PHP设计模式之享元模式
享元模式属于结构型模式
概述:运用共享技术有效地支持大量细粒度的对象
享元模式:
运用共享技术有效的支持大量细粒度的对象
享元模式变化的是对象的存储开销
享元模式中主要角色:
抽象享元(Flyweight)角色:此角色是所有的具体享元类的超类,为这些类规定出需要实现的公共接口。那些需要外运状态的操作可以通过调用商业以参数形式传入
具体享元(ConcreteFlyweight)角色:实现Flyweight接口,并为内部状态(如果有的话)拉回存储空间。ConcreteFlyweight对象必须是可共享的。它所存储的状态必须是内部的
不共享的具体享元(UnsharedConcreteFlyweight)角色:并非所有的Flyweight子类都需要被共享。Flyweigth使共享成为可能,但它并不强制共享
享元工厂(FlyweightFactory)角色:负责创建和管理享元角色。本角色必须保证享元对象可能被系统适当地共享
客户端(Client)角色:本角色需要维护一个对所有享元对象的引用。本角色需要自行存储所有享元对象的外部状态
享元模式的优点:
Flyweight模式可以大幅度地降低内存中对象的数量
享元模式的缺点:
Flyweight模式使得系统更加复杂
Flyweight模式将享元对象的状态外部化,而读取外部状态使得运行时间稍微变长
享元模式适用场景:
当一下情况成立时使用Flyweight模式:
1 一个应用程序使用了大量的对象
2 完全由于使用大量的对象,造成很大的存储开销
3 对象的大多数状态都可变为外部状态
4 如果删除对象的外部状态,那么可以用相对较少的共享对象取代很多组对象
5 应用程序不依赖于对象标识
享元模式与其它模式:
单例模式(Singleton):客户端要引用享元对象,是通过工厂对象创建或者获得的,客户端每次引用一个享元对象,都是可以通过同一个工厂对象来引用所需要的享元对象。因此,可以将享元工厂设计成单例模式,这样就可以保证客户端只引用一个工厂实例。因为所有的享元对象都是由一个工厂对象统一管理的,所以在客户端没有必要引用多个工厂对象。不管是单纯享元模式还是复合享元模式中的享元工厂角色,都可以设计成为单例模式,对于结果是不会有任何影响的。
Composite模式:复合享元模式实际上是单纯享元模式与合成模式的组合。单纯享元对象可以作为树叶对象来讲,是可以共享的,而复合享元对象可以作为树枝对象,因此在复合享元角色中可以添加聚集管理方法
/**
* 抽象享元角色
*/
abstract class Flyweight{
abstract public function operation($state);
}
/**
* 具体享元角色
*/
class ConcreteFlyweight extends Flyweight{
private $_intrinsicState = null;
/**
* @param $state 内部状态
*/
public function __construct($state){
$this->_intrinsicState = $state;
}
public function operation($state) {
echo 'ConcreteFlyweight operation, Intrinsic State = ' . $this->_intrinsicState
. ' Extrinsic State = ' . $state . '
';
}
}
/**
* 不共享的具体享元,客户端直接调用
*/
class UnsharedConcreteFlyweight extends Flyweight {
private $_intrinsicState = null;
/**
* 构造方法
* @param string $state 内部状态
*/
public function __construct($state) {
$this->_intrinsicState = $state;
}
public function operation($state) {
echo 'UnsharedConcreteFlyweight operation, Intrinsic State = ' . $this->_intrinsicState
. ' Extrinsic State = ' . $state . '
';
}
}
/**
* 享元工厂角色
*/
class FlyweightFactory{
private $_flyweights;
public function __construct(){
$this->_flyweights = array();
}
public function getFlyweight($state){
if(isset($this->_flyweights[$state])){
return $this->_flyweights[$state];
}
return $this->_flyweights[$state] = new ConcreteFlyweight($state);
}
}
/**
* 享元模式
*/
public function actionFlyweight(){
Yii::import('ext.flyweight.*');
$flyweightFactory = new FlyweightFactory();
$flyweightFactory->getFlyweight('state A');
$flyweightFactory->operation('other state A');
$flyweightFactory = new FlyweightFactory();
$flyweightFactory->getFlyweight('state B');
$flyweightFactory->operation('other state B');
$flyweightFactory = new FlyweightFactory();
$flyweightFactory->getFlyweight('state C');
$flyweightFactory->operation('other state C');
/* 不共享的对象,单独调用 */
$uflyweight = new UnsharedConcreteFlyweight('state A');
$uflyweight->operation('other state A');
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










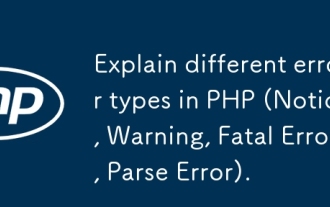
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
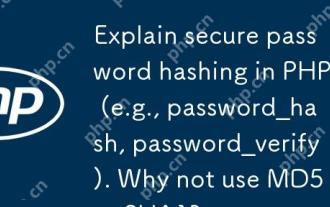
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
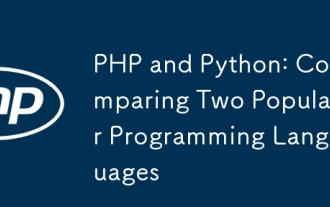
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
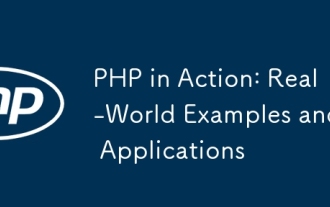
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
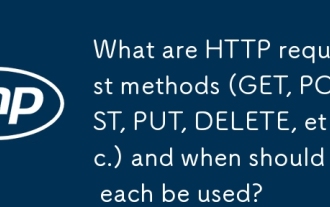
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
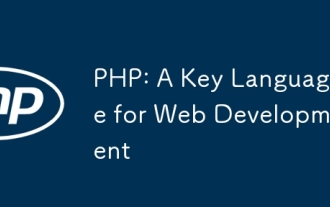
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
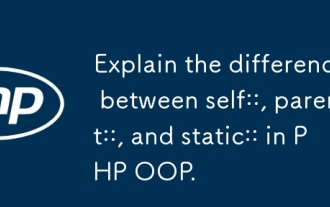
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
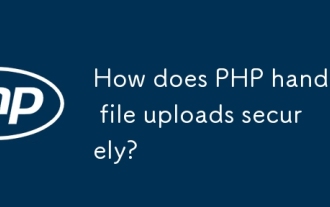
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
