


Detailed explanation of function throttling that triggers the onScroll event in JavaScript
Problem Description
Common website layout, a navigation bar at the top, we assume that this page has four columns: A, B, C, D, we click A, the anchor point jumps Go to column A, and the A button at the top is highlighted; click B, and the anchor jumps to column B, and the B button at the top is highlighted; we scroll in the Main component, and when we scroll to the B module, the B button is highlighted. The above is a model we often encounter in development. If you used jQuery for front-end development in the past, you would be very familiar with it.
Solution
I mainly want to talk about performance optimization methods in React component development.
Our page structure is like this
<div className={style.main} id="main" ref={(main) => { this.main = main; }} onScroll={ ((/detail/.test(this.props.location.pathname))) ? (() => this.throttle()()) : null } > {this.props.children} <Footer />
We set the onScroll event in the main component. In this event, we trigger the action and pass the status change to Subassembly.
My scroll event triggering function is like this (ignore the long list of if else, this is the ultimate solution to solve the bug for an afternoon, this article will not go into detail)
handleScroll() { const { changeScrollFlag } = this.props.actions; // 根据滚动距离修改TitleBox的样式 const { basicinformation, holderinformation, mainpeople, changerecord } = { basicinformation: document.getElementById('basicinformation').offsetTop - 121, holderinformation: document.getElementById('holderinformation').offsetTop - 121, mainpeople: document.getElementById('mainpeople').offsetTop - 121, changerecord: document.getElementById('changerecord').offsetTop - 121, }; if (window.screen.availHeight > this.main.scrollTop) { document.getElementById('gototop').style.display = 'none'; } else { document.getElementById('gototop').style.display = 'block'; } // 得到基础信息区域、股东信息区域、主要人员区域、变更记录区域的offsetTop,我们把它用来跟main的scrollTop比较 // 比较的结果触发action,改变TitleBox组件样式 if (this.main.scrollTop < holderinformation) { // 基础信息区域 if (basicinformation === -121) { // 如果基础信息模块不存在,我们什么也不做(当然理论上基础信息模块应该是会有的) return; } changeScrollFlag(1); return; } else if (this.main.scrollTop < mainpeople) { // 股东信息区域 changeScrollFlag(2); if (holderinformation === -121) { // 如果股东信息栏目不存在,在滚动的时候我们不应该强行把TileBox的高亮按钮设置为holderinformation // 因为holdinformation并不存在,我们跳到前一个按钮,让基础信息按钮高亮 changeScrollFlag(1); return; } return; } else if (this.main.scrollTop < changerecord) { // 主要人员区域 changeScrollFlag(3); if (mainpeople === -121) { // 如果主要人员栏目不存在,在滚动的时候我们不应该强行把TileBox的高亮按钮设置为mainpeople // mainpeople并不存在,我们跳到前一个按钮,让基础信息按钮高亮 changeScrollFlag(2); if (holderinformation === -121) { // 如果主要人员栏目不存在,而且连股东信息栏目也没有,我们跳到高亮基础信息栏目 changeScrollFlag(1); return; } return; } return; } else if (this.main.scrollTop > changerecord) { // 与上面同理 // 变更记录区域 changeScrollFlag(4); if (changerecord === -121) { changeScrollFlag(3); if (mainpeople === -121) { changeScrollFlag(2); if (holderinformation === -121) { changeScrollFlag(1); return; } return; } return; } return; } }
Among them, the changeScrollFlag() function is our action processing function.
Our function throttling
throttle() { // onScroll函数节流 let previous = 0; // previous初始设置上一次调用 onScroll 函数时间点为 0。 let timeout; const wait = 250; // 250毫秒触发一次 return () => { const now = Date.now(); const remaining = wait - (now - previous); if (remaining <= 0) { if (timeout) { window.clearTimeout(timeout); } previous = now; timeout = null; this.handleScroll(); } else if (!timeout) { timeout = window.setTimeout(this.handleScroll, wait); } }; }
Our throttling function returns a function that sets a timestamp. If the difference in our timestamps is smaller, we do nothing. Do it, but the difference between our timestamps is large, clear the timer, and trigger the scroll function. This seems to be quite simple, yes, it is indeed quite simple.
So what else do we need to do in the sub-component?
Receive action
The second-level container component receives the action and passes props to the third-level display component through the second-level container component.
We must receive this props in componentWillReceiveProps.
Remember, using this.props in componentWillReceiveProps will not receive changes in props! ! ! The component lifecycle function contains a parameter of its own.
componentWillReceiveProps(nextProps) { // 在compoWillReceiveProps里接收到Main组件里所触发onScroll事件的改变activebtn样式的index // 并且设置为本组件的state this.setState({ activebtn: nextProps.scrollFlag.scrollIndex, }); }
Our state controls which button we highlight, which is a number.
Change the style of the navigation bar
<span className={classnames({ [style.informationactive]: (this.state.activebtn === 1), })} onClick={() => this.handleClick(1, 'basicinformation')} >
Here, we have completed triggering an event from the top-level component, and achieved function throttling, passing the event layer by layer. A process to get to the underlying presentation components.
Some recent feelings about front-end development
Don’t call a function repeatedly in a component, this will cause huge consumption! Don't write a new function for what we can do with the ternary operator and template strings.
jsx Don’t be too redundant. We try to write it in the form of variables, otherwise the page structure will be complicated and it will be difficult for us to catch bugs.
Reduce back-end requests, save cookies if you can, and save localStorge if you can save localStorge.
Try to write simple components by yourself. Do not use other people’s components. Otherwise, you will have huge difficulties in changing requirements and adjusting styles, and you will do some meaningless things.
Summary
The above is the entire content of this article. I hope the content of this article can bring some help to everyone's study or work. If you have any questions, you can leave a message to communicate.
For more detailed explanations of function throttling that triggers the onScroll event in JavaScript, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










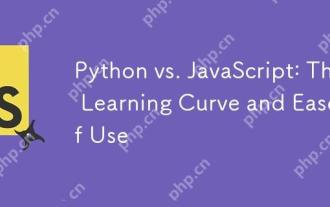
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
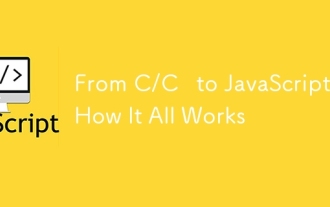
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
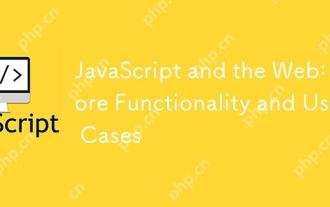
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
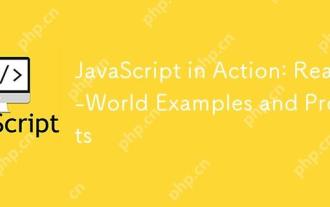
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
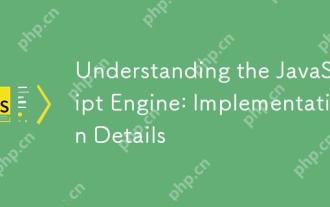
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.
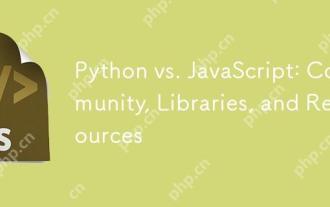
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
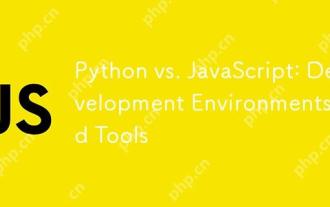
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
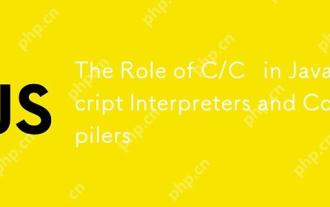
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
