdo = new PDO($dsn,$dbConf['user'], $dbConf['password'], array(PDO::ATTR_PERSISTENT => true,PDO::MYSQL_ATTR_INIT_COMMAND => "SET NAMES utf8")); //保持长连接 self::$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_WARNING); } catch (PDOException $e) { print "Error:".$e->getMessage()."<br/>"; die(); } } return self::$pdo; } }
The above is the detailed explanation of PHP connecting mysql data through Mysqli and PDO. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
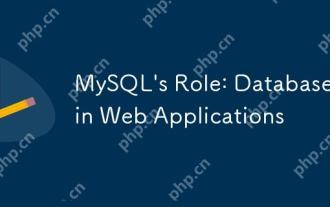
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
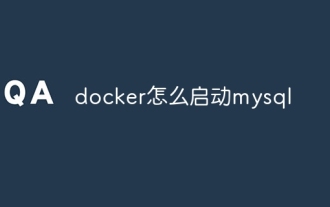
The process of starting MySQL in Docker consists of the following steps: Pull the MySQL image to create and start the container, set the root user password, and map the port verification connection Create the database and the user grants all permissions to the database
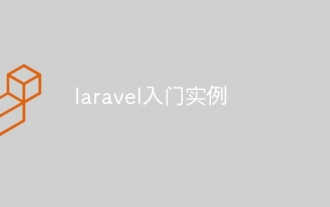
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
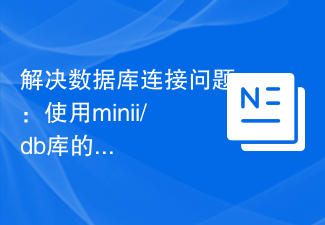
I encountered a tricky problem when developing a small application: the need to quickly integrate a lightweight database operation library. After trying multiple libraries, I found that they either have too much functionality or are not very compatible. Eventually, I found minii/db, a simplified version based on Yii2 that solved my problem perfectly.
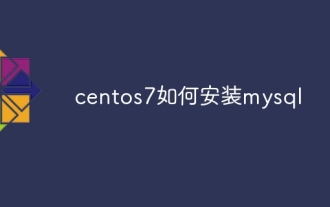
The key to installing MySQL elegantly is to add the official MySQL repository. The specific steps are as follows: Download the MySQL official GPG key to prevent phishing attacks. Add MySQL repository file: rpm -Uvh https://dev.mysql.com/get/mysql80-community-release-el7-3.noarch.rpm Update yum repository cache: yum update installation MySQL: yum install mysql-server startup MySQL service: systemctl start mysqld set up booting
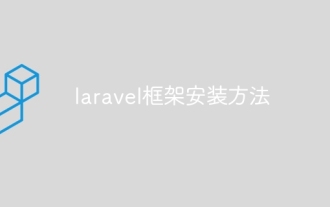
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.
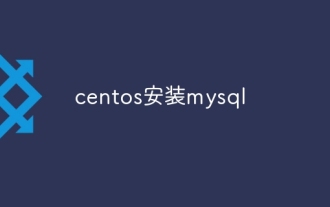
Installing MySQL on CentOS involves the following steps: Adding the appropriate MySQL yum source. Execute the yum install mysql-server command to install the MySQL server. Use the mysql_secure_installation command to make security settings, such as setting the root user password. Customize the MySQL configuration file as needed. Tune MySQL parameters and optimize databases for performance.

MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
