PHP之基础篇1
一. 基本语法
-
开始结束标记: ""
<?php echo "Hello World"?>
Copy after loginNote:文件末尾的 PHP 代码段结束标记"?>"可以不要,有些情况下当使用 include() 或者 require() 时省略掉会更好些。
同java,c一样,php每个语句后需用分号 " ; " 结束。
二. 类型
php支持的8种基本数据类型
四种标量类型:
- boolean (布尔型)
- integer (整型)
- string (字符串)
- float (浮点型)
两种复合类型:
- array (数组型)
- object (对象)
两种特殊类型:
- resource (资源)
- null (null)
Note:
- var_dump() 查看表达式值和类型,is_type() 查看某个类型。
- 整数溢出,如果给定一个整数或运算结果得出的整数超出int范围(2^32),将会解释会float。
三.字符串(string)
声明字符串: 字符串通常用单引号或双引号定义。
在单引号中的变量和特殊含义的字符不会被替换
php > $age = 12; php > echo "他很高\n他的年龄才$age"; 他很高 他的年龄才12; php > echo '他很高\n他的年龄才$age'; 他很高\n他的年龄才$age;
Copy after login字符串可以用'.' (点) 操作符连接起来
php > echo "Hello,"."World"; Hello,World
Copy after login存取和修改字符串中的字符(类似于数组)
php > $str = "Hello,World"; php > echo $str[1]; e php > echo $str{0}; H
Copy after login其他类型转换为字符串(string)
在一个值前面加上(string)或者使用strval()函数来转换成字符串型。
php > $age = 18; php > var_dump($age); int(18) php > $str = (string)$age; php > var_dump($str); string(2) "18"
Copy after login
常用字符串函数
字符串替换
str_replace ($search , $replace , $subject [, int &$count ])
$search: 查找替换目标值
$replace: $search的替换值
subject: 执行替换的数组或者字符串
count: 控制匹配和替换的次数
php > $str = "Hello,My name is Tom,what is your name?"; php > echo str_replace("name","nickname",$str); Hello,My nickname is Tom,what is your nickname?
Copy after login
去掉字符串两边空格
trim ($str)
$str: 目标字符串
php > $str = " Hello "; php > echo trim($str); Hello
Copy after login去掉字符串中html和php标记
strip_tags ($str)
$str: 目标字符串
php > $str = "<td><p>Hello World</p></td>"; php > echo strip_tags($str); Hello World
Copy after login将目标字符串重复多次
str_repeat ($str,int $num)
$str: 目标字符串
$num: 重复次数
php > $str = "Hello World!!"; php > echo str_repeat($str,5); Hello World!!Hello World!!Hello World!!Hello World!!Hello World!!
Copy after login返回字符串长度
strlen ($str)
$str: 目标字符串
php > $str = "Hello World"; php > echo strlen($str); 11
Copy after login计算字串出现的次数
substr_count (string $haystack , string $needle )
$haystack: 目标字符串
$needle: 查找出现次数的字符串
php > $str = "Hello World,Hello China"; php > echo substr_count($str,"Hello"); 2
Copy after login截取字符串
substr ( string $string , int $start [, int $length ] )
$string: 目标字符串
$start: 如果 start 是非负数,返回的字符串将从 string 的 start 位置开始,从 0 开始计算。如果 start 是负数,返回的字符串将从 string 结尾处向前数第 start 个字符开始。如果string 的长度小于或等于 start,将返回 FALSE。
$length: 1.如果提供了正数的 length,返回的字符串将从 start 处开始最多包括 length 个字符(取决于 string 的长度)。2.如果提供了负数的 length,那么 string 末尾处的许多字符将会被漏掉(若 start 是负数则从字符串尾部算起)。3.如果 start 不在这段文本中,那么将返回一个空字符串。4.如果提供了值为 0,FALSE 或 NULL 的 length,那么将返回一个空字符串。5.如果没有提供 length,返回的子字符串将从 start 位置开始直到字符串结尾。
php > $str = "Hello,World"; php > echo substr($str,1); ello,World php > echo substr($str,1,3); ell php > echo substr($str,1,-3); ello,Wo php > echo substr($str,1,10); ello,World
Copy after login

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










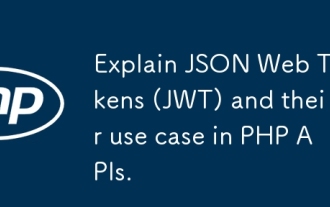
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
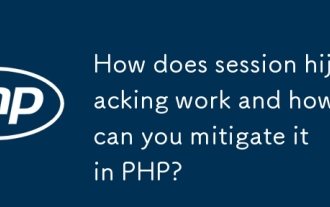
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
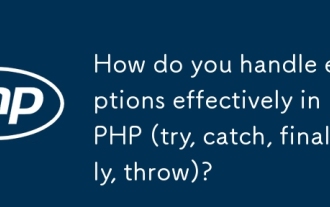
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
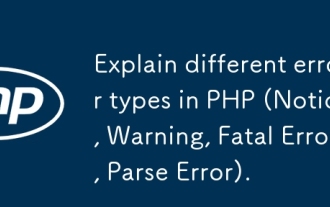
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
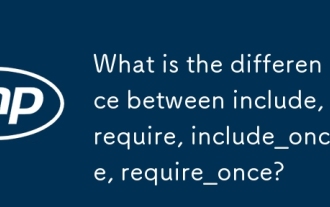
In PHP, the difference between include, require, include_once, require_once is: 1) include generates a warning and continues to execute, 2) require generates a fatal error and stops execution, 3) include_once and require_once prevent repeated inclusions. The choice of these functions depends on the importance of the file and whether it is necessary to prevent duplicate inclusion. Rational use can improve the readability and maintainability of the code.
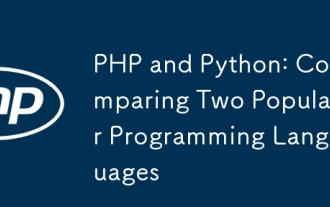
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
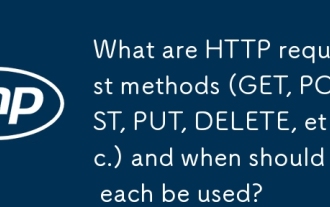
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
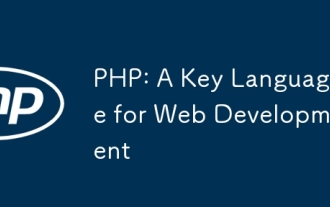
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
