


Detailed explanation of PHP Socket programming process_PHP tutorial
Detailed explanation of PHP Socket programming process
Introduction
Socket is used for inter-process communication. Inter-process communication is usually based on the client-server model. At this point, the client-server are applications that can interact with each other. The interaction between client and server requires a connection. Socket programming is responsible for establishing interactive connections between applications.
In this article, we will learn how to create a simple client-server using PHP. We will also learn how the client application sends messages to the server and how to receive messages from the server.
Use code
Purpose: Develop a client for sending string messages to the server, and the server will reverse the same information and return it to the client.
PHP Server
Step 1: Set variables like "Host" and "Port"
$host = "127.0.0.1";
$port = 5353;
// No Timeout
set_time_limit(0);
The port number can be any positive integer between 1024 -65535.
Step 2: Create socket
$socket = socket_create(AF_INET, SOCK_STREAM, 0) or die("Could not create socketn");
Step 3: Bind socket to port and host
The created socket resource is bound to the IP address and port number.
$result = socket_bind($socket, $host, $port) or die("Could not bind to socketn");
Step 4: Start socket listening
After binding to the IP and port, the server starts waiting for the client to connect. It waits until there is no connection.
$result = socket_listen($socket, 3) or die("Could not set up socket listenern");
Step 5: Accept the connection
This function will accept incoming connection requests from the created socket. After accepting a connection from the client socket, this function returns another socket resource, which is actually responsible for communicating with the corresponding client socket. The "$spawn" here is the socket resource responsible for communicating with the client socket.
$spawn = socket_accept($socket) or die("Could not accept incoming connectionn");
Up to now, we have prepared the server socket, but this script does not actually do anything. So in order to continue to accomplish the above goals, we will read the client socket message, then reverse the received message and send it back to the client socket.
Step 6: Read the message from the client socket
$input = socket_read($spawn, 1024) or die("Could not read inputn");
Step 7: Reverse the Message
$output = strrev($input) . "n";
Step 8: Send message to client socket
socket_write($spawn, $output, strlen ($output)) or die("Could not write outputn");
Close socket
socket_close($spawn);
socket_close($socket);
This completes the server side. Now, we learn how to create a PHP client.
PHP client
The first two steps are the same as the server side.
Step 1: Set variables like "Host" and "Port"
$host = "127.0.0.1";
$port = 5353;
// No Timeout
set_time_limit(0);
Note: The port and host here should be the same as those defined on the server.
Step 2: Create socket
$socket = socket_create(AF_INET, SOCK_STREAM, 0) or die("Could not create socketn");
Step 3: Connect to the server
$result = socket_connect($socket, $host, $port) or die("Could not connect toservern");
At this time, unlike the server, the client socket is not bound to the port and host. Instead, it connects to the server socket and waits to accept connections from the client socket. This step establishes a connection from the client socket to the server socket.
Step 4: Write to the server socket
socket_write($socket, $message, strlen($message)) or die("Could not send data to servern");
In this step, the data from the client socket is sent to the server socket.
Step 5: Read the response from the server
$result = socket_read ($socket, 1024) or die("Could not read server responsen");
echo "Reply From Server :".$result;
Step 6: Close the socket
socket_close($socket);
Complete code
Server server.php)
// set some variables
$host = "127.0.0.1";
$port = 25003;
// don't timeout!
set_time_limit(0);
// create socket
$socket = socket_create(AF_INET, SOCK_STREAM, 0) or die("Could not create socketn");
// bind socket to port
$result = socket_bind($socket, $host, $port) or die("Could not bind to socketn");
// start listening for connections
$result = socket_listen($socket, 3) or die("Could not set up socket listenern");
// accept incoming connections
// spawn another socket to handle communication
$spawn = socket_accept($socket) or die("Could not accept incoming connectionn");
// read client input
$input = socket_read($spawn, 1024) or die("Could not read inputn");
// clean up input string
$input = trim($input);
echo "Client Message : ".$input;
// reverse client input and send back
$output = strrev($input) . "n";
socket_write($spawn, $output, strlen ($output)) or die("Could not write outputn");
// close sockets
socket_close($spawn);
socket_close($socket);
客户端client.php)
$host = "127.0.0.1";
$port = 25003;
$message = "Hello Server";
echo "Message To server :".$message;
// create socket
$socket = socket_create(AF_INET, SOCK_STREAM, 0) or die("Could not create socketn");
// connect to server
$result = socket_connect($socket, $host, $port) or die("Could not connect to servern");
// send string to server
socket_write($socket, $message, strlen($message)) or die("Could not send data to servern");
// get server response
$result = socket_read ($socket, 1024) or die("Could not read server responsen");
echo "Reply From Server :".$result;
// close socket
socket_close($socket);
建立上述文件server.php和client.php)后,执行如下操作:
许可证
这篇文章,以及任何相关的源代码和文件,是经过The Code Project Open License (CPOL)许可的。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










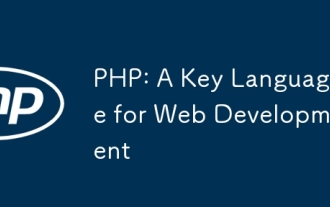
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
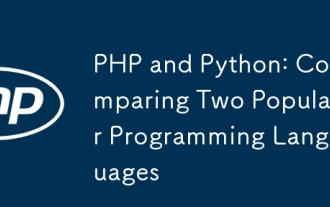
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
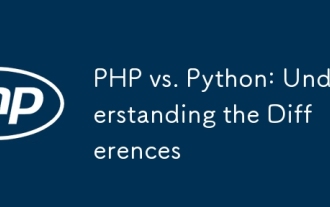
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
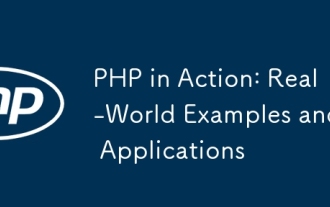
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
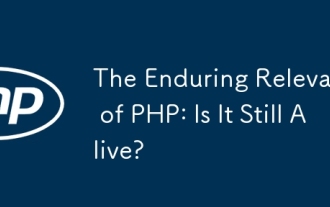
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
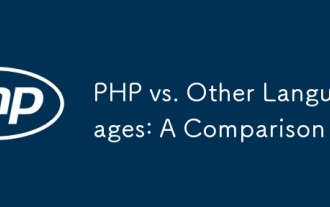
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
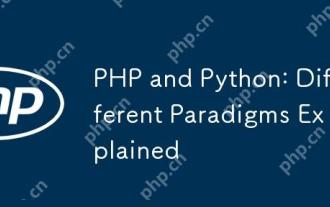
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
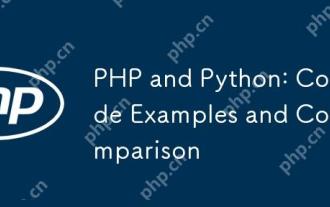
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
