


Tips for nesting if and else statements in for loops in Python
for...[if]...Construct List (List comprehension)
1. Simple for...[if]... statement
In Python, the for...[if]... statement is a concise way to construct a List. It selects elements that meet the if condition from the list given by for to form a new List, where if can be omitted. of. Here are a few simple examples to illustrate.
>>> a=[12, 3, 4, 6, 7, 13, 21] >>> newList = [x for x in a] >>> newList [12, 3, 4, 6, 7, 13, 21] >>> newList2 = [x for x in a if x%2==0] >>> newList2 [12, 4, 6]
With if omitted, newList constructs a List with the same elements as a. However, newList and a are different Lists. Executing b=a, b and newList are different. newList2 is a List composed of elements selected from a that satisfy x%2==0. If you do not use the for...[if].. statement, the following operations are required to build newList2.
>>> newList2=[] >>> for x in a: ... if x %2 == 0: ... newList2.append(x) >>> newList2 [12, 4, 6]
Obviously, using the for...[if]... statement is more concise.
2. Nested for...[if]... statements
The nested for...[if]... statement can select elements that meet the if condition from multiple Lists to form a new List. Here are a few examples.
>>>a=[12, 3, 4, 6, 7, 13, 21] >>>b=['a', 'b', 'x'] >>>newList=[(x, y) for x in a for y in b] >>>newList [(12, 'a'), (12, 'b'), (12, 'x'), (3, 'a'), (3, 'b'), (3, 'x'), (4, 'a'), (4, 'b'), (4, 'x'), (6, 'a'), (6, 'b'), (6, 'x'), (7, 'a'), (7, 'b'), (7, 'x'), (13, 'a'), (13, 'b'), (13, 'x'), (21, 'a'), (21, 'b'), (21, 'x')] >>>newList2=[(x, y) for x in a for y in b if x%2==0 and y<'x'] >>>newList2 [(12, 'a'), (12, 'b'), (4, 'a'), (4, 'b'), (6, 'a'), (6, 'b')]
The nested for...[if]... statement is equivalent to multiple for statements. The first for statement is the outermost loop.
Make good use of python’s else clause
In daily coding, the use of branch statements is very common. The logic of code execution is often controlled based on whether certain conditions are met, so everyone will be familiar with if[elif[else]]. The else clause in the branch statement will be executed when other conditions are not met. Appropriate use of branch statements can make our code logic richer.
The use of else clauses in branch statements is basically the same in some common programming languages. It is similar to providing a default execution path and used with conditional judgment statements such as if. Compared with other programming languages (c#, java, js etc.) In python, else has some special usages. It can be used with loop statements such as for and while, and can even be used with exception handling try except statements, which can make our code more concise.
1. Use with for/while loop statement
The else clause is immediately followed by the for loop statement. When the loop ends normally (without early exit such as return or break), the logic of the else clause will be executed. Let’s look at an example first:
def print_prime(n): for i in xrange(2, n): # found = True for j in xrange(2, i): if i % j == 0: # found = False break else: print "{} it's a prime number".format(i) # if found: # print "{} it's a prime number".format(i)
print_prime(7)
Result:
2 it's a prime number 3 it's a prime number 5 it's a prime number
A simple example of printing prime numbers. When judging whether a number is a prime number, you need to traverse integers smaller than itself. If any one satisfies divisibility, the judgment ends. Otherwise, print the info that this is a prime number. With else With the blessing, the logic of the entire example is quite "self-expressive", as easy to understand as pseudo code, and compared to setting the flag value when judging divisibility and then judging the flag value at the end of the function to decide whether to print the number when the prime number message , the code is cleaner and there aren't as many "procedural" preparations to describe how to do.
ps: You can run the commented code in the example to compare the effects.
2. Use with try except error control
In exception handling statements, else has similar usage. When the try code block does not throw any exception, the else statement block will be executed.
def my_to_int(str_param): try: print int(str_param) except ValueError: print 'cannot convert {} to a integer'.format(str_param) else: print 'convert {} to integer successfully'.format(str_param)
my_to_int("123") my_to_int("me123")
123 convert 123 to integer successfully cannot convert me123 to a integer
As shown in the print log, when the conversion is successful and no error occurs, the logic in the else statement will be executed. Of course, this example may not have much practical use, but it can roughly illustrate the role of else in error handling. Uses: Simplify logic, avoid using some flag values, and accurately grasp whether an error occurs to perform some actual operations (for example, if an error occurs when saving data, perform a rollback operation in the else statement block, and then immediately Then you can add finally statements to complete some cleaning operations
.Making good use of the else statement block allows us to write code that is more concise and closer to the semantics of natural language. Of course, it will also be more pythonic. You can slowly understand the subtleties.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










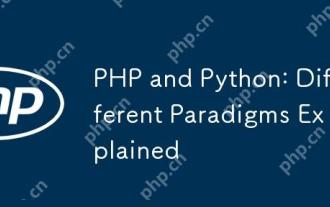
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
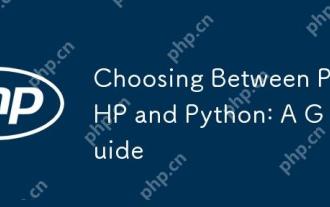
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
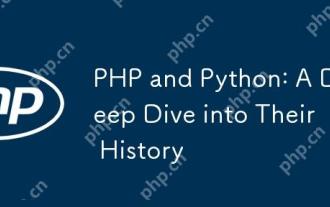
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
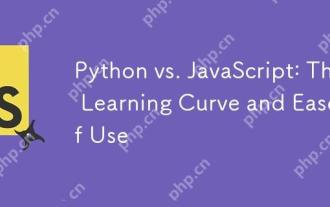
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
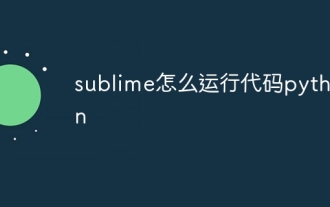
To run Python code in Sublime Text, you need to install the Python plug-in first, then create a .py file and write the code, and finally press Ctrl B to run the code, and the output will be displayed in the console.
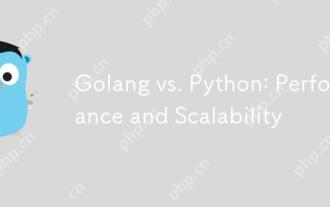
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
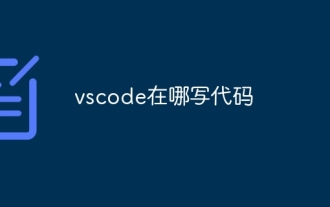
Writing code in Visual Studio Code (VSCode) is simple and easy to use. Just install VSCode, create a project, select a language, create a file, write code, save and run it. The advantages of VSCode include cross-platform, free and open source, powerful features, rich extensions, and lightweight and fast.
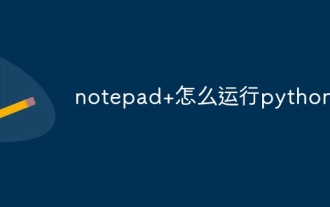
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
