


Detailed explanation of JavaScript function internal attributes and function method examples_javascript skills
A function is an event-driven or reusable block of code that executes when it is called.
Functions are objects with their own properties and methods. First, let’s take a visual look at the function attribute method output under the console:
The internal properties of a function only need to include two special objects: arguments and this.
Function attributes include: length and prototype
Function methods (non-inherited) include: apply() and call()
Inherited function methods: bind(), toString(), toLocaleString(), valueOf()
I’m not familiar with the others at the moment, I’ll add more later
1. Function internal attributes
Inside the function, there are two special objects, arguments and this.
arguments attribute
arguments is an array-like object that contains all parameters passed into the function. The main purpose of arguments is to save function parameters, but this object has a callee attribute, which is a pointer to the function that owns this arguments object, as follows It is a very classic factorial function.
function factorial (num){ if(num <= 1){ return 1; } else{ return num * factorial(num-1); } }
Recursive algorithm is generally used to define the factorial function. As shown in the above code, this definition is fine when there is a function name and the function name will not change in the future. However, the execution of this function is tightly coupled with the function name factorial. In order to eliminate this tight coupling phenomenon (function name changes, etc.), arguments.callee can be used.
function factorial(num){ if(num<=1){ return 1; } else{ return num * arguments.callee(num-1); } }
The function name factorial is no longer referenced in the function body of the rewritten factorial() function. In this way, even if the function name is changed, the recursive call can be completed normally. For example:
var trueFactorial = factorial; //改变原函数体的指针(保存位置) factorial = function (){ //factorial 指向返回0的新函数 return 0; } alert(trueFactorial(5)); //120 alert(factorial(5)); //0
If arguments.callee is not used, then trueFactory(5) also returns 0;
this attribute
2. Function methods
Each function contains two non-inherited methods: apply() and call(). The purpose of these two methods is to call functions in a specific domain (I don’t understand when I see this); their real power lies in the ability to expand the scope in which the function runs
This editor will introduce you to this much about the internal properties and function methods of JavaScript functions. I hope it will be helpful to you!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










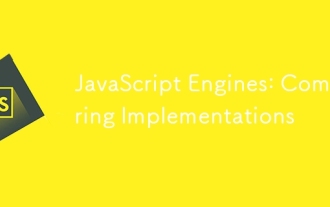
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
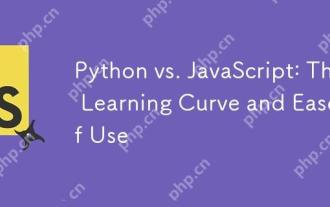
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
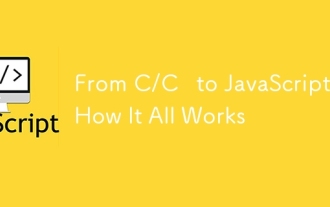
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
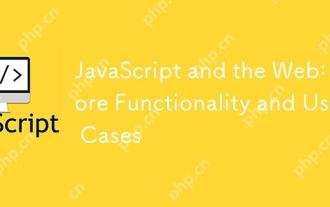
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
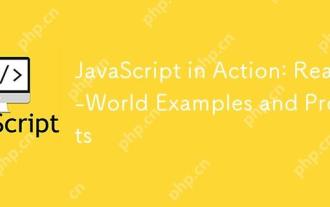
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
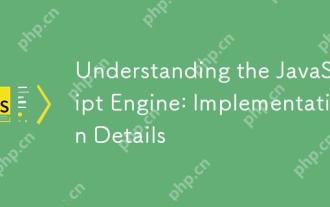
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.
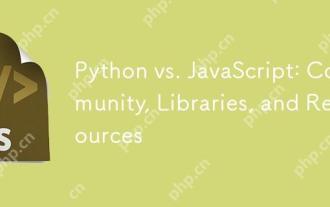
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
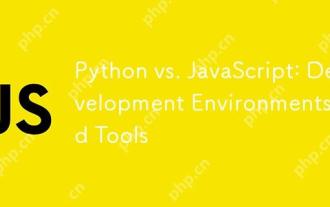
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
